Transforming Web Development with Svelte Reactive HTML Templates
Web development continues to evolve at an astonishing pace, with new languages, frameworks, and libraries constantly reshaping the landscape. One such evolution is Svelte, a relatively new JavaScript framework that’s been gaining momentum among developers and prompting an increasing number of businesses to hire Svelte developers. Touted as a radical new approach to building user interfaces, Svelte is fundamentally different from frameworks like React or Vue because it compiles your code to tiny, framework-less vanilla JavaScript, resulting in code that’s faster and leaner.
Svelte stands out for its intuitive and innovative approach to reactive programming. With its templates, it allows developers to write HTML, CSS, and JavaScript in a concise and clean manner. As companies hire Svelte developers, they are unlocking the potential of this powerful framework in their projects. This blog post will dive deep into the world of Svelte templates and demonstrate how they’re simplifying web development with reactive HTML.
What are Svelte Templates?
At their core, Svelte templates are where you structure your HTML. Like any other template in traditional web development, Svelte templates are the foundation of your user interface. The difference is that they’re reactive: any changes in your state data are automatically reflected in the DOM.
This reactivity is what makes Svelte templates special. With Svelte, you can define reactive statements that update the HTML when state variables change, eliminating the need to manually update the DOM or use a virtual DOM, as is the case with other frameworks.
Let’s delve into the magic of Svelte templates with some examples.
Example 1: The Basics
Let’s start with a simple example. Here’s a Svelte component that declares a variable and then uses it in the HTML:
```svelte <script> let name = 'Svelte'; </script> <main> <h1>Hello {name}!</h1> </main> ```
In the script tag, we define a variable called `name` and assign it a value. Then in the HTML, we can directly refer to this variable by using curly braces `{}`. When this code is compiled, Svelte creates an HTML document that says “Hello Svelte!”.
This example also demonstrates a fundamental aspect of Svelte: components are self-contained units with their scripts and styles. In other words, both the JavaScript and the CSS for a component reside inside the component’s .svelte file.
Example 2: Reactivity
Now, let’s make things a bit more interesting. Let’s update the `name` variable and see what happens:
```svelte <script> let name = 'Svelte'; setTimeout(() => { name = 'Reactive'; }, 2000); </script> <main> <h1>Hello {name}!</h1> </main> ```
In this example, we’re changing the `name` variable after 2 seconds. Because Svelte templates are reactive, the HTML will automatically update when `name` changes. So after 2 seconds, the output will change from “Hello Svelte!” to “Hello Reactive!”.
Example 3: Conditional Rendering
Svelte templates also support conditional rendering using `{#if}{/if}` blocks. Here’s an example:
```svelte <script> let visible = true; </script> {#if visible} <p>The paragraph is visible.</p> {/if} <button on:click={() => visible = !visible}>Toggle visibility</button> ```
In this example, the paragraph is only visible if the `visible` variable is true. Clicking the button toggles the `visible` variable, which in turn toggles the paragraph’s visibility.
Example 4: Loops
Looping through arrays and objects is straightforward with Svelte. You can use `{#each}{/each}` blocks to render lists of data:
```svelte <script> let items = ['Apple', 'Banana', 'Cherry']; </script> <ul> {#each items as item} <li>{item}</li> {/each} </ul> ```
In this example, we’re looping over an array of items and creating a new `<li>` for each item.
Example 5: Component Composition
Svelte makes it easy to compose complex interfaces by nesting components. Let’s say we have a `Button.svelte` component like this:
```svelte <script> export let text; </script> <button>{text}</button> ```
We can use this `Button` component in another component like so:
```svelte <script> import Button from './Button.svelte'; </script> <main> <Button text="Click me" /> </main> ```
In this example, the `Button` component accepts a `text` prop, which is then used as the button text.
Conclusion
As we’ve seen in these examples, Svelte templates offer a powerful and intuitive approach to reactive web development. By mixing HTML with reactive statements, you can write less code and achieve more, all while maintaining a clear separation of concerns.
By compiling your code to vanilla JavaScript, Svelte delivers unparalleled performance benefits. This, coupled with its innovative take on reactivity, makes Svelte a powerful tool for modern web development and a compelling reason for companies to hire Svelte developers.
If you’re a web developer who hasn’t yet given Svelte a try, I hope this post has piqued your interest. Alternatively, if you’re a business looking to streamline your web development processes, consider the benefits that come when you hire Svelte developers. You’ll find that Svelte reactive templates can dramatically simplify your codebase and enhance productivity. In the world of Svelte, web development is indeed svelte (pun intended)!
Table of Contents
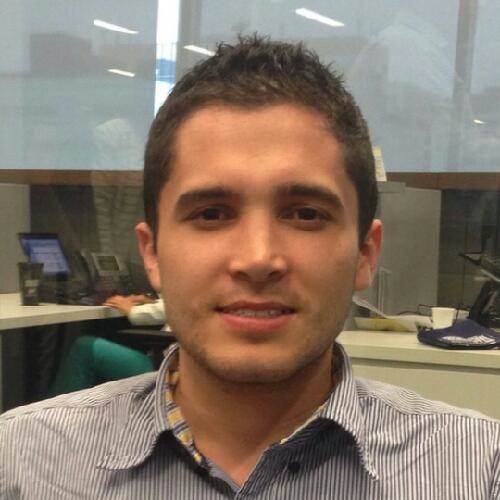
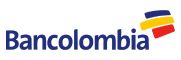