What is the ‘require’ keyword in Ruby?
In Ruby, the `require` keyword is a fundamental part of the language used for including external libraries, also known as gems or modules, into your Ruby code. It allows you to make the functionality of these external resources available in your program, enhancing Ruby’s capabilities and enabling you to use pre-written code and libraries developed by the Ruby community.
Here’s how the `require` keyword works and its key features:
- Loading External Libraries:
When you use `require` followed by the name of a gem or module, Ruby searches for and loads the corresponding library file. This file typically has the same name as the gem or module but with a `.rb` extension.
```ruby require 'json' ```
In this example, `require ‘json’` loads Ruby’s JSON library, allowing you to work with JSON data in your code.
- Loading Custom Code:
You can also use `require` to load your custom Ruby files. This is particularly useful when you want to organize your code into multiple files or reuse code across different parts of your application.
```ruby require_relative 'my_custom_module' ```
Here, `require_relative` loads a Ruby file called `my_custom_module.rb` from the same directory as your script.
- Preventing Duplicate Loading:
Ruby ensures that a library is loaded only once, even if you use `require` multiple times. This prevents unnecessary duplication and conflicts in your code.
```ruby require 'json' require 'json' # No duplicate loading occurs ```
- Load Paths:
Ruby maintains a list of directories known as the “load path” that it searches when looking for library files specified with `require`. You can add custom directories to the load path using `$LOAD_PATH` or the `$:` global variable.
```ruby $LOAD_PATH << '/path/to/my/libraries' require 'my_custom_module' ```
This allows you to organize your libraries in a structured manner and load them using `require`.
The `require` keyword in Ruby is essential for importing external libraries and custom code, making them accessible within your program. It plays a crucial role in code organization, reuse, and maintaining the modularity of Ruby applications by allowing you to harness the power of the Ruby ecosystem and community-contributed gems.
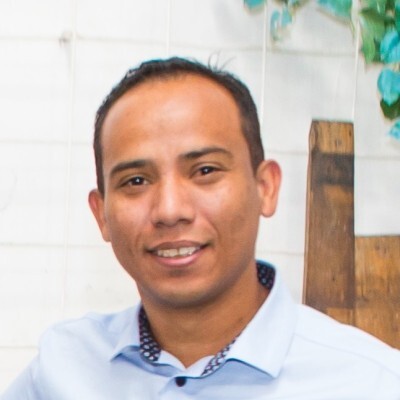
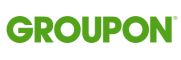