How to Use Ruby Functions for Natural Language Processing
Natural Language Processing (NLP) is an essential field in artificial intelligence, focusing on the interaction between computers and human language. Ruby, known for its simplicity and readability, offers a variety of libraries and functions that can be utilized for NLP tasks. This blog will explore how to use Ruby functions for NLP, providing practical examples and discussing key libraries that make these tasks more accessible.
Introduction to NLP with Ruby
Natural Language Processing involves several tasks, including text parsing, sentiment analysis, entity recognition, and more. While Python is often the go-to language for NLP due to its extensive library support, Ruby also offers powerful tools for these tasks. Libraries like Nokogiri, HTTParty, Treetop, and Ayla provide functionalities that can be leveraged for NLP in Ruby.
Tokenization and Text Preprocessing
Tokenization is a fundamental step in NLP, where text is split into individual tokens (words or phrases). This step is crucial for further analysis, such as sentiment detection or topic modeling.
Example: Tokenizing Text
Ruby’s native string methods and the Nokogiri gem can be used to tokenize text effectively.
```ruby require 'nokogiri' def tokenize(text) doc = Nokogiri::HTML(text) tokens = doc.text.split(/\W+/) tokens.reject(&:empty?) end text = "Natural Language Processing with Ruby is exciting!" tokens = tokenize(text) puts tokens ```
In this example, `Nokogiri` is used to parse HTML content, and the `split` method breaks the text into tokens based on non-word characters.
Sentiment Analysis
Sentiment analysis involves determining the sentiment expressed in a piece of text, such as positive, negative, or neutral. The Sentimental gem in Ruby provides a simple way to perform sentiment analysis.
Example: Sentiment Analysis with Sentimental
First, install the gem:
```bash gem install sentimental ```
Then, use it to analyze sentiment:
```ruby require 'sentimental' Sentimental.load_defaults analyzer = Sentimental.new analyzer.threshold = 0.1 text = "I love using Ruby for NLP!" sentiment = analyzer.sentiment(text) puts "Sentiment: {sentiment}" score = analyzer.score(text) puts "Score: {score}" ```
In this example, `Sentimental` is initialized, and the sentiment of a given text is analyzed. The `score` method returns a numeric score representing the sentiment’s intensity.
Named Entity Recognition (NER)
Named Entity Recognition involves identifying entities like names, dates, and locations within a text. While Ruby doesn’t have as many out-of-the-box NER libraries as Python, it can still be accomplished with regex and external APIs.
Example: Simple NER with Regex
```ruby def extract_entities(text) names = text.scan(/\b[A-Z][a-z]\b/) dates = text.scan(/\b\d{4}-\d{2}-\d{2}\b/) { names: names, dates: dates } end text = "John visited Berlin on 2024-07-31." entities = extract_entities(text) puts "Names: {entities[:names]}" puts "Dates: {entities[:dates]}" ```
In this example, regex patterns are used to extract capitalized words as names and date formats.
Language Detection
Language detection is identifying the language of a given text. The CLD3 gem can be used for this purpose.
Language Detection with CLD3
First, install the gem:
```bash gem install cld3 ```
Then, use it for language detection:
```ruby require 'cld3' text = "Bonjour tout le monde" detector = CLD3::NNetLanguageIdentifier.new language = detector.find_language(text) puts "Detected Language: {language[:language]}" ```
Here, `CLD3` is used to detect the language of a given text string.
Text Summarization
Text summarization involves creating a brief summary of a longer text. While Ruby doesn’t have a dedicated gem for summarization, you can use text rank algorithms or external APIs to achieve this.
Example: Simple Summarization with TF-IDF
```ruby require 'tf-idf-similarity' Example documents documents = [ TfIdfSimilarity::Document.new("Ruby is a great programming language."), TfIdfSimilarity::Document.new("Natural Language Processing is fun."), TfIdfSimilarity::Document.new("I enjoy learning about AI.") ] Create a model model = TfIdfSimilarity::TfIdfModel.new(documents) Rank sentences based on importance ranking = model.term_frequency_in_document(documents[0]) puts ranking ```
In this example, the Tf-Idf-Similarity gem is used to rank sentences by their importance, helping to identify key sentences for summarization.
Conclusion
Ruby offers a variety of tools and libraries for Natural Language Processing, enabling developers to perform tasks like tokenization, sentiment analysis, NER, language detection, and summarization. By leveraging these tools, you can build robust NLP applications in Ruby. While the Ruby NLP ecosystem may not be as extensive as Python’s, it still provides the necessary functionality for many common tasks.
Further Reading
Table of Contents
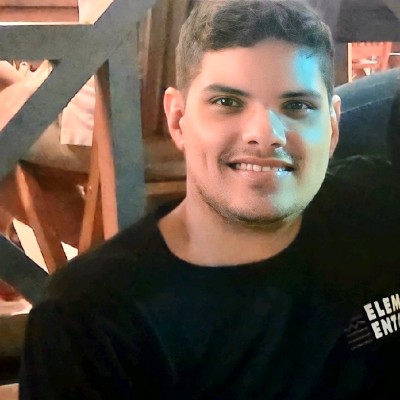
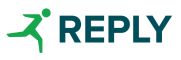