10 Ruby Gems for Blockchain Development
Blockchain technology has revolutionized various industries by providing a decentralized and secure way of storing and transferring data. Ruby, known for its simplicity and elegance, offers a variety of gems that can aid in blockchain development. Whether you’re working on smart contracts, cryptocurrency transactions, or integrating blockchain into your applications, these gems can be invaluable. In this blog, we’ll explore 10 essential Ruby gems for blockchain development.
-
Eth
Eth is a Ruby gem that provides a simple interface for interacting with Ethereum, one of the most popular blockchain platforms. It includes utilities for managing accounts, sending transactions, and working with smart contracts.
Example Usage
Add Eth to your Gemfile:
```ruby gem 'eth' ```
To send a transaction, you can use:
```ruby require 'eth' key = Eth::Key.new(priv: 'your_private_key_here') client = Eth::Client.create('https://mainnet.infura.io/v3/your_infura_project_id') tx = Eth::Tx.new({ data: '0x', gas_limit: 21_000, gas_price: 20_000_000_000, value: 1_000_000_000_000_000_000 }) tx.sign(key) client.eth_send_raw_transaction(tx.hex) ```
-
Bitcoin
Bitcoin is a comprehensive library for the Bitcoin protocol. It provides tools for generating addresses, handling transactions, and interacting with the Bitcoin network.
Example Usage
Add Bitcoin to your Gemfile:
```ruby gem 'bitcoin-ruby' ```
Create a new Bitcoin address:
```ruby require 'bitcoin' key = Bitcoin::Key.generate puts key.addr ```
-
Ethereum
Ethereum is another library for interacting with the Ethereum blockchain. It includes tools for creating and sending transactions, querying blockchain data, and more.
Example Usage
Add Ethereum to your Gemfile:
```ruby gem 'ethereum.rb' ```
Interact with a smart contract:
```ruby require 'ethereum' client = Ethereum::HttpClient.new('http://localhost:8545') abi = 'your_contract_abi_here' address = 'your_contract_address_here' contract = Ethereum::Contract.create(client: client, name: 'ContractName', address: address, abi: abi) puts contract.call.some_method ```
-
Blockchain
Blockchain is a simple wrapper for the Blockchain.info API. It provides functionalities for accessing blockchain data, including wallet operations and transaction history.
Example Usage
Add Blockchain to your Gemfile:
```ruby gem 'blockchain' ```
Fetch Bitcoin address balance:
```ruby require 'blockchain' address = 'your_bitcoin_address_here' balance = Blockchain::Wallet.new.get_address_balance(address) puts balance ```
-
Web3
Web3 is a Ruby implementation of the Ethereum Web3.js library. It allows you to interact with Ethereum nodes using RPC and IPC connections.
Example Usage
Add Web3 to your Gemfile:
```ruby gem 'web3' ```
Connect to an Ethereum node:
```ruby require 'web3' client = Web3::Client.new('http://localhost:8545') puts client.eth_block_number ```
-
Bitcoin::Protocol
Bitcoin::Protocol is a low-level implementation of the Bitcoin protocol. It allows you to work directly with Bitcoin’s data structures and network protocol.
Example Usage
Add Bitcoin::Protocol to your Gemfile:
```ruby gem 'bitcoin-protocol' ```
Parse a Bitcoin block:
```ruby require 'bitcoin/protocol' block_data = 'your_block_data_here' block = Bitcoin::Protocol::Block.new(block_data) puts block.to_json ```
-
Crypto
Crypto is a cryptographic library that supports various encryption algorithms. It is useful for handling cryptographic operations in blockchain applications.
Example Usage
Add Crypto to your Gemfile:
```ruby gem 'crypto' ```
Generate a hash using SHA-256:
```ruby require 'crypto' data = 'your_data_here' hash = Crypto.sha256(data) puts hash ```
-
RubyCoin
RubyCoin is a library for building cryptocurrency applications. It includes tools for generating keys, creating transactions, and more.
Example Usage
Add RubyCoin to your Gemfile:
```ruby gem 'rubycoin' ```
Generate a new private key:
```ruby require 'rubycoin' key = RubyCoin::Key.generate puts key.to_wif ```
-
Eth::Light
Eth::Light is a lightweight library for Ethereum. It is designed for simplicity and ease of use, providing basic functionalities for interacting with the Ethereum network.
Example Usage
Add Eth::Light to your Gemfile:
```ruby gem 'eth-light' ```
Send Ether:
```ruby require 'eth/light' client = Eth::Light::Client.new('http://localhost:8545') client.send_ether(from: 'your_address', to: 'recipient_address', value: 1_000_000_000_000_000) ```
-
RbNaCl
RbNaCl is a Ruby binding for the NaCl (Networking and Cryptography Library) cryptographic library. It provides a simple API for cryptographic operations, essential for secure blockchain applications.
Example Usage
Add RbNaCl to your Gemfile:
```ruby gem 'rbnacl' ```
Encrypt a message:
```ruby require 'rbnacl' key = RbNaCl::Random.random_bytes(RbNaCl::SecretBox.key_bytes) box = RbNaCl::SecretBox.new(key) nonce = RbNaCl::Random.random_bytes(box.nonce_bytes) ciphertext = box.encrypt(nonce, 'your_message_here') puts ciphertext ```
Conclusion
Ruby offers a rich ecosystem of gems for blockchain development, enabling developers to build, interact with, and integrate blockchain technologies into their applications. Whether you’re creating a new cryptocurrency, building a decentralized application (DApp), or working with existing blockchain networks, these gems provide powerful tools to help you succeed.
Further Reading
Table of Contents
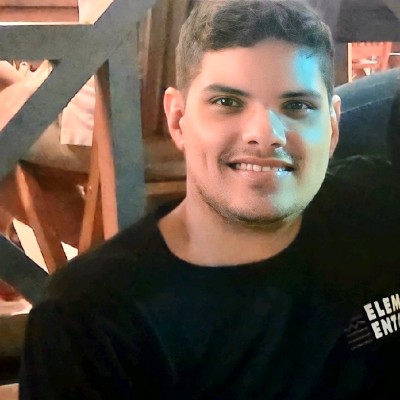
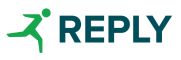