Hire Software Developers Interview Guide
Software developers are the architects of digital solutions, playing a vital role in creating applications, systems, and platforms. When hiring software developers, it’s crucial to assess their proficiency in programming languages, problem-solving abilities, and understanding of software development methodologies. This guide will help you navigate the hiring process effectively, enabling you to identify candidates with the right skills for your software development projects.
1. Reasons to Hire Software Developers
Embarking on the journey to hire software developers? Here are key reasons to consider:
1.1 Versatility
Software developers are versatile professionals capable of working across various domains, from web and mobile development to system architecture and artificial intelligence.
1.2 Problem-Solving Skills
Proficient software developers excel in problem-solving, tackling complex challenges and devising efficient and scalable solutions.
1.3 Collaboration
Software developers are adept at collaborating with cross-functional teams, contributing to the entire software development lifecycle.
2. How to Hire Software Developers
Follow these steps for a successful software developer hiring process:
2.1 Job Requirements
Define specific job prerequisites, emphasizing skills in programming languages, software development methodologies, and collaboration.
2.2 Search Channels
Utilize CloudDevs’ expertise to connect with potential software developers. Leverage job postings, online platforms, and tech communities to discover talented individuals.
2.3 Screening
Scrutinize candidates’ programming proficiency, problem-solving abilities, and experience with collaborative development. Utilize an asynchronous video interview platform to make your recruitment process more convehttps://founderstory.io/top-asynchronous-video-interview-platforms/nient.
2.4 Technical Assessment
Develop a comprehensive technical assessment, including coding challenges and real-world scenarios related to software development.
3. Core Skills of Software Developers to Look For
When evaluating software developers, focus on these core skills:
- Programming Languages: Proficiency in languages like Python, Java, JavaScript, C++, or others depending on project requirements.
- Problem-Solving: An aptitude for identifying challenges and devising efficient and scalable solutions.
- Software Development Methodologies: Understanding of Agile, Scrum, or other development methodologies.
- Version Control: Knowledge of version control systems like Git for collaborative development.
- Collaboration: Ability to work seamlessly with cross-functional teams and communicate effectively.
4. Overview of the Software Developer Hiring Process
Here’s an overview of the software developer hiring process:
4.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking in software developers.
4.2 Crafting Compelling Job Descriptions
Create engaging job descriptions that accurately convey the role, emphasizing software development-specific skills required.
4.3 Crafting Software Developer Interview Questions
Develop a comprehensive set of interview questions covering programming proficiency, problem-solving aptitude, and relevant technologies.
5. Sample Software Developer Interview Questions and Answers
Explore these sample questions with detailed answers to assess candidates’ software development skills:
Q1. Explain the principles of object-oriented programming (OOP) and provide an example.
A:
Object-oriented programming (OOP) is a programming paradigm based on the concept of “objects,” which can encapsulate data and behavior. Principles include encapsulation, inheritance, and polymorphism. Here’s an example in Java:
// Sample Answer: Java code demonstrating OOP principles class Animal { String name; public Animal(String name) { this.name = name; } public void speak() { System.out.println("Animal speaks"); } } class Dog extends Animal { public Dog(String name) { super(name); } @Override public void speak() { System.out.println("Dog barks"); } } public class Main { public static void main(String[] args) { Animal myDog = new Dog("Buddy"); myDog.speak(); // Output: Dog barks } }
Q2. Implement a function in Python to find the factorial of a number.
A:
# Sample Answer: Python function to find factorial def factorial(n): if n == 0 or n == 1: return 1 else: return n * factorial(n-1) # Example usage: result = factorial(5) # Result: 120 print(result)
Q3. Describe the difference between synchronous and asynchronous programming.
A:
Synchronous programming executes tasks one at a time, waiting for each to complete before moving on. Asynchronous programming allows tasks to overlap, continuing with the next task while waiting for the completion of the current one.
Q4. Explain the purpose of dependency injection in software development.
A:
Dependency injection is a design pattern where the dependencies of a class are injected from the outside rather than created within the class. It promotes loose coupling and makes the code more testable and maintainable.
Q5. Write a JavaScript function to fetch data from a RESTful API using the “fetch” API.
A:
// Sample Answer: JavaScript function to fetch data from a RESTful API function fetchData() { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error)); } // Example usage: fetchData();
Q6. Describe the principles of the SOLID design principles in object-oriented programming.
A:
- Single Responsibility Principle (SRP): A class should have only one reason to change.
- Open/Closed Principle (OCP): Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Objects of a superclass should be able to replace objects of a subclass without affecting the correctness of the program.
- Interface Segregation Principle (ISP): A class should not be forced to implement interfaces it does not use.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules. Both should depend on abstractions.
Q7. Write a SQL query to retrieve unique values from a column in a table.
A:
-- Sample Answer: SQL query to retrieve unique values SELECT DISTINCT column_name FROM table_name;
Q8. Explain the concept of a design pattern and provide an example.
A:
A design pattern is a general repeatable solution to a commonly occurring problem in software design. An example is the “Singleton Pattern,” where a class has only one instance and provides a global point of access to it.
Q9. How does garbage collection work in programming languages like Java?
A:
Garbage collection is an automatic memory management process. In Java, the Java Virtual Machine (JVM) automatically reclaims memory by identifying and deleting objects that are no longer reachable. It uses algorithms like the generational garbage collection algorithm to efficiently manage memory.
Q10. Describe the process of debugging and tools commonly used for debugging.
A:
Debugging is the process of finding and fixing errors in a program. Common debugging tools include:
- Print Statements: Inserting print statements in the code to trace the flow and values.
- IDE Debuggers: Integrated Development Environments (IDEs) provide debuggers to set breakpoints, inspect variables, and step through code.
- Logging: Using logging frameworks to record the program’s behavior during execution.
- Profiling Tools: Tools that analyze program performance to identify bottlenecks and areas for improvement.
6. Hiring Software Developers through CloudDevs
Step 1: Connect with CloudDevs
Initiate a conversation with a CloudDevs consultant to discuss your project requirements, preferred skills, and expected experience.
Step 2: Discover Your Ideal Match
Within a short timeframe, CloudDevs presents you with carefully selected software developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial
Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By leveraging the expertise of CloudDevs, you can effortlessly identify and hire exceptional software developers, ensuring your team possesses the skills required to build robust and innovative software solutions.
7. Conclusion
With these additional technical questions and insights at your disposal, you’re now well-prepared to assess software developers comprehensively. Whether you’re developing web applications, mobile apps, or complex software systems, securing the right software developers for your team is pivotal to the success of your software development projects.
Table of Contents
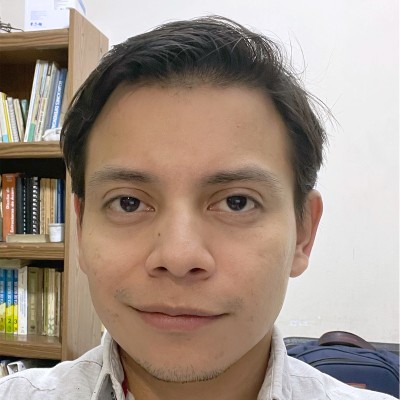
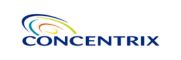