How to Use Ruby Functions for Automated Testing and Continuous Integration
Automated testing and continuous integration (CI) are essential practices in modern software development. They help ensure that your code is robust, reliable, and ready for deployment. Ruby, with its powerful libraries and tools, provides excellent support for these practices. This blog will explore how to use Ruby functions for automated testing and continuous integration, including practical examples and recommended tools.
Automated Testing in Ruby
Automated testing involves running tests to verify that your code behaves as expected. Ruby offers several gems and tools to simplify the process, including RSpec, Minitest, and FactoryBot.
RSpec
RSpec is a widely-used testing framework in Ruby that provides a readable syntax for writing tests. It supports various testing types, including unit tests, integration tests, and behavior-driven development (BDD).
Example: Writing Tests with RSpec
First, add RSpec to your Gemfile:
```ruby Gemfile group :test do gem 'rspec-rails' end ```
Run the following command to install the gem:
```bash bundle install ```
Initialize RSpec in your project:
```bash rails generate rspec:install ```
Create a test for a model:
```ruby spec/models/user_spec.rb require 'rails_helper' RSpec.describe User, type: :model do it 'is valid with valid attributes' do user = User.new(name: 'John Doe', email: 'john@example.com') expect(user).to be_valid end it 'is not valid without an email' do user = User.new(name: 'John Doe') expect(user).to_not be_valid end end ```
Run your tests with:
```bash bundle exec rspec ```
Minitest
Minitest is another popular testing library that comes bundled with Ruby. It provides a fast and straightforward way to write and execute tests.
Example: Writing Tests with Minitest
Create a test file:
```ruby test/models/user_test.rb require 'test_helper' class UserTest < ActiveSupport::TestCase test 'should be valid with valid attributes' do user = User.new(name: 'John Doe', email: 'john@example.com') assert user.valid? end test 'should not be valid without an email' do user = User.new(name: 'John Doe') assert_not user.valid? end end ```
Run your tests with:
```bash rails test ```
Continuous Integration (CI) with Ruby
Continuous integration involves automatically building and testing your codebase every time changes are made. This ensures that any new changes are verified and integrated seamlessly into the project. Popular CI tools like CircleCI, Travis CI, and GitHub Actions can be configured to work with Ruby projects.
- CircleCI
CircleCI is a cloud-based CI tool that integrates easily with GitHub and Bitbucket repositories. It automates the build, test, and deployment process.
Example: Configuring CircleCI
- Create a `.circleci/config.yml` file in your project root:
```yaml version: 2.1 jobs: build: docker: - image: circleci/ruby:2.7 steps: - checkout - run: name: Install dependencies command: bundle install - run: name: Run tests command: bundle exec rspec workflows: version: 2 build_and_test: jobs: - build ```
- Push your changes to trigger CircleCI:
```bash git add .circleci/config.yml git commit -m "Add CircleCI configuration" git push ```
- GitHub Actions
GitHub Actions is a CI/CD tool built into GitHub that allows you to automate workflows directly within your repository.
Example: Configuring GitHub Actions
- Create a `.github/workflows/ci.yml` file:
```yaml name: Ruby CI on: [push, pull_request] jobs: build: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Setup Ruby uses: ruby/setup-ruby@v1 with: ruby-version: 2.7 - name: Install dependencies run: bundle install - name: Run tests run: bundle exec rspec ```
Commit and push your workflow file:
```bash git add .github/workflows/ci.yml git commit -m "Add GitHub Actions configuration" git push ```
Conclusion
Leveraging Ruby functions for automated testing and continuous integration is crucial for maintaining code quality and ensuring smooth deployment processes. By using tools like RSpec, Minitest, CircleCI, and GitHub Actions, you can streamline your development workflow and catch issues early, leading to more reliable and maintainable software.
Further Reading
Table of Contents
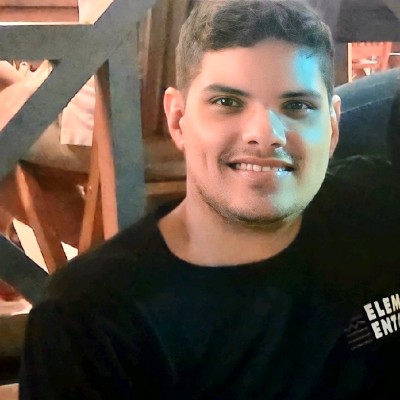
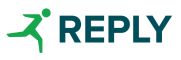