Building Web Applications with Ruby: Leveraging Its Frameworks
Web development is a continually evolving landscape with a range of languages and frameworks at a developer’s disposal. Among the myriad of choices, Ruby shines with its syntax that emphasizes simplicity and productivity. Furthermore, when you hire Ruby developers, you gain access to their expertise in utilizing Ruby’s powerful frameworks like Rails and Sinatra. These frameworks empower developers to construct robust and scalable web applications with efficiency.
This article delves into how to leverage these frameworks effectively, providing insights that can be greatly beneficial when you decide to hire Ruby developers to enhance your web application development efforts.
A Brief Overview of Ruby
Ruby, a high-level, interpreted language, was designed to facilitate programming work and make it more fun. Its creator, Yukihiro “Matz” Matsumoto, incorporated elements from his favorite languages – Perl, Smalltalk, Eiffel, Ada, and Lisp to create Ruby, ensuring it was natural to write and easy to read.
One of the significant advantages of Ruby is its principle of least astonishment (POLA). This principle means that Ruby behaves in a way that minimizes confusion for experienced users, thereby reducing the potential for unexpected bugs and system crashes. Furthermore, Ruby employs garbage collection and dynamic typing, facilitating easy and quick coding.
Ruby on Rails
Ruby on Rails, often simply referred to as Rails, is a server-side web application framework written in Ruby. Rails adopts the MVC (Model-View-Controller) architecture, providing default structures for databases, web pages, and web services.
Rails is designed with the philosophy of “convention over configuration,” which means that the programmer doesn’t have to spend a lot of time configuring files to get set up. This feature helps speed up the development process significantly. In addition, Rails also encourages DRY (Don’t Repeat Yourself) and RESTful application design, making your application more maintainable and efficient.
To give you a glimpse of how straightforward it is to set up a new Rails application, here’s a simple procedure. All you need is Ruby and Rails installed on your system:
```ruby # Create a new Rails application rails new myapp # Move into the new application's directory cd myapp # Start the server rails server
Your application will be running on `http://localhost:3000`, and you can begin building immediately.
Sinatra
While Rails is excellent for larger, complex applications, it might be overkill for smaller, simpler ones. That’s where Sinatra comes in. Sinatra is a DSL (Domain Specific Language) for quickly creating web applications in Ruby with minimal effort.
Unlike Rails, Sinatra doesn’t follow the MVC pattern or any specific project structure – it’s simple and flexible. In fact, you can create a basic web application with just a single file.
Here’s a simple Sinatra application:
```ruby require 'sinatra' get '/' do 'Hello, world!' end
This application responds with ‘Hello, world!’ for requests to the root URL or route.
Choosing the Right Framework
The choice between Sinatra and Ruby on Rails depends on the specific requirements of your web application. Let’s illustrate this with examples.
Example 1: Building a Blog
Let’s say you’re building a blog application that requires features like user authentication, commenting, archiving posts, and more. These features necessitate interaction with a database, and Rails, with its built-in ActiveRecord ORM (Object-Relational Mapping), makes it easy to interact with databases. It also provides scaffolding, which makes it easy to create the initial version of the web application.
Moreover, Rails follows the MVC pattern which will keep your code organized and maintainable as your application grows. Additionally, using gems like Devise for authentication, you can easily add complex features with minimal coding. Therefore, for a feature-rich blog application, Rails would be the right choice.
Example 2: Building a Microservice
Now, let’s assume you’re building a simple microservice for a weather app that fetches the current weather data from an API and displays it. It’s a lightweight, single-purpose application with one or two routes. In this case, the full functionality and structure of Rails might be more than what you need.
Sinatra, with its simplicity and flexibility, shines in these scenarios. You could write a Sinatra application to do this in just a few lines of code:
```ruby require 'sinatra' require 'net/http' require 'json' get '/weather/:location' do uri = URI("https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q=#{params[:location]}") res = Net::HTTP.get_response(uri) weather_data = JSON.parse(res.body) "The current temperature in #{params[:location]} is #{weather_data['current']['temp_c']} degrees Celsius." end
This code creates a simple web server that fetches weather data based on a location parameter and returns it. For simple, specific functionality like this, Sinatra would be an ideal choice.
Example 3: Building an E-commerce Website
For an e-commerce website, you’d need multiple functionalities like user authentication, product management, shopping cart, payment gateway integration, and much more. This scenario calls for a robust framework that can handle complexity with ease – Rails.
Rails’ MVC architecture and built-in features like ActiveRecord, ActionMailer, ActiveSupport, and the availability of numerous gems, make it easier to build, test, and maintain large-scale applications. For instance, you could use gems like Spree or Solidus to add e-commerce functionality to your Rails application quickly.
Choosing the right Ruby framework depends largely on the size, complexity, and specific requirements of your project. While Rails is built for creating complex, large-scale applications, Sinatra excels at building simple, lightweight, and specific-use applications. Understanding the strengths and ideal use cases for each can help you make the right decision for your web application project.
Ruby’s Ecosystem
Ruby’s ecosystem is rich, providing a vast array of libraries (gems) that can assist you in your web development journey. These gems cater to a wide range of functionalities, from authentication (Devise) to file uploads (CarrierWave), background jobs (Sidekiq), and even APIs (Grape). This diverse landscape of resources simplifies the development process significantly, and when you hire Ruby developers, you leverage their expertise in navigating and utilizing these resources effectively. With their help, you’re likely to find a gem that precisely fits your application’s needs.
Conclusion
Ruby, with its intuitive syntax and focus on developer happiness, combined with the power and flexibility of Rails and Sinatra, makes it a solid choice for web application development. Whether you’re building a complex, feature-rich application or a simple web service, Ruby and its frameworks have got you covered. If you’re looking to rapidly scale your web application, you might consider the option to hire Ruby developers.
Remember, the beauty of software development lies in the ability to choose the best tools for your specific needs. By understanding the capabilities of both Rails and Sinatra, you can ensure that you’re leveraging Ruby to its full potential. Hiring skilled Ruby developers can further augment this potential, bringing extensive experience to your project.
In the end, Ruby is much more than a programming language – it’s a community. The Ruby community, full of professional Ruby developers available for hire, is one of the most welcoming. It provides plenty of resources to help you learn and grow as a developer. So dive in, start building, and experience the joy of programming with Ruby!
Table of Contents
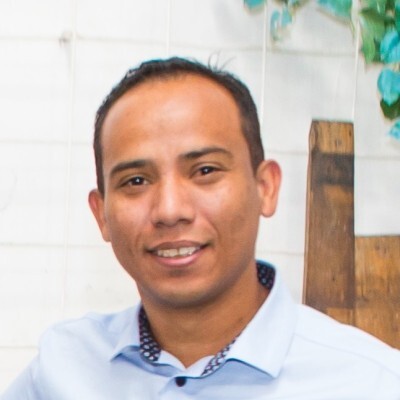
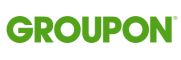