Ruby on Rails Tutorial: Understanding Model Callbacks and Observers
In Ruby on Rails, models are the foundation of the MVC architecture, representing the data and business logic of an application. To manage the flow of data and ensure certain actions occur at specific points in an object’s lifecycle, Rails provides model callbacks and observers. This blog will delve into what these are, how they work, and how you can use them to streamline your application logic.
What Are Model Callbacks?
Model callbacks are methods that get called at various stages of an object’s lifecycle. They allow you to hook into the process of creating, updating, and destroying records, enabling you to execute specific code before or after these events. Rails provides a set of standard callbacks, such as `before_save`, `after_create`, `before_destroy`, and more.
Common Callbacks
Here’s a list of some commonly used callbacks:
– `before_validation`: Runs before validations are checked.
– `after_validation`: Runs after validations are checked.
– `before_save`: Runs before the record is saved.
– `after_save`: Runs after the record is saved.
– `before_create`: Runs before a new record is created.
– `after_create`: Runs after a new record is created.
– `before_update`: Runs before an existing record is updated.
– `after_update`: Runs after an existing record is updated.
– `before_destroy`: Runs before a record is destroyed.
– `after_destroy`: Runs after a record is destroyed.
Implementing Callbacks in Rails
Callbacks are implemented as methods in your model. You can define them directly in the model or as separate private methods and then hook them into the lifecycle events.
Example: Using Callbacks in a Model
```ruby class User < ApplicationRecord before_save :normalize_name after_create :send_welcome_email before_destroy :check_if_admin private def normalize_name self.name = name.downcase.titleize end def send_welcome_email UserMailer.welcome_email(self).deliver_later end def check_if_admin throw :abort if admin? end end ```
In this example, `normalize_name` ensures the user’s name is in a consistent format before saving, `send_welcome_email` sends an email after the user is created, and `check_if_admin` prevents deletion if the user is an admin.
What Are Observers?
Observers are a way to extract callback methods into separate classes, promoting a cleaner and more organised codebase. They are especially useful when you have multiple models that need similar callback logic or when you want to adhere to the Single Responsibility Principle by keeping model classes lean.
Implementing an Observer
To use an observer, you first define an observer class and then link it to the model. Here’s how you can implement an observer in Rails:
```ruby app/models/user_observer.rb class UserObserver < ActiveRecord::Observer def after_create(user) UserMailer.welcome_email(user).deliver_later end def before_destroy(user) throw :abort if user.admin? end end ```
To activate the observer, you need to include it in the application’s configuration:
```ruby config/application.rb config.active_record.observers = :user_observer ```
In this setup, `UserObserver` handles the logic for sending a welcome email and preventing the deletion of admin users, similar to the previous example using callbacks within the model.
Best Practices for Callbacks and Observers
- Keep Callbacks Short: Callbacks should be concise and focused on specific tasks. Complex logic should be extracted into service objects or other classes.
- Avoid Business Logic: Avoid placing business logic in callbacks; instead, use them for housekeeping tasks like formatting data or triggering notifications.
- Use Observers for Reusability: When multiple models share similar callback logic, consider using observers to promote code reusability and separation of concerns.
Conclusion
Model callbacks and observers are powerful features in Ruby on Rails that help manage the lifecycle of your objects. While callbacks allow for inline method execution, observers offer a cleaner, more modular approach by separating concerns. Understanding when and how to use these tools can significantly enhance the maintainability and organization of your Rails application.
Further Reading
- [Rails Guides: Active Record Callbacks](https://guides.rubyonrails.org/active_record_callbacks.html)
- [Understanding Rails Observers](https://api.rubyonrails.org/classes/ActiveRecord/Observer.html)
- [Rails Best Practices](https://rails-bestpractices.com/)
By mastering callbacks and observers, you can streamline your Rails development process, ensuring your application behaves as expected at every stage of an object’s lifecycle. Happy coding!
Table of Contents
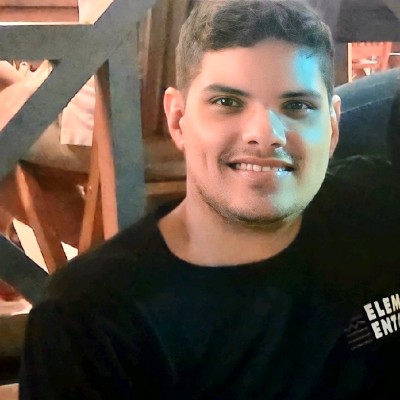
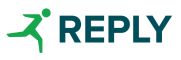