Unlocking the Power of Customization in SAP Systems through ABAP Programming
SAP ABAP (Advanced Business Application Programming) is a high-level programming language developed by the German software company SAP. It is integral to the customization and functionality of SAP systems and lies at the heart of SAP software packages. This expertise makes SAP developers highly sought after in the IT industry. This article aims to explore the essentials of SAP ABAP programming, shedding light on why many companies are eager to hire SAP developers, and delving deeper into some of the language’s more advanced features.
Introduction to SAP ABAP
SAP ABAP, the primary language in which many SAP applications are written, provides a platform for application development and runtime environment, including essential services for business applications. SAP systems such as SAP ECC and SAP S/4HANA have countless ABAP programs running behind the scenes that allow for the customization and enhancement of system functionalities. As a result of this wide utilization and the need for customization in SAP systems, businesses are increasingly looking to hire SAP developers proficient in ABAP. Their expertise in running and optimizing these applications is invaluable to fully leverage the potential of SAP’s software solutions.
The Basics of SAP ABAP Programming
Like any programming language, ABAP has its syntax and conventions that programmers need to follow to create successful, functional programs.
Data Types and Data Objects
In ABAP, data types define the characteristics of the data, such as length and format. Some common data types include:
– C (Character): Used for alphanumeric characters, with a maximum length of 65535.
– N (Numeric Text): Used for numeric texts, with a maximum length of 65535.
– I (Integer): Used for whole numbers.
– F (Floating-Point Number): Used for decimal numbers.
– D (Date): Used for dates in the format YYYYMMDD.
Data objects are the instances of data types. For example, you might define a character data type and then create a data object called CUSTOMER_NAME of that type.
```ABAP DATA: CUSTOMER_NAME TYPE C LENGTH 30. ```
Operators
ABAP supports a range of operators for performing operations such as arithmetic calculations, comparisons, and logical operations. Some common operators include:
– Arithmetic: + (Addition), – (Subtraction), * (Multiplication), / (Division).
– Comparison: = (Equal to), <> (Not equal to), < (Less than), > (Greater than), <= (Less than or equal to), >= (Greater than or equal to).
– Logical: AND, OR, NOT.
Control Structures
Control structures allow you to control the flow of execution in your programs. The most common structures include IF…ELSE…ENDIF, CASE…ENDCASE, and LOOP…ENDLOOP.
Example of an IF statement:
```ABAP IF score >= 50. WRITE: 'You passed'. ELSE. WRITE: 'You failed'. ENDIF. ```
Subroutines and Function Modules
Subroutines are blocks of code that can be called from anywhere within a program. They can be defined using the FORM…ENDFORM statements.
```ABAP FORM display_message. WRITE: 'Hello, World!'. ENDFORM. ```
Function Modules are similar to subroutines but are more powerful. They are defined in the ABAP Function Builder and can be called from any program within the SAP system.
Beyond the Basics: Advanced SAP ABAP Programming
After mastering the basics, you can start to explore some of the more advanced features of ABAP.
Object-Oriented Programming
ABAP supports Object-Oriented Programming (OOP), which allows you to create reusable and modular code. This involves concepts such as classes, objects, inheritance, and polymorphism.
An example of defining a class and creating an object:
```ABAP CLASS class_name DEFINITION. PUBLIC SECTION. METHODS: method_name. ENDCLASS. CLASS class_name IMPLEMENTATION. METHOD method_name. WRITE: 'This is a method'. ENDMETHOD. ENDCLASS. DATA: object_name TYPE REF TO class_name. CREATE OBJECT object_name. CALL METHOD object_name->method_name. ```
ALV Programming
ABAP List Viewer (ALV) is a powerful tool for creating interactive reports. It provides many standard functionalities such as sorting, filtering, and grouping.
Example of creating an ALV report:
```ABAP DATA: lt_data TYPE TABLE OF flightinfo, lo_alv TYPE REF TO cl_salv_table. SELECT * FROM sflight INTO TABLE lt_data. cl_salv_table=>factory( IMPORTING r_salv_table = lo_alv CHANGING t_table = lt_data ). lo_alv->display( ). ```
BAPIs and RFCs
BAPI (Business Application Programming Interface) and RFC (Remote Function Call) are used to communicate with SAP systems. They allow you to access data and execute functions in other SAP systems or external systems.
Example of calling a BAPI:
```ABAP DATA: lt_flightdata TYPE TABLE OF bapisflight, lv_return TYPE bapireturn1. CALL FUNCTION 'BAPI_FLIGHT_GETLIST' TABLES flightlist = lt_flightdata EXCEPTIONS system_failure = 1 communication_failure = 2. IF sy-subrc <> 0. WRITE: / 'Error occurred'. ELSE. LOOP AT lt_flightdata INTO DATA(lv_flightdata). WRITE: / lv_flightdata-carrid, lv_flightdata-connid, lv_flightdata-fldate. ENDLOOP. ENDIF. ```
Conclusion
SAP ABAP programming can be as straightforward or complex as you make it. From the basics of data types and control structures to the intricacies of object-oriented programming and ALV reporting, ABAP is a comprehensive language that offers plenty of possibilities for customization and enhancement in the SAP environment. Mastering ABAP can significantly enhance your skills as an SAP professional, making you an excellent candidate for businesses looking to hire SAP developers. As such, proficiency in ABAP can open new avenues for development and innovation, providing you with a competitive edge in the SAP market.
Table of Contents
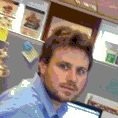
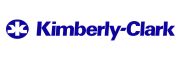