Animations and Transitions in Svelte: Adding Life to Your Web Apps
In the ever-evolving landscape of web development, user experience (UX) has become paramount. Users expect more than just static and bland interfaces; they crave dynamic, engaging, and visually appealing experiences. This is where animations and transitions come into play. They can add a touch of elegance, personality, and interactivity to your web applications, making them more enjoyable and memorable for users. In this article, we will delve into the world of animations and transitions in Svelte, a modern JavaScript framework that provides a streamlined approach to building web applications.
Why Animations and Transitions Matter
Imagine visiting a website that instantly greets you with a burst of color and movement as elements smoothly slide into place. This immediate engagement captures your attention and invites you to explore further. Animations and transitions serve as a visual storytelling tool, guiding users through various interactions and actions. They can convey changes in state, highlight important information, and create a sense of fluidity, all of which contribute to a richer and more intuitive user experience.
Getting Started with Svelte Animations
Svelte is renowned for its efficiency and simplicity in building user interfaces. When it comes to adding animations and transitions, Svelte continues to shine with its straightforward approach. Let’s dive into the essential steps to incorporate animations and transitions into your Svelte applications.
1. Installing Svelte
If you haven’t already, start by installing Svelte using npm:
bash npm install -g degit degit sveltejs/template svelte-app cd svelte-app npm install
2. Creating a Basic Animation
To begin, let’s create a simple fade-in animation for an element. In your Svelte component (e.g., MyComponent.svelte), add the following code:
svelte <script> import { fade } from 'svelte/transition'; </script> <style> .box { width: 100px; height: 100px; background-color: #3498db; opacity: 0; /* Start with zero opacity */ } </style> <div class="box" transition:fade> <!-- Content here --> </div>
In this example, the fade transition gradually changes the opacity of the element from 0 to 1, creating a smooth fade-in effect.
3. Transitioning Between States
Transitions become particularly valuable when switching between different states in your application. For instance, let’s consider a scenario where you want to show or hide a section when a button is clicked.
svelte <script> let isVisible = false; function toggleVisibility() { isVisible = !isVisible; } </script> <button on:click={toggleVisibility}>Toggle Section</button> {#if isVisible} <div transition:slide> <!-- Content of the section --> </div> {/if}
Here, the slide transition animates the appearance and disappearance of the section with a sliding motion.
4. Customizing Transitions
Svelte offers a variety of built-in transitions like fade, slide, scale, and more. However, you can also create custom transitions to fit your design aesthetics perfectly. Let’s create a custom spin transition as an example.
svelte <script> import { spring } from 'svelte/motion'; export let duration = 400; // Duration of the transition in milliseconds export let angle = 360; // Angle of rotation in degrees </script> <style> div { transform-origin: center; } </style> {#if visible} <div style="transform: rotate({spring(angle, { stiffness: 0.1, damping: 0.6 })}deg);" transition:spring > <!-- Content --> </div> {/if}
In this example, the spring transition applies a rotation animation to the element.
Advanced Animation Techniques
As you become more comfortable with Svelte animations and transitions, you can explore advanced techniques to create even more captivating user experiences.
1. Delayed Animations
Adding delays to animations can enhance the overall flow of your application. For instance, you can stagger the entrance of multiple elements to avoid overwhelming the user with too much information at once.
svelte <script> import { fly } from 'svelte/transition'; let items = ['Item 1', 'Item 2', 'Item 3']; </script> {#each items as item, i (item)} <div class="item" transition:fly="{{ delay: i * 100 }}" > {item} </div> {/each}
Here, each item fades in with a slight delay, creating a visually appealing sequence.
2. Easing Functions
Easing functions determine the pace at which an animation accelerates or decelerates. Svelte provides a range of easing options that can be applied to transitions for added realism.
svelte <script> import { elasticOut } from 'svelte/easing'; let isVisible = false; </script> <button on:click={() => isVisible = !isVisible}>Toggle</button> {#if isVisible} <div class="box" transition:fly="{{ easing: elasticOut, duration: 800 }}" > <!-- Content --> </div> {/if}
In this example, the elasticOut easing function creates a bouncy effect during the transition.
Conclusion
Animations and transitions are powerful tools in modern web development, enhancing the user experience and bringing life to your applications. With Svelte’s intuitive approach to incorporating animations, you can easily create engaging interactions that captivate your users. Whether you’re a beginner or an experienced developer, exploring animations and transitions in Svelte opens up a realm of possibilities for creating visually stunning and delightful web apps. So, go ahead and add that touch of magic to your projects, and watch as your applications come to life before your users’ eyes!
Table of Contents
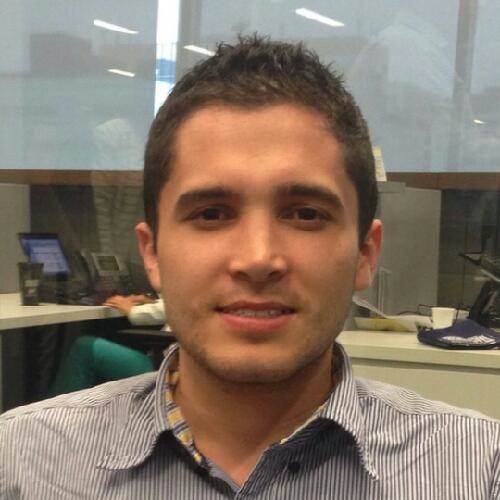
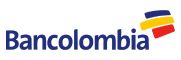