10 Ruby Gems for Mobile App Development
Ruby is widely known for its elegant syntax and powerful web development capabilities with Rails, but it can also play a significant role in mobile app development. Whether you’re building a mobile backend or working with cross-platform frameworks, several Ruby gems can streamline your development process. This blog will explore ten essential Ruby gems for mobile app development, covering various aspects like testing, networking, and UI.
1. Fastlane
Fastlane is a powerful toolset for automating tedious tasks in mobile app development, such as screenshots, beta deployments, and releases.
Example Usage
Add Fastlane to your project by installing it:
```bash gem install fastlane ```
Initialize Fastlane in your project:
```bash fastlane init ```
Use Fastlane to automate app builds and deployments with simple commands:
```bash fastlane ios beta ```
Fastlane integrates with both iOS and Android, streamlining the deployment process.
2. Cocoapods
Cocoapods is the dependency manager for Swift and Objective-C Cocoa projects. It simplifies managing third-party libraries and frameworks in your iOS projects.
Example Usage
Install Cocoapods:
```bash gem install cocoapods ```
Initialize Cocoapods in your project directory:
```bash pod init ```
Add dependencies to your `Podfile`:
```ruby Podfile platform :ios, '10.0' target 'YourApp' do use_frameworks! pod 'Alamofire', '~> 5.4' end ```
Install the dependencies:
```bash pod install ```
3. RestClient
RestClient is a simple HTTP and REST client that helps you make network requests effortlessly. It’s particularly useful for interacting with APIs in mobile app backends.
Example Usage
Install RestClient:
```bash gem install rest-client ```
Use RestClient to make a GET request:
```ruby require 'rest-client' response = RestClient.get 'https://api.example.com/data' puts response.body ```
4. HTTParty
HTTParty is another popular gem for making HTTP requests. It’s known for its simplicity and flexibility.
Example Usage
Add HTTParty to your Gemfile:
```ruby gem 'httparty' ```
Fetch and parse JSON data:
```ruby require 'httparty' response = HTTParty.get('https://api.example.com/data') data = response.parsed_response puts data ```
5. Capybara
Capybara is a Ruby library used for testing web applications. It simulates how a user would interact with your app, making it ideal for integration testing.
Example Usage
Add Capybara to your Gemfile:
```ruby gem 'capybara' ```
Write a simple feature test:
```ruby require 'capybara/rspec' Capybara.current_driver = :selenium feature 'User visits homepage' do scenario 'successfully' do visit '/' expect(page).to have_content('Welcome to My App') end end ```
6. Puma
Puma is a concurrent web server for Ruby/Rack applications. It’s optimized for performance and can handle multiple requests simultaneously, making it suitable for mobile app backends.
Example Usage
Add Puma to your Gemfile:
```ruby gem 'puma' ```
Configure Puma by editing the `config/puma.rb` file. Example configuration:
```ruby workers 2 threads 1, 6 port 3000 ```
Start Puma server:
```bash bundle exec puma ```
7. Sidekiq
Sidekiq is a background job processor that handles asynchronous tasks and improves app performance by offloading work to background workers.
Example Usage
Add Sidekiq to your Gemfile:
```ruby gem 'sidekiq' ```
Create a job:
```ruby class HardWorker include Sidekiq::Worker def perform(name, count) puts "Doing hard work" end end ```
Configure Sidekiq with Redis and start processing jobs:
```bash bundle exec sidekiq ```
8. SimpleCov
SimpleCov is a code coverage analysis tool for Ruby. It helps ensure your code is well-tested by providing detailed coverage reports.
Example Usage
Add SimpleCov to your Gemfile for the test group:
```ruby group :test do gem 'simplecov' end ```
Configure SimpleCov in your test setup file:
```ruby require 'simplecov' SimpleCov.start ```
9. Faker
Faker is a library for generating fake data, which is useful for creating realistic test data for your mobile app.
Example Usage
Add Faker to your Gemfile:
```ruby gem 'faker' ```
Generate fake data:
```ruby require 'faker' puts Faker::Name.name puts Faker::Address.city ```
10. FactoryBot
FactoryBot is a library for setting up Ruby objects as test data. It’s especially useful for creating consistent test data for your mobile app’s backend.
Example Usage
Add FactoryBot to your Gemfile:
```ruby gem 'factory_bot_rails' ```
Define a factory:
```ruby spec/factories/users.rb FactoryBot.define do factory :user do name { "John Doe" } email { "john@example.com" } end end ```
Create a user in your tests:
```ruby user = FactoryBot.create(:user) ```
Conclusion
These ten Ruby gems provide valuable tools and functionalities for mobile app development, from managing dependencies and making network requests to testing and background processing. By integrating these gems into your development workflow, you can enhance your productivity and streamline the development process for mobile applications.
Further Reading
Table of Contents
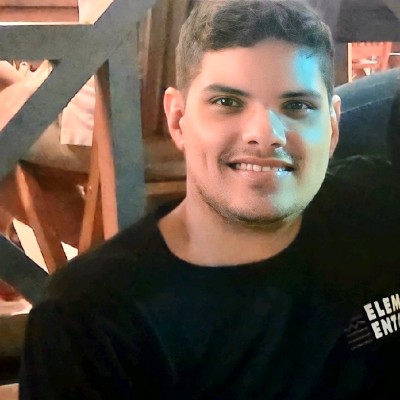
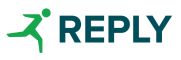