10 Ruby Gems for Social Media Analytics and Monitoring
Social media analytics and monitoring are crucial for understanding engagement, tracking performance, and shaping marketing strategies. Ruby, a versatile and powerful programming language, has a range of gems that can help you analyze and monitor social media platforms efficiently. In this blog, we’ll explore 10 Ruby gems that can aid in social media analytics and monitoring, providing practical examples and use cases for each.
1. Koala
Koala is a Ruby gem for working with the Facebook Graph API. It allows you to access and interact with Facebook’s data, including posts, pages, and user information.
Example Usage
```ruby require 'koala' Initialize the Facebook Graph API client @graph = Koala::Facebook::API.new('your_access_token') Fetch a user's posts user_posts = @graph.get_connections('me', 'posts') puts user_posts ```
Koala makes it easy to integrate Facebook data into your applications and analyze user interactions.
2. twitter
The twitter gem provides a simple way to interact with Twitter’s API. You can use it to fetch tweets, analyze trends, and monitor user activity.
Example Usage
```ruby require 'twitter' Initialize the Twitter client client = Twitter::REST::Client.new do |config| config.consumer_key = 'your_consumer_key' config.consumer_secret = 'your_consumer_secret' config.access_token = 'your_access_token' config.access_token_secret = 'your_access_token_secret' end Fetch recent tweets containing a specific hashtag tweets = client.search('ruby', result_type: 'recent').take(10) tweets.each do |tweet| puts tweet.text end ```
This gem is essential for tracking Twitter mentions, hashtags, and user engagement.
3. linkedin_v2
linkedin_v2 is a Ruby gem for accessing the LinkedIn API, allowing you to gather insights into professional interactions and network activity.
Example Usage
```ruby require 'linkedin_v2' Initialize the LinkedIn client client = LinkedIn::Client.new('your_client_id', 'your_client_secret') Authenticate and fetch profile data client.authorize_from_access('your_access_token') profile = client.profile puts profile ```
Use this gem to track LinkedIn interactions and monitor professional networking metrics.
4. Octokit
Octokit is a Ruby client for the GitHub API. While not specifically for social media, it can be used to monitor GitHub activity and analyze developer engagement.
Example Usage
```ruby require 'octokit' Initialize the GitHub client client = Octokit::Client.new(access_token: 'your_access_token') Fetch recent issues from a repository issues = client.issues('owner/repo') issues.each do |issue| puts issue.title end ```
Octokit is useful for tracking repository activity and developer contributions.
5. Foursquare2
Foursquare2 is a Ruby gem for interacting with the Foursquare API. It helps you gather location-based data and analyze user check-ins and venue information.
Example Usage
```ruby require 'foursquare2' Initialize the Foursquare client client = Foursquare2::Client.new(:oauth_token => 'your_oauth_token') Search for venues nearby venues = client.search_venues(ll: '40.7,-74', query: 'coffee') venues.each do |venue| puts venue.name end ```
Monitor location-based interactions and analyze check-in data with this gem.
6. Pusher
Pusher is a gem for integrating real-time notifications into your application. It’s useful for monitoring and responding to live social media interactions.
Example Usage
```ruby require 'pusher' Initialize the Pusher client Pusher.app_id = 'your_app_id' Pusher.key = 'your_key' Pusher.secret = 'your_secret' Pusher.cluster = 'your_cluster' Pusher.encrypted = true Trigger a real-time event Pusher.trigger('my-channel', 'my-event', { message: 'hello world' }) ```
Use Pusher to create real-time updates and notifications based on social media activity.
7. Google Analytics API
Although not a Ruby gem per se, the google-api-client gem allows you to interact with the Google Analytics API, essential for monitoring social media campaign performance.
Example Usage
```ruby require 'google/apis/analytics_v3' Initialize the Google Analytics client analytics = Google::Apis::AnalyticsV3::AnalyticsService.new analytics.authorization = 'your_access_token' Fetch analytics data response = analytics.get_ga_data('your_profile_id', '30daysAgo', 'today', 'ga:sessions') puts response ```
This gem helps in analyzing web traffic and social media campaign results.
8. Socialite
Socialite is a Ruby gem for managing social media authentication and integrating with multiple social media platforms. It simplifies handling OAuth authentication.
Example Usage
```ruby require 'socialite' Initialize Socialite socialite = Socialite::Client.new(provider: 'twitter', api_key: 'your_api_key', api_secret: 'your_api_secret') Authenticate and fetch user data auth_url = socialite.authenticate_url puts auth_url ```
Manage social media logins and integrations easily with Socialite.
9. Tweepy
Tweepy is a popular Ruby gem for interacting with Twitter’s API, similar to the `twitter` gem but with additional functionalities for deep analytics.
Example Usage
```ruby require 'tweepy' Initialize the Tweepy client client = Tweepy::Client.new(api_key: 'your_api_key', api_secret: 'your_api_secret') Fetch user timeline timeline = client.user_timeline('twitter_username') timeline.each do |tweet| puts tweet.text end ```
Use Tweepy to gather detailed Twitter analytics and insights.
10. Scraper
Scraper is a versatile gem for extracting data from websites, including social media sites where APIs are limited.
Example Usage
```ruby require 'scraper' Initialize Scraper scraper = Scraper.new(url: 'https://twitter.com/twitter_username') Scrape data data = scraper.scrape puts data ```
Use Scraper to gather data from social media platforms that may not have robust APIs.
Conclusion
These 10 Ruby gems provide powerful tools for social media analytics and monitoring, from integrating with APIs to real-time notifications and data scraping. By leveraging these gems, you can gain valuable insights, track performance, and enhance your social media strategy effectively.
Further Reading
Table of Contents
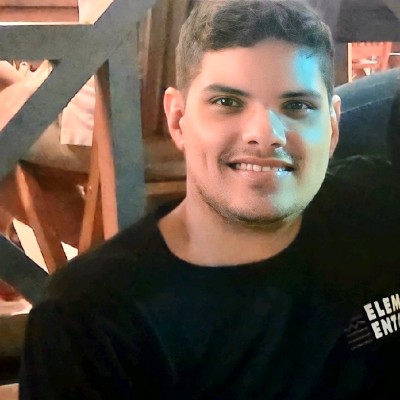
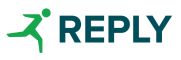