Svelte and Progressive Web Apps (PWAs): Building Offline-capable Web Applications
In today’s digital age, web applications have become an integral part of our daily lives. However, one of the challenges they face is maintaining functionality when the internet connection becomes unstable or unavailable. Progressive Web Apps (PWAs) have emerged as a solution to this problem, allowing web applications to work seamlessly offline. In this article, we will explore how to combine the power of Svelte, a modern front-end framework, with PWAs to build offline-capable web applications that provide a reliable and engaging user experience.
1. Understanding Progressive Web Apps (PWAs):
1.1 What Are PWAs?
Progressive Web Apps (PWAs) are web applications that leverage modern web technologies to provide a native-app-like experience to users, both online and offline. PWAs aim to bridge the gap between traditional web apps and native mobile apps by offering features like offline access, fast loading, push notifications, and more. These apps are designed to work across different devices and platforms, making them versatile and user-friendly.
1.2 Key Features of PWAs:
- Offline Capability: PWAs can function without an active internet connection, allowing users to access content even in low or no connectivity scenarios.
- Responsive Design: PWAs are built with responsive design principles, ensuring that the app looks and works seamlessly across various screen sizes and orientations.
- App-Like Experience: PWAs offer a user experience similar to native mobile apps, with smooth animations, gestures, and an immersive full-screen mode.
- Fast Loading: PWAs are optimized for quick loading, reducing the time users have to wait before they can start using the app.
- Push Notifications: Just like native apps, PWAs can send push notifications to users, keeping them engaged and informed even when the app is not open.
2. Introducing Svelte:
2.1 Why Choose Svelte?
Svelte is a modern JavaScript framework that stands out for its innovative approach to building web applications. Unlike traditional frameworks that perform much of their work in the browser, Svelte shifts the heavy lifting to build-time, resulting in highly optimized and efficient code. This approach leads to faster runtime performance and smaller bundle sizes, which are crucial for delivering a smooth user experience, especially in low connectivity situations.
2.2 Getting Started with Svelte:
To get started with Svelte, make sure you have Node.js and npm (Node Package Manager) installed. If not, you can download them from the official Node.js website.
Installing Svelte:
Open your terminal and run the following command to install the Svelte project template:
bash npx degit sveltejs/template svelte-pwa-app
Navigating to the Project:
Navigate to the project directory:
bash cd svelte-pwa-app
Installing Dependencies:
Install the project’s dependencies:
bash npm install
Starting the Development Server:
Start the development server:
bash npm run dev
3. Building an Offline-Capable Svelte PWA:
3.1 Setting Up the Service Worker:
A Service Worker is a JavaScript file that runs in the background, separate from the web page, and provides key PWA functionalities. To enable offline access, we need to set up a Service Worker in our Svelte project.
Create a new file named service-worker.js in the public directory. Add the following code:
javascript // service-worker.js const CACHE_NAME = "offline-cache"; const urlsToCache = ["/", "/index.html", "/global.css", /* add other assets here */]; self.addEventListener("install", (event) => { event.waitUntil( caches.open(CACHE_NAME).then((cache) => { return cache.addAll(urlsToCache); }) ); }); self.addEventListener("fetch", (event) => { event.respondWith( caches.match(event.request).then((response) => { return response || fetch(event.request); }) ); });
In the above code, the install event is used to cache the specified assets when the Service Worker is installed, and the fetch event intercepts network requests and serves cached responses if available.
3.2 Registering the Service Worker:
To register the Service Worker, open the src/main.js file and add the following code at the bottom:
javascript // src/main.js if ("serviceWorker" in navigator) { navigator.serviceWorker.register("/service-worker.js") .then((registration) => { console.log("Service Worker registered with scope:", registration.scope); }) .catch((error) => { console.error("Service Worker registration failed:", error); }); }
3.3 Making the App Installable:
PWAs can be installed on a user’s device, providing quick access and allowing users to interact with the app like a native app. To make the Svelte app installable, we need to add a Web App Manifest.
Create a file named manifest.json in the public directory and add the following code:
json { "name": "Svelte PWA App", "short_name": "Svelte PWA", "start_url": "/", "display": "standalone", "background_color": "#ffffff", "theme_color": "#ff3e00", "icons": [ { "src": "/icon.png", "sizes": "192x192", "type": "image/png" } ] }
In the above code, you can customize the app’s name, short name, colors, and icon. The display property set to “standalone” indicates that the app should open in a standalone mode, resembling a native app.
3.4 Optimizing for Offline Usage:
To enhance the offline experience, consider optimizing your Svelte components to handle scenarios where the user might not have an internet connection. Here are a few strategies:
- Cached Data: Store essential data in the cache during the Service Worker’s installation phase. This way, even if the user is offline, they can access some content.
- Offline Page: Create a special offline page that users are redirected to when they try to access the app without an internet connection. This page can provide basic information and suggest actions users can take once they are back online.
- Caching Strategies: Implement caching strategies to ensure that when the user is online, the app fetches and caches new data, so it’s available offline.
Conclusion
Building offline-capable web applications is a significant step towards improving user engagement and satisfaction. By harnessing the power of Svelte and Progressive Web App technologies, developers can create applications that seamlessly adapt to varying network conditions and provide a reliable experience. With Svelte’s efficient code generation and the capabilities offered by PWAs, you can create performant and user-friendly apps that are not hindered by unreliable internet connections. Embrace the future of web development by mastering these technologies and crafting compelling offline experiences for your users.
Table of Contents
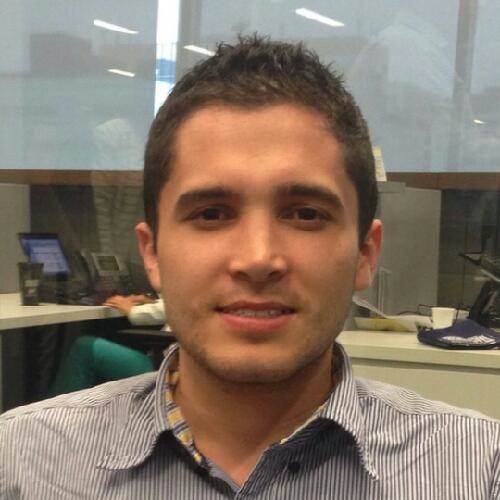
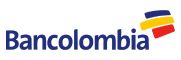