How to concatenate strings in Ruby?
In Ruby, you can concatenate strings using several methods and operators to combine multiple string values into a single string. Here are some common approaches to concatenate strings in Ruby:
- String Interpolation: One of the most straightforward and commonly used methods for concatenating strings in Ruby is string interpolation. You can embed expressions or variables within a string using `#{}` inside double-quoted strings. Ruby evaluates the expression or variable and replaces it with its value within the string.
```ruby first_name = "John" last_name = "Doe" full_name = "#{first_name} #{last_name}" ```
In this example, `full_name` will contain the concatenated full name “John Doe.”
- `+` Operator: You can use the `+` operator to concatenate two or more strings together. This operator combines the contents of the strings on either side of it.
```ruby greeting = "Hello, " name = "Alice" message = greeting + name ```
The `message` variable will contain the concatenated string “Hello, Alice.”
- `<<` Operator: The `<<` operator is used to append one string to another. It modifies the original string to include the additional content.
```ruby text = "Hello, " text << "world!" ```
After this code, the `text` variable will hold the string “Hello, world!”
- `.concat` Method: Ruby provides the `.concat` method that works similarly to the `<<` operator, allowing you to append one string to another.
```ruby sentence = "Ruby is " sentence.concat("awesome!") ```
The `sentence` variable will now contain “Ruby is awesome!”
- `.join` Method (for Arrays): If you have an array of strings, you can use the `.join` method to concatenate them with a delimiter string in between.
```ruby words = ["Ruby", "is", "fun"] sentence = words.join(" ") ```
The `sentence` variable will hold the string “Ruby is fun.”
These methods and operators provide flexibility for concatenating strings in Ruby, allowing you to choose the approach that best suits your coding style and specific use case.
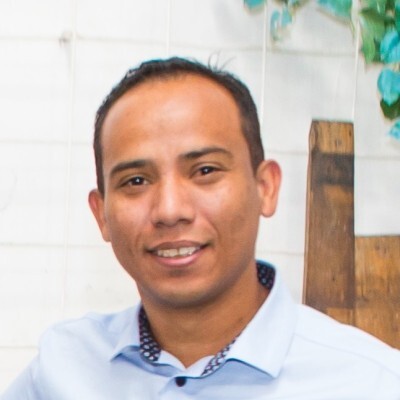
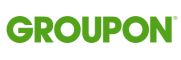