Ruby on Rails Q & A
How to internationalize a Rails app?
Internationalizing a Rails app means preparing it for multiple languages, ensuring users across different regions can engage with it in their preferred language. Here’s how you can internationalize a Rails application:
- Use the I18n Library: Rails comes with a built-in internationalization (I18n) framework that allows you to externalize strings in your application. Initialize it by setting a default locale in `config/application.rb` like so: `config.i18n.default_locale = :en`.
- Store Translations: Create YAML files under `config/locales` to store translations. For example, `en.yml` for English, `es.yml` for Spanish, and so forth. Within these files, you define key-value pairs where the key remains constant, but the value changes based on the language.
- Externalize Strings: Replace hard-coded strings in views, controllers, and models with calls like `I18n.t(‘greeting’)`. Here, `’greeting’` would be a key in your YAML translation file.
- Use Locale with URLs: To provide a localized experience, you can set up your routes to include the locale. For instance, URLs can be structured as `/en/products` or `/es/products`. The locale can then be extracted in your controllers to serve appropriate content.
- Pluralization: The I18n framework supports pluralization. For languages with multiple plural forms, you can specify them in your YAML files and use them in your views.
- Date, Time, and Currency Formatting: Different regions have varying conventions for date, time, and currency. Rails I18n supports these variations, allowing you to present data in a way that feels native to each audience.
- Fallbacks: Sometimes a translation might be missing for a specific language. Configure fallbacks in `config/application.rb` using `config.i18n.fallbacks = [:default_locale]` to ensure the application still provides information in such cases.
- Leverage Gems: There are several gems like `globalize` that extend the I18n functionalities, helping manage model content translations or even automate translations.
Rails offers a comprehensive set of tools for internationalizing an app. When building for a global audience, ensure translations are accurate, culturally appropriate, and continuously updated.
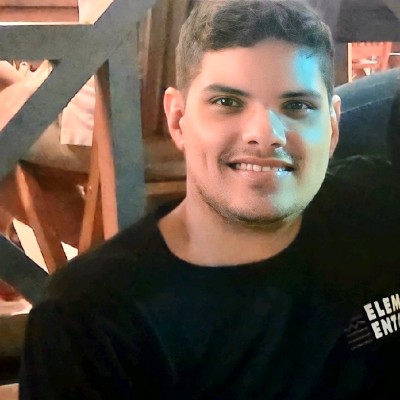
Previously at
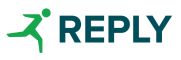
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.