Svelte and Authentication: Implementing User Login and Authorization
In the world of web development, user authentication and authorization are essential components of building secure and functional applications. Svelte, a modern JavaScript framework, provides an elegant solution for creating interactive and efficient user interfaces. In this blog post, we’ll delve into the process of implementing user login and authorization in Svelte applications. We’ll cover the key concepts, step-by-step instructions, and code samples to guide you through creating a secure authentication system.
Understanding User Authentication and Authorization:
Before we dive into the implementation details, let’s clarify the terms “authentication” and “authorization.” Authentication is the process of verifying the identity of a user, usually through a username and password. Once a user is authenticated, authorization comes into play, determining what actions and resources they are allowed to access based on their role or permissions.
Creating a Svelte Project:
To get started, make sure you have Node.js installed. If not, download and install it from the official website. Once Node.js is installed, you can create a new Svelte project using the following commands:
bash npx degit sveltejs/template svelte-authentication cd svelte-authentication npm install
This will create a new Svelte project named “svelte-authentication” and install the necessary dependencies.
Setting Up a Backend:
For this tutorial, we’ll use a basic JSON-based backend for simplicity. However, in a real-world scenario, you’d likely integrate with a more robust backend or API for authentication. Create a file named “users.json” in your project’s root directory with the following content:
json [ { "id": 1, "username": "user123", "password": "password123", "role": "user" }, { "id": 2, "username": "admin", "password": "admin123", "role": "admin" } ]
Creating the Login Component:
In Svelte, components are the building blocks of the user interface. Let’s create a Login component that allows users to enter their credentials and submit the form. Inside the “src” folder, create a new file named “Login.svelte”:
svelte <script> let username = ""; let password = ""; async function handleLogin() { const response = await fetch("/api/login", { method: "POST", headers: { "Content-Type": "application/json" }, body: JSON.stringify({ username, password }) }); if (response.ok) { // Redirect user to dashboard or authorized route } else { // Handle login error } } </script> <form on:submit|preventDefault={handleLogin}> <label for="username">Username:</label> <input type="text" id="username" bind:value={username} /> <label for="password">Password:</label> <input type="password" id="password" bind:value={password} /> <button type="submit">Login</button> </form>
In this code, we’ve defined two variables, “username” and “password,” and bound them to the input fields. The “handleLogin” function sends a POST request to the “/api/login” endpoint with the provided credentials. If the response is successful, you can redirect the user to the appropriate route; otherwise, handle the login error.
Implementing Authentication Logic:
Now let’s create the authentication logic that verifies the user’s credentials and provides a token upon successful login. In the “src” folder, create a new file named “auth.js”:
javascript import users from "../users.json"; export function authenticateUser(username, password) { const user = users.find(u => u.username === username && u.password === password); if (user) { const { id, role } = user; const token = generateToken(id, role); return { token, role }; } return null; } function generateToken(userId, userRole) { // Generate a JWT token here or use any authentication mechanism }
In this module, the “authenticateUser” function checks if the provided username and password match any user in the “users” array. If a match is found, it generates a token using the “generateToken” function (which you would implement using a secure authentication method like JWT).
Routing and Authorization:
After a successful login, you’ll likely want to direct users to different routes based on their roles. Svelte doesn’t provide built-in routing, but you can use libraries like “svelte-routing” or “svelte-navigator” for this purpose.
For example, let’s assume you’re using “svelte-routing.” Install it using:
bash npm install svelte-routing
Then, create a protected route that requires authentication and specific authorization. In your “App.svelte” file:
svelte <script> import { Router, Route, Link } from "svelte-routing"; import Login from "./Login.svelte"; import Dashboard from "./Dashboard.svelte"; import AdminPanel from "./AdminPanel.svelte"; </script> <Router> <Route path="/" component={Login} /> <Route path="/dashboard" component={Dashboard} /> <Route path="/admin" component={AdminPanel} /> </Router>
In this example, the “/dashboard” route is accessible to all authenticated users, while the “/admin” route is only accessible to users with an “admin” role.
Conclusion
Implementing user login and authorization in Svelte applications is crucial for building secure and functional web applications. With the steps outlined in this blog post, you’ve learned how to create a user login component, authenticate users, and implement basic authorization based on roles. Remember that security is paramount, and you should use secure authentication mechanisms like JWT in a production environment.
Svelte’s simplicity and efficiency make it an excellent choice for building dynamic and responsive user interfaces with robust authentication systems. By following best practices and staying informed about security updates, you can create web applications that provide a seamless and secure user experience. Happy coding!
Table of Contents
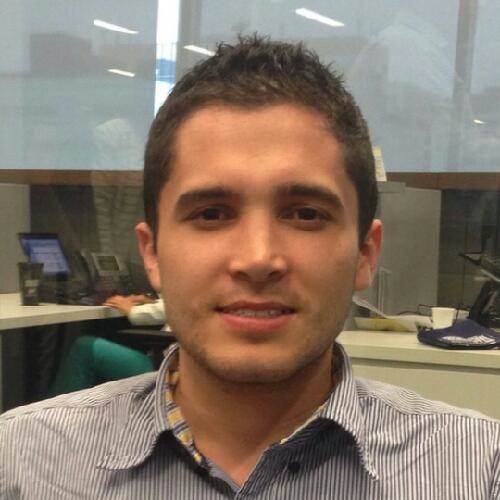
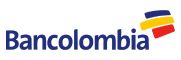