Ruby on Rails Tutorial: Understanding Rails Plugins and Extensions
Ruby on Rails, a powerful web application framework, provides a rich ecosystem of tools to enhance and extend its functionality. Two key components of this ecosystem are plugins and extensions, which allow developers to add features and customize their applications without modifying the core framework. This blog will explore the concept of Rails plugins and extensions, how they differ, and provide practical examples to illustrate their usage.
What Are Rails Plugins and Extensions?
Plugins and extensions in Rails are mechanisms to modularize and reuse code across multiple applications. While often used interchangeably, they serve slightly different purposes:
– Plugins are essentially packages of code that can be dropped into a Rails application to extend its functionality. They are self-contained and can include models, controllers, views, and other components.
– Extensions are modifications or additions to the Rails framework itself, often enhancing or altering the behavior of existing components.
Both plugins and extensions are valuable for developers looking to add features or modify behavior without directly altering the Rails codebase.
Creating a Rails Plugin
Creating a Rails plugin is straightforward, thanks to the built-in generator. Let’s walk through creating a simple plugin.
Step 1: Generate the Plugin
You can generate a new plugin using the Rails command-line tool. For example, to create a plugin named `MyPlugin`:
```bash rails plugin new MyPlugin --full ```
The `–full` option generates a complete structure for the plugin, including a directory for models, controllers, views, and more.
Step 2: Define the Plugin Functionality
Inside your newly created plugin, you can define any functionality you need. For example, let’s add a simple model:
```ruby my_plugin/app/models/my_plugin/simple_model.rb module MyPlugin class SimpleModel < ApplicationRecord validates :name, presence: true end end ```
This model can then be used within the main Rails application, just like any other model.
Step 3: Using the Plugin
To use the plugin in a Rails application, you typically include it in the `Gemfile`:
```ruby Gemfile gem 'my_plugin', path: 'path/to/my_plugin' ```
Then, run `bundle install` to install the plugin. You can now use `SimpleModel` in your application.
Understanding Rails Extensions
Rails extensions are typically implemented as gems that add or modify functionality within the Rails framework. They can range from simple patches to extensive features. For instance, the Devise gem is a popular extension for user authentication.
Example: Creating a Simple Extension
Let’s create a simple extension to add a helper method to all controllers in a Rails application.
- Create the Gem Structure:
Use `bundler` to create a new gem:
```bash bundle gem my_extension ```
2. Define the Extension:
In the generated gem, define a module that will be included in controllers:
```ruby my_extension/lib/my_extension/controller_helpers.rb module MyExtension module ControllerHelpers def say_hello puts 'Hello from MyExtension!' end end end ```
3. Include the Module in Rails Controllers:
In the gem’s main file, include the module in Rails controllers:
```ruby my_extension/lib/my_extension.rb require 'my_extension/controller_helpers' module MyExtension class Railtie < Rails::Railtie initializer 'my_extension.controller_helpers' do ActiveSupport.on_load(:action_controller) do include MyExtension::ControllerHelpers end end end end ```
- Using the Extension:
Include the gem in your Rails application’s `Gemfile` and call `say_hello` from any controller.
Best Practices for Using Plugins and Extensions
- Modularity and Reusability: Ensure that plugins and extensions are modular and reusable. They should solve specific problems without being tightly coupled to the application.
- Avoid Overloading: While plugins and extensions are useful, avoid overloading your application with too many. This can lead to maintenance challenges and compatibility issues.
- Documentation and Testing: Properly document and test your plugins and extensions. This ensures that other developers can understand and use them effectively.
Conclusion
Rails plugins and extensions provide a powerful way to extend the functionality of your Rails applications. Whether you’re adding new features or customizing existing behavior, these tools enable you to modularize and reuse code effectively. By understanding how to create and implement plugins and extensions, you can enhance your Rails applications and streamline your development process.
Further Reading
Table of Contents
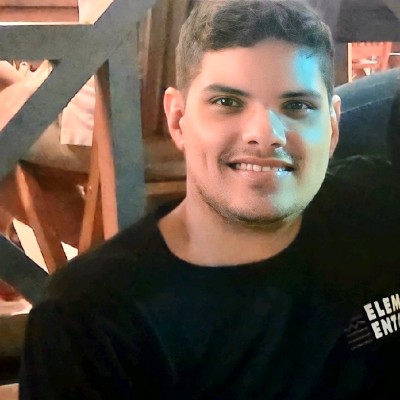
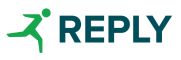