Advanced Svelte Techniques: Building Reactive Web Apps with Superior Efficiency
Svelte is rapidly emerging as a powerful contender in the realm of modern front-end development. This innovative JavaScript framework shatters traditional paradigms, making it a compelling choice when you’re looking to hire Svelte developers. Offering a fresh, engaging, and efficient way to build web applications, Svelte stands out from other frameworks.
Unlike React or Vue, which rely on a virtual DOM, Svelte compiles your code to highly efficient imperative code that directly manipulates the DOM. This unique approach results in faster performance and less code, an advantage that Svelte developers can leverage to build high-performance applications.
If you’re already familiar with the basics of Svelte, or if you plan to hire Svelte developers to boost your project, this blog post will help you master its advanced concepts and unlock its full potential. Understanding these principles can significantly streamline your hiring process and ensure that the Svelte developers you hire can effectively exploit the framework’s strengths.
1. Reactive Declarations
One of Svelte superpowers is its approach to reactivity. Svelte implements reactivity at the language level. It’s not a library that you import, but an integral part of Svelte syntax.
A simple example of a reactive statement looks like this:
```svelte <script> let count = 0; // Reactive statement $: doubled = count * 2; </script> ```
The `$:` is Svelte syntax for reactivity. The variable `doubled` will automatically update whenever `count` changes.
2. Computed Properties
Computed properties are a way to create variables that depend on other variables and automatically update when those variables change. Here’s how you would create a computed property in Svelte:
```svelte <script> let firstName = 'John'; let lastName = 'Doe'; // Computed property $: fullName = `${firstName} ${lastName}`; </script> ```
The `fullName` will now always be a combination of `firstName` and `lastName`, and any changes to these variables will automatically cause `fullName` to update.
3. Context API
In Svelte, parent components can communicate with child components through props. However, for components that are deeply nested, passing down props can become tedious. Svelte solves this with the Context API, providing a simple way to share values between components.
Here’s how you can use it:
```svelte <script> import { setContext } from 'svelte'; let user = { name: 'John Doe', age: 25 }; setContext('user', user); </script> ```
In a child component, you can retrieve the value with `getContext`:
```svelte <script> import { getContext } from 'svelte'; let user = getContext('user'); </script> ```
4. Slots and Slot Props
Slots are a way to create reusable components that can accept custom content. This is similar to React’s children props or Vue’s slots. Here’s an example:
```svelte <!-- Message.svelte --> <div class="message"> <slot></slot> </div> ```
You can then use this component like this:
```svelte <script> import Message from './Message.svelte'; </script> <Message> This is a custom message! </Message> ```
In addition to this, Svelte also provides slot props. They allow the parent component to pass values to the slotted content:
```svelte <!-- Message.svelte --> <div class="message"> <slot name="header"></slot> <slot></slot> </div> ```
And use it like this:
```svelte <Message> <h1 slot="header">Hello, world!</h1> <p>This is a custom message!</p> </Message> ```
5. Lifecycle Hooks
Svelte provides a handful of lifecycle hooks that you can use to run code at specific times in a component’s lifecycle. The most commonly used are `onMount`, `beforeUpdate`, and `afterUpdate`.
Here’s a quick example of how you can use them:
```svelte <script> import { onMount, beforeUpdate, afterUpdate } from 'svelte'; let count = 0; onMount(() => { console.log('Component has mounted'); }); beforeUpdate(() => { console.log('Count is about to update'); }); afterUpdate(() => { console.log('Count has updated'); }); </script> ```
6. Animation and Transition
One of Svelte strengths is its first-class support for animations and transitions. With Svelte, you can easily animate elements entering, updating, or leaving the DOM.
Here’s an example of how you can animate an element fading in:
```svelte <script> import { fade } from 'svelte/transition'; </script> <div in:fade> Hello, world! </div> ```
With just a few lines of code, Svelte provides a smooth fade-in animation for your element.
7. Store
Svelte provides a simple state management solution in the form of Stores. A Svelte store is an object with a `subscribe` method that allows reactive components to respond as the store’s data changes.
Here’s how you can create and use a Svelte store:
```svelte <script> import { writable } from 'svelte/store'; const count = writable(0); // You can subscribe to changes count.subscribe(value => { console.log(value); }); // You can also use it reactively in components $: console.log($count); </script> ```
8. Svelte Actions
Svelte actions are functions that can be run when an element is added to the DOM. You can use actions to add behavior to DOM elements, like tooltips, intersection observers, or any other DOM-related tasks.
Here’s a simple action that logs the width of an element when it’s added to the DOM:
```svelte <script> function logWidth(node) { console.log(node.offsetWidth); return { destroy() { // cleanup code here } }; } </script> <div use:logWidth> Hello, world! </div> ```
Conclusion
Mastering these advanced concepts in Svelte can significantly bolster your ability to develop highly efficient, reactive web applications. Whether you’re looking to refine your personal skills or hire Svelte developers for a team, understanding the power of this tool is crucial. The Svelte unique compile-step advantage, simplified syntax, and reduced boilerplate code position it as a compelling choice in the modern JavaScript frameworks ecosystem.
Its seamless integration of imperative and declarative programming gives developers, including those Svelte developers you might be looking to hire, the flexibility to construct complex UIs with unparalleled ease and elegance. By fully unleashing Svelte potential, you can elevate your web development capabilities, whether it’s for personal projects or guiding your hired Svelte developers. This leads to the creation of truly reactive web apps. Happy Svelting!
Table of Contents
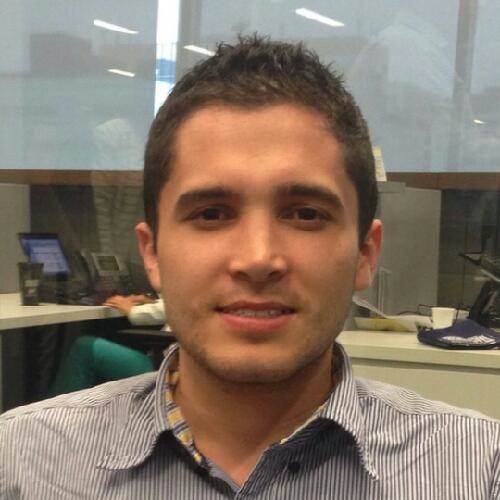
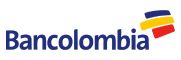