How to Use Ruby Functions for Chatbot Analytics
In the age of digital communication, chatbots have become a crucial tool for businesses to engage with customers, provide support, and streamline processes. However, building a chatbot is just the beginning. To truly harness its potential, it’s essential to analyze interactions and user behavior. This is where chatbot analytics comes into play. By using Ruby functions, you can effectively gather, process, and analyze data from chatbot interactions to gain valuable insights. This blog will explore how to implement chatbot analytics using Ruby, covering data collection, analysis, and visualization.
Setting Up Data Collection
The first step in chatbot analytics is collecting data from user interactions. This data can include user messages, response times, conversation duration, and more. Ruby, with its extensive library ecosystem, provides tools for efficiently logging and storing this data.
Logging User Interactions
One of the simplest ways to collect data is by logging user interactions. You can create a simple logger to store conversations in a database or a file.
Example: Basic Logger
```ruby require 'logger'
- Create a logger instance
logger = Logger.new('chatbot_interactions.log') Log a user interaction def log_interaction(user_id, message, response) logger.info("User ID: {user_id}, Message: {message}, Response: {response}") end
Example usage
log_interaction(123, 'Hello, how can I reset my password?', 'Please visit the account settings page.') ```
This example logs each user interaction, including the user ID, message, and the chatbot’s response. The logs can later be parsed and analyzed.
Storing Data in a Database
For more structured data storage, you can use a database. Ruby’s ActiveRecord gem makes it easy to interact with databases and store structured data.
Example: Storing Interactions with ActiveRecord
- First, set up a database and create a model for interactions.
```ruby Gemfile gem 'activerecord', '~> 6.0' gem 'sqlite3' Run bundle install ```
- Create a migration to define the interactions table:
```ruby db/migrate/20210731000000_create_interactions.rb class CreateInteractions < ActiveRecord::Migration[6.0] def change create_table :interactions do |t| t.integer :user_id t.text :message t.text :response t.timestamps end end end ```
- Define the Interaction model:
```ruby app/models/interaction.rb class Interaction < ActiveRecord::Base end ```
- Save an interaction:
```ruby Interaction.create(user_id: 123, message: 'How do I reset my password?', response: 'Visit account settings.') ```
Analyzing Chatbot Data
Once the data is collected, the next step is analysis. This can involve identifying common user queries, measuring response effectiveness, and tracking user engagement over time.
Common User Queries
To identify common user queries, you can analyze the frequency of certain phrases or keywords in user messages.
Example: Counting Keywords
```ruby def keyword_count Interaction.group(:message).count end puts keyword_count ```
This function counts the number of times each message appears, helping you identify frequently asked questions or common issues.
Response Effectiveness
You can measure response effectiveness by analyzing user feedback or the outcome of interactions.
- Example: Tracking User Feedback
If your chatbot asks for feedback, you can store and analyze it to gauge user satisfaction.
```ruby Assuming feedback is stored in the database def average_feedback_score Interaction.average(:feedback_score) end puts "Average Feedback Score: {average_feedback_score}" ```
Visualizing Data
Data visualization helps in making complex data more understandable. Ruby, combined with tools like Chartkick and Google Charts, can visualize chatbot analytics data.
Using Chartkick for Visualization
Chartkick is a Ruby gem that helps create beautiful JavaScript charts with minimal code.
- Example: Generating a Chart
```ruby Gemfile gem 'chartkick' Run bundle install ```
In your controller:
```ruby app/controllers/analytics_controller.rb class AnalyticsController < ApplicationController def index @interactions = Interaction.group_by_day(:created_at).count end end ```
In your view:
```erb <%= line_chart @interactions %> ```
This example generates a line chart showing the number of interactions over time.
Conclusion
Implementing chatbot analytics using Ruby functions allows you to gather valuable insights from user interactions. By collecting, analyzing, and visualizing data, you can improve your chatbot’s effectiveness, enhance user satisfaction, and optimize engagement strategies. Whether you’re logging interactions, measuring response effectiveness, or visualizing data trends, Ruby provides the tools you need to make informed decisions and refine your chatbot experience.
Further Reading
Table of Contents
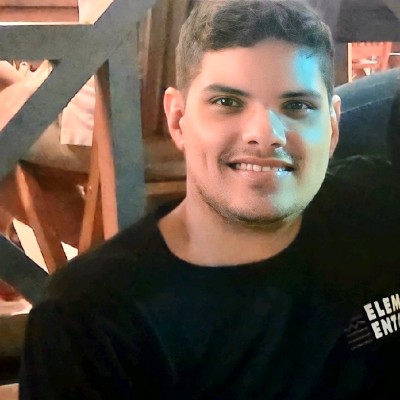
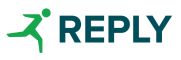