How to Use Ruby Functions for Content Management Systems
Content Management Systems (CMS) are the backbone of many websites, allowing users to create, edit, and manage digital content with ease. Ruby, known for its elegant syntax and powerful libraries, is an excellent choice for developing and managing CMS functionalities. This blog will explore how to use Ruby functions to build and enhance CMS features, providing practical examples to illustrate their applications.
Understanding the Role of Ruby in CMS Development
Ruby’s flexibility and the power of the Ruby on Rails framework make it a popular choice for CMS development. Ruby can handle various CMS functionalities, from content creation and editing to user management and media handling. Let’s dive into how Ruby functions can be used to streamline these tasks.
Implementing Content Creation and Management
Content creation and management are core functionalities of any CMS. Ruby functions can help automate and simplify these processes.
Example: Creating a Simple Content Model
In a Rails-based CMS, you might start by defining a model for your content. Here’s a basic example of a `Post` model that handles content creation:
```ruby app/models/post.rb class Post < ApplicationRecord validates :title, presence: true validates :body, presence: true belongs_to :user Method to publish the post def publish self.update(published: true, published_at: Time.current) end Method to mark the post as draft def draft self.update(published: false, published_at: nil) end end ```
In this example, the `Post` model includes methods for publishing and drafting posts. These methods update the post’s status and timestamp accordingly.
Handling User Management
Managing user roles and permissions is essential for a CMS. Ruby functions can be used to implement user authentication and authorization.
Example: User Authentication with Devise
The Devise gem simplifies user authentication. First, add Devise to your Gemfile and install it:
```ruby Gemfile gem 'devise' ```
Run the installation generator:
```bash rails generate devise:install ```
Generate a User model:
```bash rails generate devise User ```
The `devise` gem provides built-in functions for user authentication, including sign-up, login, and password recovery.
To customize user roles, you might extend the User model:
```ruby app/models/user.rb class User < ApplicationRecord Devise modules devise :database_authenticatable, :registerable, :recoverable, :rememberable, :validatable Roles enum role: { guest: 0, author: 1, admin: 2 } Check if the user is an admin def admin? role == 'admin' end end ```
Managing Media and Attachments
Handling media and attachments is another critical aspect of a CMS. Ruby provides several gems to simplify file uploads and management.
Example: Using Active Storage for File Uploads
Rails’ Active Storage library supports file uploads and attachment management. Add it to your application:
```bash rails active_storage:install rails db:migrate ```
In your model, use `has_one_attached` to associate files:
```ruby app/models/post.rb class Post < ApplicationRecord has_one_attached :image end ```
To upload and display an image in a view:
```erb <!-- app/views/posts/show.html.erb --> <%= image_tag @post.image if @post.image.attached? %> ```
Implementing Search Functionality
Search functionality enhances user experience by allowing users to find content easily. Ruby provides various libraries to implement search features.
Example: Using Ransack for Advanced Search
The Ransack gem provides advanced search capabilities. Add it to your Gemfile:
```ruby Gemfile gem 'ransack' ```
Create a search form in your view:
```erb <!-- app/views/posts/index.html.erb --> <%= search_form_for @q do |f| %> <%= f.label :title_cont, 'Search by Title:' %> <%= f.search_field :title_cont %> <%= f.submit 'Search' %> <% end %> <% @posts.each do |post| %> <%= post.title %> <% end %> ```
In your controller, set up the search query:
```ruby app/controllers/posts_controller.rb def index @q = Post.ransack(params[:q]) @posts = @q.result end ```
Conclusion
Ruby provides a rich set of tools and libraries for building and managing Content Management Systems. From content creation and user management to media handling and search functionality, Ruby functions can streamline these processes and enhance the CMS experience. By leveraging Ruby’s capabilities and integrating popular gems, you can create robust and efficient CMS solutions tailored to your needs.
Further Reading
Table of Contents
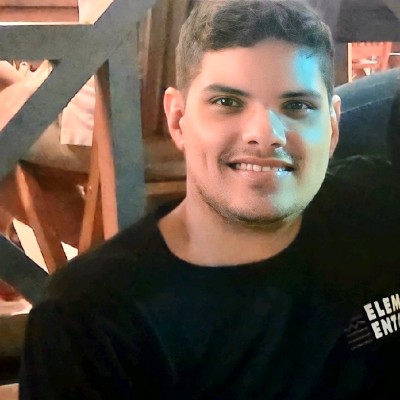
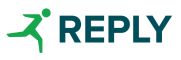