Svelte and Performance Optimization: Techniques for Faster Web Apps
In today’s digital landscape, where users demand seamless and fast experiences, web app performance optimization has become a crucial aspect of development. Slow-loading or sluggish web applications can lead to user frustration and abandonment. This is where Svelte, a cutting-edge JavaScript framework, comes into play. Svelte not only simplifies the development process but also offers several techniques to enhance web app performance. In this blog post, we will delve into various performance optimization techniques provided by Svelte that can help you create blazing-fast web apps.
Understanding the Performance Landscape
Before we dive into Svelte’s optimization techniques, it’s important to have a solid understanding of the factors that influence web app performance. Some of the key aspects to consider include:
Rendering Efficiency
Efficient rendering is essential for a smooth user experience. Rendering involves the process of translating your app’s logic and data into the visual elements that users see on their screens. Unoptimized rendering can result in unnecessary re-renders and increased processing time.
Bundle Size
The size of your JavaScript bundle greatly impacts the loading time of your web app. Larger bundles take longer to download and parse, leading to delayed interactions and slower performance.
Network Efficiency
Web apps rely on network requests to fetch data and assets. Minimizing the number of requests and optimizing the data transfer process can significantly improve loading times, especially on slower connections.
Svelte’s Performance Optimization Techniques
Svelte offers a range of techniques to enhance the performance of your web applications. Let’s explore some of the most effective ones:
1. Efficient Reactivity
Svelte’s reactive system is designed to update the DOM only when necessary. Unlike traditional frameworks that may re-render the entire component hierarchy, Svelte intelligently updates only the parts of the DOM that have changed. This results in a more efficient rendering process and reduces the risk of performance bottlenecks caused by excessive re-renders.
svelte <script> let count = 0; function increment() { count += 1; } </script> <button on:click={increment}> Clicked {count} {count === 1 ? 'time' : 'times'} </button>
2. Lazy Loading
Svelte provides a built-in mechanism for lazy loading components. This means that components are loaded only when they are actually needed, reducing the initial bundle size and improving the app’s startup performance. Lazy loading is particularly useful for larger applications with complex component structures.
svelte <script> import { lazy } from 'svelte/lazy'; let showComponent = false; const LazyComponent = lazy(() => import('./LazyComponent.svelte')); </script> {#if showComponent} <LazyComponent /> {:else} <button on:click={() => showComponent = true}>Load Component</button> {/if}
3. Code Splitting
Svelte’s code splitting feature allows you to split your application into smaller chunks that can be loaded separately. This helps reduce the initial loading time by only fetching the necessary code when a specific route or feature is accessed. Code splitting can be achieved using dynamic imports.
svelte <script> import('./DynamicComponent.svelte').then(module => { const { default: DynamicComponent } = module; // Use DynamicComponent here }); </script>
4. Optimized CSS Output
Svelte optimizes your styles by eliminating unused CSS classes, resulting in a smaller overall bundle size. It achieves this by analyzing your component’s markup and generating only the necessary styles. This not only reduces the amount of CSS that needs to be loaded but also enhances the app’s runtime performance.
svelte <style> .container { padding: 16px; background-color: #f2f2f2; } </style> <div class="container"> <!-- Content here --> </div>
5. Bundling and Minification
Svelte’s built-in bundler automatically optimizes your code by removing whitespace, comments, and unused code. This results in a smaller bundle size, which leads to faster loading times. Additionally, you can enable minification to further reduce the bundle size by shortening variable and function names.
6. Server-Side Rendering (SSR)
Svelte supports server-side rendering, allowing you to pre-render your app on the server before sending it to the client. SSR improves initial loading times and enhances SEO by providing search engines with fully-rendered content. SvelteKit, a framework built on top of Svelte, simplifies the process of implementing SSR.
Conclusion
In the competitive world of web development, optimizing performance is no longer a luxury but a necessity. Svelte equips developers with powerful tools and techniques to create lightning-fast web apps. By leveraging efficient reactivity, lazy loading, code splitting, optimized CSS output, and other features, you can ensure that your applications deliver exceptional user experiences. Whether you’re building a simple portfolio site or a complex web application, Svelte’s performance optimization techniques are your gateway to a faster and more responsive digital future.
Table of Contents
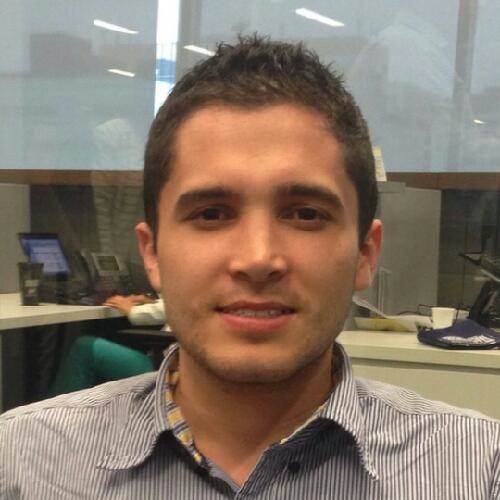
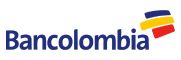