What is the difference between `has_many` and `has_and_belongs_to_many` in Rails?
In Rails, both `has_many` and `has_and_belongs_to_many` are used to define relationships between models, specifically, they represent types of associations. Understanding the distinction between them is crucial for modeling data correctly in a Rails application.
- `has_many` Association: This is a basic one-to-many relationship. When a model `A` `has_many` of another model `B`, it implies that a single instance of model `A` can be associated with multiple instances of model `B`, but each instance of model `B` can only be associated with one instance of model `A`. For example, in a blog application, a `User` model might `has_many` `Posts` since a single user can write multiple posts, but each post is written by one and only one user. This association requires the child model (in this case, `Post`) to have a foreign key column pointing to the parent model (in this case, `User`).
- `has_and_belongs_to_many` Association: This represents a many-to-many relationship between two models. Let’s take an example of `Books` and `Authors`. A book can be written by multiple authors, and an author can write multiple books. Here, neither model is a clear parent or child. To facilitate this relationship in the database, Rails uses a join table, typically named using the plural names of both models in alphabetical order (like `authors_books`). This table will hold foreign keys for both models, creating a matrix of relationships. However, it’s worth noting that if you need to store additional information about the relationship itself, it’s recommended to use a `has_many :through` association instead of `has_and_belongs_to_many`.
The difference lies in the nature of the relationship. `has_many` is for one-to-many relationships where one record in a model is related to multiple records in another. `has_and_belongs_to_many` caters to many-to-many relationships, allowing multiple records in a model to be related to multiple records in another model. When defining relationships in Rails, it’s important to choose the right association to accurately represent the data’s structure and relationships.
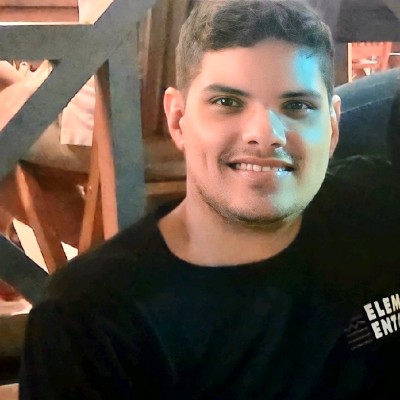
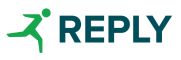