Ruby on Rails Tutorial: Understanding Rails Internationalization and Localization
As the world becomes more connected, creating applications that support multiple languages and regions is increasingly important. Ruby on Rails offers powerful tools for internationalization (I18n) and localization (L10n), making it easier to adapt your application to various languages and cultural contexts. This blog will guide you through the basics of Rails internationalization and localization, explaining how to set up and manage translations, date formats, and more.
Understanding Internationalization (I18n) and Localization (L10n)
Internationalization (I18n) refers to the process of designing a software application so that it can be adapted to various languages and regions without engineering changes. Localization (L10n) is the adaptation of your application to a specific language and region, including translating text and adjusting formats for dates, times, numbers, and currencies.
Setting Up Internationalization in Rails
Rails includes built-in support for I18n. By default, it provides a simple way to manage translations and locale-specific configurations.
Configuring Locales
Locales are defined in YAML files, typically located in the `config/locales` directory. Rails comes with an English locale file by default (`en.yml`). To add a new locale, such as French, you can create a file named `fr.yml`:
```yaml config/locales/fr.yml fr: hello: "Bonjour" welcome: "Bienvenue à notre application" ```
You can specify the default locale for your application in `config/application.rb`:
```ruby config/application.rb module MyApp class Application < Rails::Application config.i18n.default_locale = :en end end
To change the locale dynamically, you can use the `I18n.locale` method:
```ruby I18n.locale = :fr ```
Using Translations in Views
To use translations in your views, you can use the `t` method, which stands for “translate.” For example, to display a translated welcome message:
```erb <!-- app/views/home/index.html.erb --> <h1><%= t('welcome') %></h1> ```
If the current locale is set to French (`:fr`), this will output “Bienvenue à notre application.”
Interpolation in Translations
You can also use interpolation in your translations. This is useful for dynamic content:
```yaml config/locales/en.yml en: hello_name: "Hello, %{name}!" config/locales/fr.yml fr: hello_name: "Bonjour, %{name}!" ```
In your view, you can use:
```erb <p><%= t('hello_name', name: @user.name) %></p> ```
Localizing Dates, Times, and Numbers
Rails allows you to localize dates, times, and numbers according to the current locale. This is done using the `l` method.
Localizing Dates and Times
To display a localized date or time, use the `l` method:
```erb <p><%= l Time.now, format: :short %></p> ```
Define custom date and time formats in your locale files:
```yaml config/locales/en.yml en: time: formats: short: "%b %d, %Y" config/locales/fr.yml fr: time: formats: short: "%d %b %Y" ```
Handling Multiple Locales
To support multiple locales, you’ll need to detect and switch the locale based on user preferences, browser settings, or URLs. A common approach is to set the locale in a `before_action` callback in your `ApplicationController`:
```ruby app/controllers/application_controller.rb class ApplicationController < ActionController::Base before_action :set_locale def set_locale I18n.locale = params[:locale] || I18n.default_locale end def default_url_options { locale: I18n.locale } end end ```
This example sets the locale based on a `locale` parameter in the URL and includes it in all generated URLs.
Best Practices for I18n and L10n
- . Keep Translation Keys Descriptive: Use descriptive keys that make it easy to understand the context, such as `greetings.hello` instead of just `hello`.
- . Avoid Hardcoding Strings: Use the I18n `t` method for all user-visible strings to ensure they can be translated.
- . Use Pluralization: Rails supports pluralization in translations, allowing you to specify different strings for singular and plural forms.
Conclusion
Implementing internationalization and localization in a Ruby on Rails application enables you to reach a broader audience by providing a localized experience. By leveraging Rails’ built-in I18n support, you can easily manage translations and locale-specific formatting. Following best practices and using Rails’ powerful tools, you can create a truly global application.
Further Reading
Table of Contents
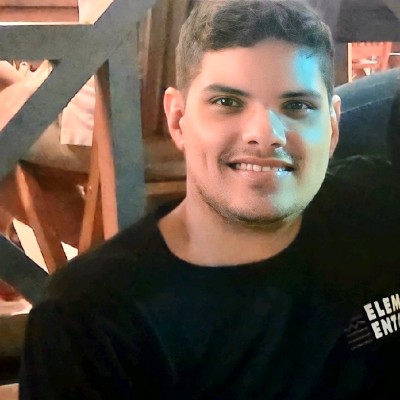
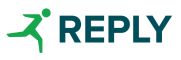