How to Use Ruby Functions for Fraud Detection and Prevention
Fraud detection and prevention are critical aspects of any system handling financial transactions or sensitive data. With the increasing sophistication of fraudulent activities, leveraging programming languages like Ruby can be an effective way to build robust detection systems. This blog will explore how to use Ruby functions for fraud detection and prevention, providing practical examples and tips to get started.
Understanding the Basics of Fraud Detection
Fraud detection involves identifying potentially fraudulent activities by analysing patterns, behaviours, and anomalies. Prevention focuses on implementing measures to minimize the likelihood of such activities occurring. In Ruby, functions can be crafted to perform these tasks efficiently.
Key Ruby Functions for Fraud Detection
- Data Validation Functions
One of the first steps in fraud detection is ensuring the integrity of the data. Ruby offers various functions to validate data, such as checking formats, ranges, and data types.
```ruby def valid_email?(email) !!email.match(/\A[\w+\-.]+@[a-z\d\-.]+\.[a-z]+\z/i) end def valid_transaction_amount?(amount) amount > 0 && amount < 10_000 end ```
These functions validate email formats and ensure transaction amounts fall within expected ranges, helping to filter out erroneous or suspicious entries.
- Pattern Recognition Functions
Pattern recognition is crucial for detecting unusual activities. Ruby’s string and array manipulation capabilities can be used to identify patterns in user behavior.
```ruby def detect_frequent_login_attempts(login_times) threshold = 3 login_times.each_cons(threshold).any? do |attempts| attempts.last - attempts.first < 60 * 5 end end login_times = [1609459200, 1609460000, 1609460400, 1609460450] puts detect_frequent_login_attempts(login_times) Output: true ```
In this example, `detect_frequent_login_attempts` checks for multiple login attempts within a short time frame, flagging them as suspicious.
Implementing Fraud Prevention Measures
- Anomaly Detection Functions
Anomaly detection involves identifying outliers in data. Ruby can be used to implement simple statistical methods for this purpose.
```ruby def detect_anomaly(transactions, threshold = 2) mean = transactions.sum / transactions.size std_dev = Math.sqrt(transactions.map { |t| (t - mean) 2 }.sum / transactions.size) transactions.select { |t| (t - mean).abs > threshold * std_dev } end transactions = [100, 150, 200, 5000, 100] puts detect_anomaly(transactions) Output: [5000] ```
This function calculates the mean and standard deviation of transaction amounts, identifying those that deviate significantly from the norm.
- User Behaviour Monitoring Functions
Monitoring user behaviour over time helps in identifying potential fraudsters. Ruby can track login times, transaction patterns, and more.
```ruby def track_user_behavior(user_actions) suspicious_actions = user_actions.select do |action| action[:type] == 'login' && action[:ip_address] != action[:last_known_ip] end !suspicious_actions.empty? end user_actions = [ { type: 'login', ip_address: '192.168.1.1', last_known_ip: '192.168.1.1' }, { type: 'login', ip_address: '192.168.1.2', last_known_ip: '192.168.1.1' } ] puts track_user_behavior(user_actions) Output: true ```
Here, `track_user_behavior` checks if a user logs in from an unexpected IP address, which could indicate unauthorised access.
Conclusion
Ruby provides a flexible and powerful set of tools for building fraud detection and prevention systems. By using simple functions for data validation, pattern recognition, anomaly detection, and user behavior monitoring, developers can create systems that not only detect fraud but also prevent it proactively. As fraud techniques evolve, continuous monitoring and updating of these functions are crucial.
Further Reading
- [An Introduction to Fraud Detection](https://www.kaggle.com/learn/fraud-detection)
- [Ruby on Rails Security Guide](https://guides.rubyonrails.org/security.html)
- [Machine Learning for Fraud Detection](https://towardsdatascience.com/machine-learning-for-fraud-detection-cc0c96e0e197)
By implementing these strategies, you can better protect your systems and data from fraudulent activities. Start experimenting with these Ruby functions today and stay ahead in the game of fraud prevention!
Table of Contents
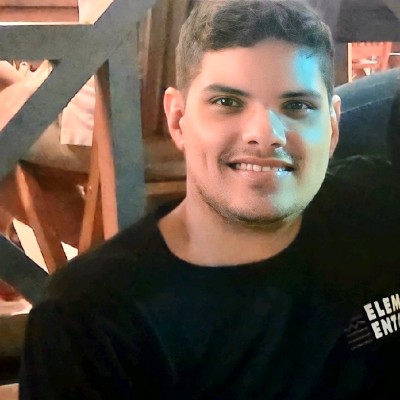
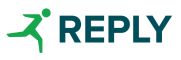