Creating Dynamic, High-Performance UIs with Svelte Reactive Components
Svelte, a radical new approach to building user interfaces, has seen a significant rise in popularity over the past few years. This has led to an increase in demand to hire Svelte developers, who can leverage this innovative tool to create cutting-edge web applications. Unlike traditional frameworks, Svelte compiles your code to tiny, imperative JavaScript modules at build time, leading to fast, efficient, and robust applications.
One of the most compelling features of Svelte is its reactive system. This system, which is a key reason why many businesses choose to hire Svelte developers, provides a simple and intuitive way to manage state in your applications. This blog post will introduce you to reactive components in Svelte and demonstrate how they can be used to build dynamic user interfaces (UIs). These benefits highlight why hiring Svelte developers could be a strategic move for your next project.
What is Svelte?
Svelte is a JavaScript framework developed by Rich Harris. It’s a tool for building fast, efficient, and reactive web applications. Instead of using techniques like virtual DOM diffing, Svelte writes code that surgically updates the DOM when the state of your application changes. This leads to incredibly performant applications that feel smooth and responsive to user interaction.
What are Reactive Components?
In Svelte, components are the building blocks of your application. Each component has its own state, and when this state changes, the component re-renders to reflect those changes. This is what we refer to as “reactive”. When a variable changes, anything that relies on that variable will be updated.
The beautiful thing about Svelte is how this reactivity is written. It’s very similar to writing regular JavaScript, which makes it incredibly intuitive. Any statement with a variable assignment that starts with `$:` is reactive. Let’s see how this works.
Basic Reactive Statement
Here’s a simple example of a reactive statement:
```svelte <script> let count = 0; $: double = count * 2; </script> <p>Count: {count}</p> <p>Double: {double}</p> <button on:click={() => count += 1}>Increment</button> ```
In the example above, whenever `count` changes, `double` will also change. Clicking the “Increment” button will increase `count` by one, and `double` will reactively update.
Reactive Dependencies
Reactive statements in Svelte are smart. They understand their dependencies and only run if one of their dependencies has changed.
```svelte <script> let a = 1; let b = 2; $: sum = a + b; $: product = a * b; </script> <p>Sum: {sum}</p> <p>Product: {product}</p> ```
In this example, `sum` and `product` are reactive statements dependent on `a` and `b`. If either `a` or `b` changes, the corresponding reactive statement will run and the UI will update accordingly.
Reactive Statements and Conditionals
Reactive statements can also handle conditions, which are very powerful for managing complex state relationships.
```svelte <script> let weather = 'sunny'; $: outfit = weather === 'sunny' ? 'T-shirt' : 'Coat'; </script> <p>Weather: {weather}</p> <p>Outfit: {outfit}</p> <button on:click={() => weather = 'sunny'}>Sunny</button> <button on:click={() => weather = 'rainy'}>Rainy</button> ```
In the example above, the `outfit` is determined by the current `weather`. Clicking the “Sunny” or “Rainy” buttons will change the `weather`, and the `outfit` will update reactively.
Svelte Stores for Global State Management
For managing global state, Svelte provides a simple and intuitive solution: Stores. A Store is simply an object with a `subscribe` method that allows reactive components to get notified of changes.
```svelte import { writable } from 'svelte/store'; export const count = writable(0); ```
In the example above, we create a writable store named `count` with an initial value of 0. We can use this store in any component like this:
```svelte <script> import { count } from './store.js'; function increment() { $count += 1; } </script> <p>Count: {$count}</p> <button on:click={increment}>Increment</button> ```
In the example above, we use `$count` to access the value of the count store, and when the “Increment” button is clicked, the count increases by 1.
Svelte Await Block for Async Operations
Svelte also provides an efficient way to handle promises with the `{#await}` block. This makes it easier to manage asynchronous operations in our components.
```svelte <script> let promise = fetch('https://api.example.com/data'); </script> {#await promise} <p>Loading...</p> {:then data} <p>{data}</p> {:catch error} <p>{error.message}</p> {/await} ```
In the above example, while the promise is pending, “Loading…” is displayed. When the promise is resolved, the returned data is shown. If there is an error, the error message is displayed.
Conclusion
Svelte approach to reactivity provides an easy, intuitive, and efficient way to build dynamic UIs. Its reactive components allow us to build applications that are incredibly fast and efficient, yet straightforward to understand and maintain. By harnessing the power of reactive programming in Svelte, we can build complex applications with ease, ensuring that our code stays clean, readable, and efficient.
As a result of these advantages, many businesses are looking to hire Svelte developers to bring these benefits to their projects. Skilled Svelte developers can help companies create highly performant, user-friendly web applications.
So why not give Svelte a spin? With its powerful reactive system, component-based architecture, and performance benefits, it could be just the tool you need to create your next great web application. Or better yet, consider the value that hiring Svelte developers could add to your team and project.
Table of Contents
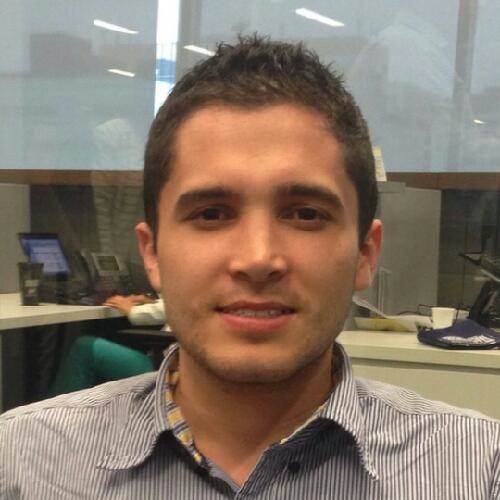
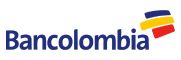