Exploring Ruby’s File Manipulation Functions: Reading, Writing, and Beyond
Ruby, a versatile and elegant programming language, provides developers with an array of powerful features for file manipulation. Whether you’re reading data from files, writing data to them, or performing more complex file operations, Ruby has got you covered. In this blog, we’ll dive deep into Ruby’s file manipulation functions, explore various techniques, and provide code samples to help you understand and leverage these capabilities effectively.
Table of Contents
1. Introduction to File Manipulation in Ruby
File manipulation is a fundamental aspect of programming, and Ruby makes it a breeze with its built-in methods and classes. Before diving into the specifics, let’s understand the basic flow of file manipulation in Ruby:
- Opening: Before reading from or writing to a file, we need to open it. Ruby provides various modes for opening files, depending on the type of operation we want to perform.
- Processing: Once the file is open, we can read its contents, write data to it, or perform other operations.
- Closing: After we’re done with the file, it’s essential to close it to release system resources.
Throughout this blog, we’ll explore each step in detail, along with practical code examples.
2. Reading Data from Files
2.1. Reading a Entire File
To read the entire contents of a file, we can use the File.read method. It simplifies reading small files in one go:
ruby file_contents = File.read('sample.txt') puts file_contents
2.2. Reading Line by Line
For larger files, reading them entirely into memory might not be feasible. Ruby provides a more memory-efficient way to read files line by line using File.foreach:
ruby File.foreach('large_file.log') do |line| puts line end
2.3. Reading with IO Objects
Ruby’s File class inherits from the IO class, which means we can also use IO methods to read files. The advantage here is the ability to control the read buffer size for performance optimization:
ruby file = File.open('data.csv', 'r') while (line = file.read(4096)) puts line end file.close
3. Writing Data to Files
3.1. Writing to a File
To write data to a file, we can use the File.write method. This method creates a new file if it doesn’t exist or overwrites the existing content:
ruby data = "Hello, this is some content to be written to the file!" File.write('output.txt', data)
3.2. Appending to a File
If we want to add content to an existing file without overwriting it, we can use the File.open method with the append mode ‘a’:
ruby File.open('log.txt', 'a') do |file| file.puts "This line will be appended to the end of the file." end
3.3. Writing with IO Objects
Similar to reading, we can also write to a file using IO objects:
ruby file = File.open('data.csv', 'w') file.puts "Name, Age, Occupation" file.puts "John Doe, 30, Engineer" file.puts "Jane Smith, 25, Designer" file.close
4. File Pointers and Seek
In Ruby, file objects maintain an internal pointer called the “file pointer” that keeps track of the current position in the file. When we read from or write to a file, the file pointer moves accordingly. We can also manually control the file pointer using the seek method:
ruby file = File.open('large_file.log', 'r') file.seek(100, IO::SEEK_SET) # Move 100 bytes from the start of the file puts file.read(50) # Read and print the next 50 bytes from the new position file.close
5. File Handling Best Practices
Working with files requires attention to detail to ensure smooth operations and prevent potential issues. Here are some best practices:
5.1. Always Close Files
Failing to close files after processing can lead to resource leaks. Use the File.open block syntax to ensure the file is automatically closed after use:
ruby File.open('data.txt', 'r') do |file| # File processing code here end
5.2. Use File Methods with Blocks
Whenever possible, use methods that accept blocks (e.g., File.foreach, File.open) to avoid manually managing file closing and error handling.
5.3. Error Handling
Wrap file operations in a begin…rescue…ensure block to handle any potential exceptions:
ruby begin File.open('missing_file.txt', 'r') do |file| # File processing code here end rescue Errno::ENOENT => e puts "File not found: #{e.message}" ensure file&.close end
6. Advanced File Operations
6.1. Renaming and Deleting Files
Ruby allows us to rename and delete files using File.rename and File.delete methods, respectively:
ruby File.rename('old_name.txt', 'new_name.txt') File.delete('file_to_be_deleted.txt')
6.2. Checking File Existence
To check if a file exists before performing any operations on it, we can use the File.exist? method:
ruby file_name = 'data.csv' if File.exist?(file_name) puts "#{file_name} exists!" else puts "#{file_name} does not exist!" end
6.3. Getting File Information
Ruby provides several methods to retrieve file information:
ruby file_name = 'data.csv' puts "File size: #{File.size(file_name)} bytes" puts "File type: #{File.ftype(file_name)}" puts "File directory: #{File.dirname(file_name)}" puts "File extension: #{File.extname(file_name)}" puts "File created at: #{File.ctime(file_name)}" puts "File modified at: #{File.mtime(file_name)}"
6.4. Directory Operations
Ruby also allows us to work with directories using Dir class methods:
ruby Dir.mkdir('new_directory') Dir.chdir('new_directory') puts Dir.pwd # Perform operations in the new directory Dir.chdir('..') # Move back to the original directory Dir.rmdir('new_directory') # Remove the empty directory
7. Working with CSV Files
Handling CSV (Comma-Separated Values) files is a common task in data processing. Ruby’s CSV library simplifies working with CSV files:
ruby require 'csv' # Reading CSV CSV.foreach('data.csv') do |row| puts row.join(', ') end # Writing CSV data = [ ['Name', 'Age', 'Occupation'], ['John Doe', 30, 'Engineer'], ['Jane Smith', 25, 'Designer'] ] CSV.open('output.csv', 'w') do |csv| data.each { |row| csv << row } end
8. Binary File Handling
In some cases, we might need to work with binary files, such as images or audio files. Ruby can handle binary data effectively:
ruby # Reading binary file data = File.binread('image.png') # Writing binary file File.binwrite('copy_image.png', data)
9. Error Handling and File Operations
When dealing with files, there is always a chance of encountering errors, such as file not found or permissions issues. Ensure proper error handling to make your code robust and user-friendly.
Conclusion
In this blog, we’ve explored Ruby’s file manipulation functions, covering reading, writing, and advanced file operations. Armed with this knowledge, you can confidently work with files, process data, and build powerful applications in Ruby. Remember to follow best practices, handle errors gracefully, and explore the vast range of possibilities that file manipulation in Ruby has to offer! Happy coding!
Table of Contents
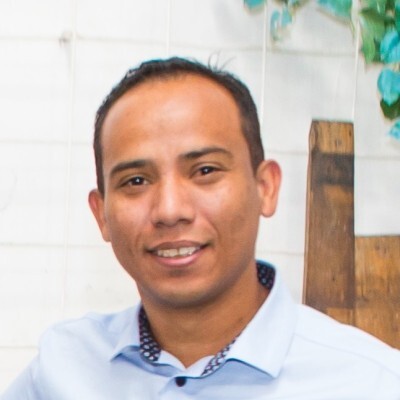
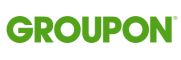