Building Better User Interfaces with Svelte Components
Developing sleek and interactive user interfaces (UI) is the bread and butter of any front-end web developer, which is one of the reasons many are choosing to hire Svelte developers. However, with the proliferation of various JavaScript frameworks, picking the right one to optimize your work can be daunting. Among the popular choices such as React, Vue, and Angular, a new player has been rapidly gaining ground – Svelte.
Svelte is a modern JavaScript compiler that helps developers to write and compile their code into efficient JavaScript that directly manipulates the DOM. This unique feature is causing an increasing number of companies to hire Svelte developers. Unlike other libraries and frameworks, Svelte does away with the need for a virtual DOM, resulting in faster performance and less memory consumption.
In this post, we’re going to explore one of the key features of Svelte: Components. These are another reason why companies are choosing to hire Svelte developers. We’ll delve into how we can leverage components to build reusable and efficient UI building blocks in Svelte. We’ll also walk through a few practical examples to further solidify our understanding. Whether you’re looking to hire Svelte developers or become one yourself, this knowledge is essential.
What are Svelte Components?
In Svelte, a component is a reusable piece of code that encapsulates HTML, CSS, and JavaScript. Each component is a self-contained entity, meaning it has its own state, behavior, and style. This encapsulation makes components highly reusable, maintainable, and testable.
Components are at the core of Svelte, making it easier to structure your application and reuse code. This brings a number of benefits, including faster development times and more consistent UIs.
Let’s understand this better with some examples.
Creating a Basic Svelte Component
First, let’s create a simple Svelte component.
- Create a new Svelte file with the extension `.svelte`. Let’s call it `Button.svelte`.
- Inside this file, write the following code:
```svelte <script> export let label = "Button"; </script> <button>{label}</button> <style> button { padding: 10px 20px; background-color: #4CAF50; color: white; border: none; cursor: pointer; } </style> ```
This code creates a button component with a customizable label. The export keyword makes the `label` variable a prop that you can pass when you use the `Button` component.
- To use this component, import it in another Svelte file:
```svelte <script> import Button from './Button.svelte'; </script> <Button label="Click me"/> ```
In this example, we imported the `Button` component and used it with the label “Click me”.
Interactivity in Svelte Components
Let’s make our components a bit more interactive by adding event handling. We’ll add functionality to our button to alert a message when clicked.
In `Button.svelte`, add an `onClick` event handler to the button:
```svelte <script> export let label = "Button"; function handleClick() { alert('Button clicked!'); } </script> <button on:click={handleClick}>{label}</button> ```
In this code, we added a `handleClick` function that shows an alert when the button is clicked. The `on:click` attribute binds the `click` event to the `handleClick` function.
Nesting Svelte Components
Svelte allows us to nest components inside other components, allowing for more complex UI structures.
Let’s create a `Card` component that uses our `Button` component. Create a new `Card.svelte` file and add the following code:
```svelte <script> import Button from './Button.svelte'; export let title = "Card Title"; export let content = "Card Content"; </script> <div class="card"> <h2>{title}</h2> <p>{content}</p> <Button label="Learn more" /> </div> <style> .card { padding: 20px; margin: 20px 0; background-color: #f5f5f5; border-radius: 5px; } </style> ```
In this code, we created a `Card` component with a title, content, and a button. The `Button` component is nested inside the `Card` component.
Passing Data Between Components
Passing data between components in Svelte is achieved using props. You’ve already seen how to pass data to a component, but what if a child component needs to send data back to a parent component?
Let’s modify our `Button` component to emit a custom event when it’s clicked:
```svelte <script> import { createEventDispatcher } from 'svelte'; export let label = "Button"; const dispatch = createEventDispatcher(); function handleClick() { dispatch('click', { clicked: true }); } </script> <button on:click={handleClick}>{label}</button> ```
Here, we used Svelte `createEventDispatcher` function to create a `dispatch` function. Inside the `handleClick` function, we used `dispatch` to emit a ‘click’ event with an accompanying data object.
In the parent `Card` component, we can listen for this event and handle it:
```svelte <script> import Button from './Button.svelte'; export let title = "Card Title"; export let content = "Card Content"; function handleButtonClick(event) { console.log('Button was clicked!', event.detail); } </script> <div class="card"> <h2>{title}</h2> <p>{content}</p> <Button label="Learn more" on:click={handleButtonClick} /> </div> ```
In this code, we added a `handleButtonClick` function to the `Card` component and used it to handle the ‘click’ event from the `Button` component.
Conclusion
This post barely scratches the surface of what’s possible with Svelte components, which is why many businesses are keen to hire Svelte developers. We’ve covered creating basic Svelte components, adding interactivity, nesting components, and passing data between components.
Svelte components are a powerful tool that skilled Svelte developers utilize to build reusable and efficient UI building blocks. By encapsulating HTML, CSS, and JavaScript code into self-contained units, Svelte components promote modularity, reusability, and maintainability in your web applications.
Whether you’re a business looking to hire Svelte developers, new to Svelte, or a seasoned pro, understanding and effectively utilizing components will significantly improve your productivity and code quality. So why wait? Start leveraging the power of Svelte components today!
Table of Contents
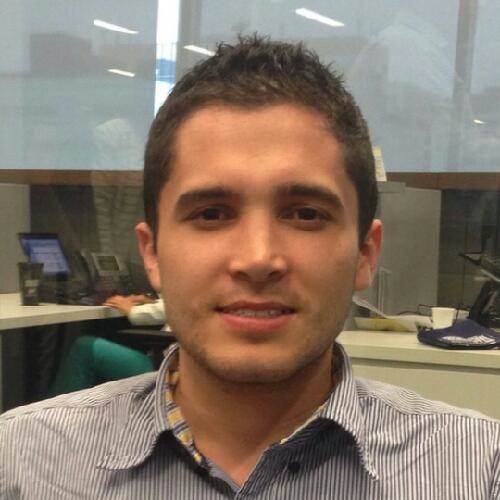
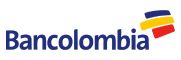