Ruby for Network Automation: Simplifying Infrastructure Management
In the fast-paced world of network and infrastructure management, automation has become a cornerstone for efficiency, scalability, and reliability. Among the array of programming languages available for this purpose, Ruby stands out as a versatile and powerful option. Its elegant syntax, extensive libraries, and community support make it an ideal choice for simplifying complex infrastructure management tasks. In this blog post, we will delve into how Ruby can be utilized for network automation, exploring its benefits and providing practical insights through code samples.
Table of Contents
1. Why Ruby for Network Automation?
1.1. Clean and Intuitive Syntax
One of the key reasons why Ruby is favored for network automation is its clean and intuitive syntax. The language’s syntax is designed to be easily readable, resembling natural language to a significant extent. This aspect makes it approachable for both experienced developers and those new to programming, enhancing collaboration between different teams involved in infrastructure management.
ruby # Example: Connecting to a network device using NetSSH require 'net/ssh' hostname = '192.168.1.1' username = 'admin' password = 'secret' Net::SSH.start(hostname, username, password: password) do |ssh| result = ssh.exec!("show interface status") puts result end
1.2. Rich Ecosystem of Libraries and Gems
Ruby boasts an extensive ecosystem of libraries and gems (packages) that can significantly expedite network automation tasks. Gems like net-ssh, net-telnet, and net-http offer pre-built modules for SSH connections, Telnet interactions, and HTTP requests, respectively. This allows developers to focus on implementing specific automation logic rather than dealing with low-level networking intricacies.
2. Getting Started with Ruby for Network Automation
2.1. Installation and Setup
To begin utilizing Ruby for network automation, you need to have Ruby installed on your system. You can download the installer for your operating system from the official Ruby website (https://www.ruby-lang.org). Once Ruby is installed, you can install required gems using the following commands:
bash gem install net-ssh gem install net-telnet gem install net-http
2.2. Connecting to Network Devices
Let’s start with a basic example of connecting to a network device using the net-ssh gem.
ruby require 'net/ssh' hostname = '192.168.1.1' username = 'admin' password = 'secret' Net::SSH.start(hostname, username, password: password) do |ssh| result = ssh.exec!("show interface status") puts result end
In this example, we first require the net/ssh library. Then, we provide the hostname, username, and password for the network device we want to connect to. The Net::SSH.start block establishes the SSH connection, and within the block, we execute the command show interface status and print the result.
2.3. Automating Network Configuration Changes
One of the most common use cases for network automation is making configuration changes across multiple devices. Let’s see how we can use Ruby to automate such tasks.
<p><span style="font-weight: 400;">In the fast-paced world of network and infrastructure management, automation has become a cornerstone for efficiency, scalability, and reliability. Among the array of programming languages available for this purpose, Ruby stands out as a versatile and powerful option. Its elegant syntax, extensive libraries, and community support make it an ideal choice for simplifying complex infrastructure management tasks. In this blog post, we will delve into how Ruby can be utilized for network automation, exploring its benefits and providing practical insights through code samples.</span></p> <p> </p> <h2><span style="font-weight: 400;">Why Ruby for Network Automation?</span></h2> <h3><span style="font-weight: 400;">Clean and Intuitive Syntax</span></h3> <p><span style="font-weight: 400;">One of the key reasons why Ruby is favored for network automation is its clean and intuitive syntax. The language's syntax is designed to be easily readable, resembling natural language to a significant extent. This aspect makes it approachable for both experienced developers and those new to programming, enhancing collaboration between different teams involved in infrastructure management.</span></p> <pre class="EnlighterJSRAW" data-enlighter-language="generic">ruby # Example: Connecting to a network device using NetSSH require 'net/ssh' hostname = '192.168.1.1' username = 'admin' password = 'secret' Net::SSH.start(hostname, username, password: password) do |ssh| result = ssh.exec!("show interface status") puts result end </pre> <h3><span style="font-weight: 400;">Rich Ecosystem of Libraries and Gems</span></h3> <p><span style="font-weight: 400;">Ruby boasts an extensive ecosystem of libraries and gems (packages) that can significantly expedite network automation tasks. Gems like net-ssh, net-telnet, and net-http offer pre-built modules for SSH connections, Telnet interactions, and HTTP requests, respectively. This allows developers to focus on implementing specific automation logic rather than dealing with low-level networking intricacies.</span></p> <p> </p> <h2><span style="font-weight: 400;">Getting Started with Ruby for Network Automation</span></h2> <h3><span style="font-weight: 400;">Installation and Setup</span></h3> <p><span style="font-weight: 400;">To begin utilizing Ruby for network automation, you need to have Ruby installed on your system. You can download the installer for your operating system from the official Ruby website (https://www.ruby-lang.org). Once Ruby is installed, you can install required gems using the following commands:</span></p> <pre class="EnlighterJSRAW" data-enlighter-language="generic">bash gem install net-ssh gem install net-telnet gem install net-http </pre> <h3><span style="font-weight: 400;">Connecting to Network Devices</span></h3> <p><span style="font-weight: 400;">Let's start with a basic example of connecting to a network device using the net-ssh gem.</span></p> <pre class="EnlighterJSRAW" data-enlighter-language="generic">ruby require 'net/ssh' hostname = '192.168.1.1' username = 'admin' password = 'secret' Net::SSH.start(hostname, username, password: password) do |ssh| result = ssh.exec!("show interface status") puts result end </pre> <p><span style="font-weight: 400;">In this example, we first require the net/ssh library. Then, we provide the hostname, username, and password for the network device we want to connect to. The Net::SSH.start block establishes the SSH connection, and within the block, we execute the command show interface status and print the result.</span></p> <p> </p> <h3><span style="font-weight: 400;">Automating Network Configuration Changes</span></h3> <p><span style="font-weight: 400;">One of the most common use cases for network automation is making configuration changes across multiple devices. Let's see how we can use Ruby to automate such tasks.</span></p> <p><br /><br /></p>
In this example, we define an array of devices and their credentials, as well as an array of configuration changes to be applied. The script then iterates through each device, establishes an SSH connection, executes the configuration change commands, and prints a confirmation message.
2.4. Retrieving Network Device Information
Ruby can also be used to gather information from network devices, such as interface status, routing tables, or device statistics.
ruby require 'net/telnet' hostname = '192.168.1.1' username = 'admin' password = 'secret' telnet = Net::Telnet.new( 'Host' => hostname, 'Username' => username, 'Password' => password ) output = telnet.cmd('show interface status') puts output telnet.close
In this example, we use the net-telnet gem to establish a Telnet connection to the network device. We provide the hostname, username, and password. The telnet.cmd method is used to send the command and retrieve the output.
3. Benefits of Using Ruby for Network Automation
3.1. Rapid Development and Prototyping
Ruby’s concise and expressive syntax allows network engineers to quickly prototype automation scripts and solutions. This is particularly advantageous when dealing with evolving network environments or urgent tasks that demand swift action.
3.2. Community Support and Documentation
Ruby has a strong and active community, which means you can find ample resources, tutorials, and discussions related to network automation. This community support ensures that you’re not alone when facing challenges and can benefit from the experiences of others.
3.3. Integration with DevOps Pipelines
Integrating network automation scripts written in Ruby into your DevOps pipelines is seamless. Ruby scripts can be integrated with tools like Jenkins, GitLab CI/CD, and Ansible, allowing for continuous integration and deployment of network configurations.
3.4. Extensibility and Customization
Ruby’s object-oriented nature and dynamic capabilities enable developers to create highly customizable automation solutions. You can build libraries, frameworks, and reusable components tailored to your organization’s specific network infrastructure.
Conclusion
Network automation has become essential for managing modern, complex infrastructures efficiently. Ruby’s elegant syntax, rich ecosystem of libraries, and supportive community make it a powerful tool for simplifying network automation tasks. By harnessing the capabilities of Ruby, network engineers and administrators can streamline configuration changes, gather device information, and seamlessly integrate automation into their workflow. Whether you’re a seasoned developer or new to programming, Ruby opens doors to enhanced infrastructure management through automation. So, why not explore the world of Ruby for network automation and elevate your infrastructure management game?
Table of Contents
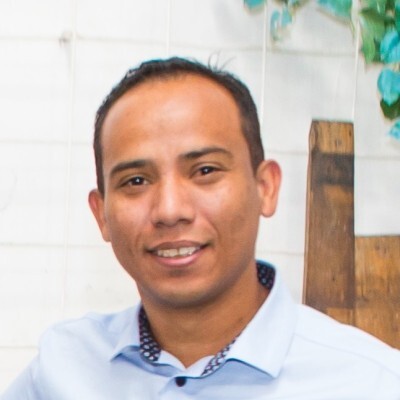
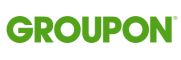