Revolutionizing UI Styling with Svelte: An Exploration of CSS-in-JS and Scoped CSS
When it comes to UI development, one critical aspect that developers, particularly those proficient in Svelte, pay close attention to is the styling of components. This determines the visual appeal of the user interface and the overall user experience of the application. In the world of component-based UI development, Svelte, a preferred tool for many professional developers available for hire, has made a name for itself due to its simplicity and elegance. It offers a refreshing alternative to more traditional methods of styling, a reason why many businesses are looking to hire Svelte developers. Svelte opens up new paradigms like CSS-in-JS and scoped CSS, further enhancing its reputation in the UI development world.
Understanding CSS-in-JS
CSS-in-JS is a styling technique where CSS is composed using JavaScript instead of defining styles in external stylesheets. The CSS rules are co-located with the JavaScript code that generates the DOM elements they are meant to style. This practice eliminates the context-switching between different files and enables more dynamic styling options.
```javascript <script> let color = 'blue'; function changeColor() { color = color === 'blue' ? 'red' : 'blue'; } </script> <button on:click={changeColor} style="color: {color};">Change my color</button> ```
In the above Svelte example, we’re dynamically setting the color of the button text using a variable `color`. When the button is clicked, the `changeColor` function is invoked, which toggles the color between ‘blue’ and ‘red’. The button’s color style is directly linked to the `color` variable. This is a simple example of CSS-in-JS, and it illustrates the power of being able to tie styles directly to your component’s state.
The Power of Scoped CSS
One common problem developers encounter with CSS is the global nature of styles. When you define a style in a CSS file, it’s applied across your entire application unless explicitly overridden. This can lead to tricky bugs and complex style hierarchies as your application grows.
Scoped CSS solves this problem by isolating styles to specific components. With scoped CSS, styles defined within a component only apply to that component and won’t unintentionally affect other parts of your application. This concept plays nicely with the component-based architecture of modern web development, as each component is a self-contained unit with its own styles.
```javascript <style> h1 { color: blue; } </style> <h1>This is a blue heading</h1> ```
In this Svelte example, the `h1` style is scoped to this specific component. If there’s another `h1` tag in a different component, it won’t be blue unless explicitly styled that way in its component.
Styling Components in Svelte
The simplicity of Svelte allows for flexible and intuitive ways to style your components. You can include a `<style>` tag directly in your .svelte component file, making it easy to see at a glance how a component is styled. Svelte will automatically scope this CSS to the component, so you don’t have to worry about style collisions.
```javascript <script> let isImportant = true; </script> <style> h1 { color: {isImportant ? 'red' : 'black'}; font-size: 2em; } </style> <h1>Hello world!</h1> ```
In this example, we’re using CSS-in-JS inside our `<style>` tag. The color of the `h1` tag will depend on the `isImportant` variable’s value. The ability to use JavaScript directly in your CSS opens up a world of possibilities for dynamic and reactive styles.
Svelte also provides support for CSS preprocessors like SCSS, LESS, and PostCSS, giving you access to features like variables, nesting, and mixins. This enables more powerful and expressive styles.
```javascript <style lang="scss"> $primaryColor: blue; h1 { color: $primaryColor; font-size: 2em; &:hover { color: darken($primaryColor, 10%); } } </style> <h1>Hello world!</h1> ```
Here, we’re using SCSS to define a color variable, a hover effect, and a nested style rule. This gives us more control over our styles and keeps our CSS DRY (Don’t Repeat Yourself).
The Advantage of Svelte Approach
The benefits of CSS-in-JS and scoped CSS are magnified in the Svelte reactive paradigm. With Svelte reactivity, your styles can respond in real-time to changes in your component’s state. This is perfect for creating engaging, interactive user interfaces.
Svelte approach to styling is simpler and more intuitive than other frameworks. There’s no need to learn a new CSS dialect or use a special API for scoped styles. You can just write regular CSS (or your favorite preprocessor), and Svelte takes care of the rest.
Moreover, the use of standard CSS makes it easier for designers and developers to work together, as they can communicate using a shared language. It’s also easier for new developers to get started with Svelte, as they don’t have to learn a unique way of handling styles.
Conclusion
Svelte approach to styling, through the use of CSS-in-JS and scoped CSS, provides a powerful, intuitive, and flexible way to style component-based user interfaces. This unique strength of Svelte is one of the many reasons why companies choose to hire Svelte developers. Whether you’re building a small interactive widget or a large-scale application, Svelte styling capabilities, enhanced by skilled Svelte developers, can handle it with grace and performance.
The Svelte innovative approach to styling illustrates that it’s not just another UI library, but a valuable tool that can bring simplicity and power to your web development workflow. This underscores the value you get when you hire Svelte developers for your projects. So if you haven’t yet, consider giving Svelte a try and hire Svelte developers to experience the difference yourself. Happy coding!
Table of Contents
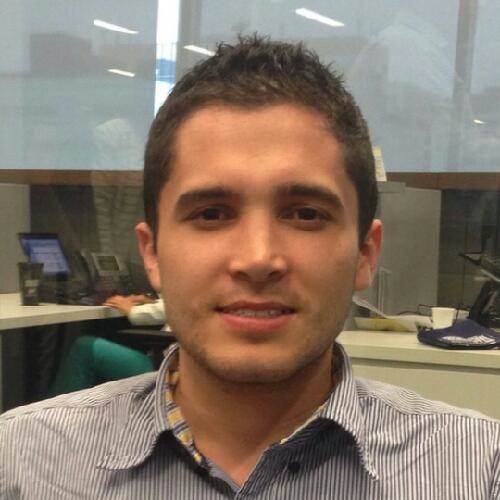
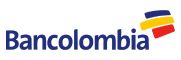