Ruby on Rails Tutorial: Understanding Model Transactions and Locking
In Ruby on Rails, managing data integrity and handling concurrent database access are crucial for building reliable and scalable applications. Two key mechanisms for achieving this are model transactions and locking. This blog will delve into these concepts, explaining how to use them effectively to maintain data consistency and handle concurrent operations.
What Are Model Transactions?
Model transactions in Rails allow you to group multiple database operations into a single unit of work. If any operation within the transaction fails, the entire transaction is rolled back, ensuring that your database remains in a consistent state.
Basic Usage of Transactions
Rails transactions are typically used to wrap multiple model operations. Here’s a basic example:
```ruby app/controllers/posts_controller.rb class PostsController < ApplicationController def create ActiveRecord::Base.transaction do @post = Post.create!(post_params) @comment = Comment.create!(comment_params.merge(post_id: @post.id)) end rescue ActiveRecord::RecordInvalid => e flash[:error] = "Error creating post and comment: {e.message}" render :new end private def post_params params.require(:post).permit(:title, :body) end def comment_params params.require(:comment).permit(:content) end end ```
In this example, both `Post` and `Comment` records are created within a transaction. If either creation fails, the entire transaction is rolled back, and no records are saved.
Understanding Locking Mechanisms
Locking mechanisms are used to handle concurrent access to records in the database. When multiple users or processes attempt to modify the same record simultaneously, locking ensures that these operations do not interfere with each other, thus maintaining data consistency.
Pessimistic Locking
Pessimistic locking involves locking a record for update when it is read, preventing other transactions from modifying it until the lock is released.
```ruby app/controllers/orders_controller.rb class OrdersController < ApplicationController def update Order.transaction do @order = Order.lock('FOR UPDATE').find(params[:id]) @order.update!(order_params) end rescue ActiveRecord::StaleObjectError flash[:error] = "The order was updated by someone else." redirect_to @order end private def order_params params.require(:order).permit(:status) end end ```
In this example, the `lock(‘FOR UPDATE’)` method is used to lock the record for update. If another process attempts to update the same record, it will be blocked until the current transaction is complete.
Optimistic Locking
Optimistic locking relies on a version column in the database to manage concurrent updates. When a record is updated, Rails checks if the version number has changed since it was last read. If it has, the update is rejected.
To use optimistic locking, add a `lock_version` column to your model:
```ruby db/migrate/xxxxxx_add_lock_version_to_orders.rb class AddLockVersionToOrders < ActiveRecord::Migration[6.1] def change add_column :orders, :lock_version, :integer, default: 0, null: false end end ```
Update your model:
```ruby app/models/order.rb class Order < ApplicationRecord No additional code needed for optimistic locking end ```
In this case, Rails automatically handles version checks. If a concurrent update occurs, an `ActiveRecord::StaleObjectError` will be raised.
Best Practices for Transactions and Locking
- Keep Transactions Short: Ensure transactions are short and focused to minimize lock contention and improve performance.
- Use Appropriate Locking: Choose the right locking strategy based on your application’s needs. Pessimistic locking is suitable for scenarios with high contention, while optimistic locking works well when conflicts are rare.
- Handle Exceptions Gracefully: Ensure that your application handles transaction rollbacks and locking exceptions gracefully to provide a good user experience.
- Test Concurrent Scenarios: Test your application’s behavior under concurrent access conditions to ensure data integrity and correct handling of locking scenarios.
Conclusion
Understanding and implementing model transactions and locking mechanisms in Ruby on Rails are essential for maintaining data integrity and handling concurrent database access. By using transactions to group database operations and choosing the appropriate locking strategy, you can build robust and reliable Rails applications that perform well under concurrent loads.
Further Reading
Table of Contents
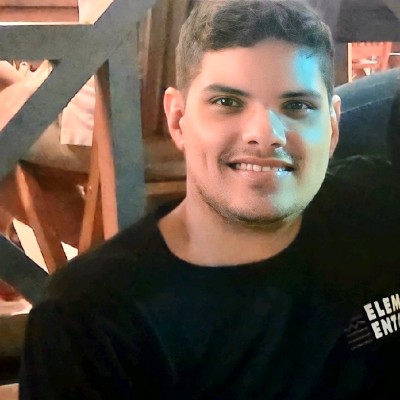
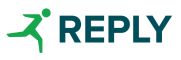