Svelte and API Integration: Building Data-driven Web Applications
In the world of web development, creating interactive and data-driven applications is essential to engage users and provide meaningful experiences. Svelte, a revolutionary front-end framework, offers a powerful approach to building such applications with seamless API integration. In this blog, we will embark on a journey to understand how Svelte and API integration come together to create dynamic web applications. Through code samples, examples, and insights, you’ll gain the knowledge you need to craft your own data-driven UIs.
1. Introduction to Svelte and API Integration
What is Svelte?
Svelte is a modern JavaScript framework that shifts the paradigm of front-end development. Unlike traditional frameworks that run in the browser, Svelte shifts much of the work to build-time, resulting in highly efficient and performant applications. It compiles components into optimized JavaScript code, leading to faster loading times and smoother interactions. This efficiency makes Svelte an ideal choice for creating data-driven web applications.
The Importance of API Integration
APIs (Application Programming Interfaces) serve as bridges that allow different software applications to communicate and share data. Integrating APIs into your web application empowers it to fetch and display real-time data from external sources, such as databases or third-party services. This integration is crucial for building dynamic and engaging web applications that provide users with up-to-date information and interactive features.
2. Setting Up Your Development Environment
Installing Node.js and NPM
Before we dive into building with Svelte and API integration, ensure you have Node.js and NPM (Node Package Manager) installed on your system. These tools will facilitate package management and the development server.
bash # Check if Node.js and NPM are installed node -v npm -v # If not installed, download from https://nodejs.org/
Creating a New Svelte Project
To start a new Svelte project, use the Svelte template from the command line:
bash npx degit sveltejs/template svelte-app cd svelte-app npm install npm run dev
This sets up a basic Svelte application structure and starts the development server, allowing you to see your application in action.
3. Fetching Data from an API
Using the fetch API for Data Retrieval
The fetch API is a powerful tool to make network requests in a browser environment. It allows you to fetch data from a given URL and handle the resulting Promise to access the response.
javascript // Fetching data from an API fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { console.log(data); // Further data processing and UI updates }) .catch(error => { console.error('Error fetching data:', error); });
Handling Promises and Asynchronous Operations
API requests are asynchronous operations, meaning they don’t block the main thread. Svelte’s reactivity and component model make it easy to handle asynchronous data.
svelte <script> let apiData; fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { apiData = data; }) .catch(error => { console.error('Error fetching data:', error); }); </script> {#if apiData} <ul> {#each apiData as item (item.id)} <li>{item.name}</li> {/each} </ul> {:else} <p>Loading data...</p> {/if}
4. Creating Dynamic UI Components
Utilizing Svelte’s Reactive Statements
Svelte’s reactivity system allows you to declaratively update the UI in response to data changes. The framework automatically detects dependencies and updates the DOM efficiently.
svelte <script> let count = 0; function increment() { count += 1; } </script> <button on:click={increment}>Increment</button> <p>Count: {count}</p>
Binding Data to UI Elements
Svelte’s binding syntax enables two-way data binding between the UI and your component’s state. Changes in UI elements are reflected back to the component’s data.
svelte <script> let name = 'John Doe'; </script> <input bind:value={name} placeholder="Enter your name" /> <p>Hello, {name}!</p>
5. Displaying API Data in Your Application
Rendering API Responses in Svelte Components
Svelte components can be used to encapsulate UI logic and rendering. You can pass API data as props to components for dynamic rendering.
svelte <script> export let items; </script> <ul> {#each items as item (item.id)} <li>{item.name}</li> {/each} </ul>
Mapping Data to UI Elements
Svelte’s #each block allows you to iterate through arrays and render UI elements for each item.
svelte <script> let apiData = []; fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { apiData = data; }) .catch(error => { console.error('Error fetching data:', error); }); </script> {#each apiData as item (item.id)} <p>{item.name}</p> {/each}
6. Building Interactive User Interfaces
Adding Event Listeners with Svelte
Svelte’s event handling syntax simplifies adding event listeners to UI elements.
svelte <button on:click={handleClick}>Click me</button> <script> function handleClick() { alert('Button clicked!'); } </script>
Responding to User Actions
User interactions can trigger changes in the component’s state, leading to updates in the UI.
svelte <script> let count = 0; function increment() { count += 1; } </script> <button on:click={increment}>Increment</button> <p>Count: {count}</p>
7. State Management and Reactivity
Understanding Svelte’s Stores
Svelte offers stores for managing application state. Stores are reactive, ensuring that changes propagate throughout the application.
svelte <script> import { writable } from 'svelte/store'; // Create a writable store const count = writable(0); </script> <button on:click={() => $count += 1}>Increment</button> <p>Count: {$count}</p>
Managing and Updating Application State
Svelte’s store methods like set and $ allow you to update and access store values.
svelte <script> import { writable } from 'svelte/store'; const count = writable(0); function increment() { count.update(n => n + 1); } </script> <button on:click={increment}>Increment</button> <p>Count: {$count}</p>
8. Enhancing UI with Transitions and Animations
Applying Transition Effects to Elements
Svelte provides built-in transition effects to add smooth animations to UI changes.
svelte <script> let visible = false; function toggle() { visible = !visible; } </script> <button on:click={toggle}>Toggle</button> {#if visible} <p transition:fade>Hello, Svelte!</p> {/if}
Creating Smooth and Engaging Animations
You can use Svelte’s animation features to create custom animations for your UI elements.
svelte <script> import { fade } from 'svelte/transition'; let visible = false; function toggle() { visible = !visible; } </script> <button on:click={toggle}>Toggle</button> {#if visible} <p transition:fade>Hello, Svelte!</p> {/if} <style> p { transition: opacity 0.5s; } </style>
9. Error Handling and Feedback
Implementing Error Handling for API Requests
Proper error handling ensures that your application gracefully handles API failures.
svelte <script> let apiData = []; let error = null; fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('API request failed'); } return response.json(); }) .then(data => { apiData = data; }) .catch(err => { error = err.message; }); </script> {#if error} <p>Error: {error}</p> {:else if apiData.length > 0} <ul> {#each apiData as item (item.id)} <li>{item.name}</li> {/each} </ul> {:else} <p>Loading data...</p> {/if}
Providing User Feedback
Feedback informs users about the state of their interactions. Use Svelte to provide meaningful feedback.
svelte <script> let isSubmitting = false; function handleSubmit() { isSubmitting = true; // Simulate API request setTimeout(() => { isSubmitting = false; // Display success message }, 2000); } </script> <form on:submit|preventDefault={handleSubmit}> <button type="submit" disabled={isSubmitting}> {#if isSubmitting} Submitting... {:else} Submit {/if} </button> </form>
10. Deploying Your Svelte App
Building and Bundling Your Application
Before deployment, build your Svelte application to create optimized and minified bundles.
bash npm run build
This generates production-ready files in the public directory.
Hosting and Deployment Options
Various hosting platforms offer easy ways to deploy your Svelte application, such as Netlify, Vercel, or GitHub Pages. Choose the platform that best suits your needs and follow their deployment instructions.
Conclusion
In conclusion, the combination of Svelte’s efficient framework and seamless API integration opens the door to creating powerful, data-driven web applications. By following the steps outlined in this blog, you can harness the capabilities of Svelte to build responsive, interactive, and dynamic user interfaces that fetch and display real-time data from APIs. With Svelte’s reactivity, component model, and transition effects, you’ll be well-equipped to create engaging web applications that provide exceptional user experiences. So, roll up your sleeves and start your journey into the world of Svelte and API integration – where innovation meets usability, and creativity flourishes. Happy coding!
Table of Contents
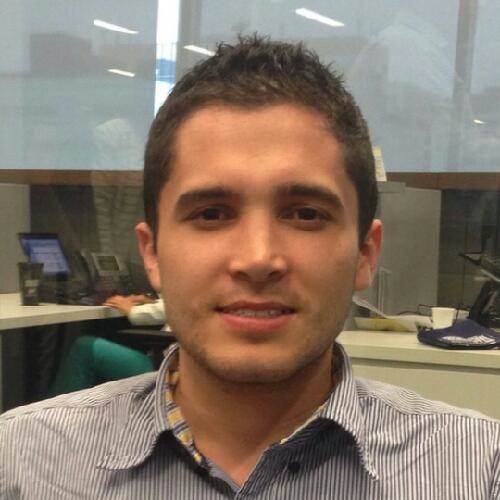
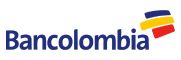