The Definitive Guide to Routing in Svelte Single-Page Applications
Single-Page Applications (SPAs) have emerged as a modern solution for building user-friendly, efficient web applications. Unlike traditional web applications that require a full-page reload when switching between pages, SPAs dynamically rewrite the current page, providing a more seamless and intuitive user experience.
Svelte, an innovative JavaScript framework for building user interfaces, has gained popularity for its simplicity, performance, and ease of understanding. This is why many businesses hire Svelte developers to build engaging and efficient applications. However, one feature Svelte doesn’t provide out of the box is routing.
So, how do we navigate between pages in a Svelte SPA? This post will delve into Svelte routing, with practical examples to guide you through building seamless navigation. If the process seems complex, remember that you can always hire Svelte developers who have the expertise to set up efficient navigation in your SPA.
What is Routing?
In web development, routing is the process of defining how an application responds to a client request for a specific endpoint. In the context of SPAs, routing refers to the ability to load and display different components or views when a user navigates to different URLs within the app. Routing is fundamental for any SPA, as it enables navigation between different parts of the application without the need to reload the entire page.
Svelte Routing with `svelte-routing`
Svelte doesn’t natively support routing, but there are several excellent third-party libraries available. One such library is `svelte-routing`, which provides a set of components to manage navigation and render components based on the current URL.
Let’s walk through a basic example of setting up routing with `svelte-routing`.
Step 1: Installing `svelte-routing`
First, install `svelte-routing` using npm:
```bash npm install svelte-routing ```
Step 2: Setting Up Routes
After installation, set up your routes using the `Route` and `Router` components from `svelte-routing`. Here’s an example with three routes: Home, About, and Contact.
```jsx <script> import { Router, Route } from 'svelte-routing'; import Home from './routes/Home.svelte'; import About from './routes/About.svelte'; import Contact from './routes/Contact.svelte'; </script> <Router> <Route path="/" component={Home} /> <Route path="about" component={About} /> <Route path="contact" component={Contact} /> </Router> ```
Each `Route` component has a `path` prop, which matches the URL path, and a `component` prop, which takes the component to render for that path. The `Router` component wraps around all `Route` components and manages the overall routing logic.
Step 3: Navigation
For navigation between pages, `svelte-routing` provides a `Link` component. Here’s how you can create navigation links:
```jsx <script> import { Link } from 'svelte-routing'; </script> <nav> <Link to="/">Home</Link> <Link to="/about">About</Link> <Link to="/contact">Contact</Link> </nav> ```
Clicking a `Link` will update the URL, and the corresponding component will be rendered.
More Complex Routing: Nested and Parameterized Routes
For more complex applications, you may need nested routes (routes within other routes) or parameterized routes (routes that contain dynamic parts).
Nested Routes
Nested routes are useful when you want to create a hierarchical URL structure.
Suppose you have a “User” page, and under that, you want individual pages for each user. Here’s how you can do it:
```jsx <script> import { Router, Route, Link } from 'svelte-routing'; import User from './routes/User.svelte'; import UserProfile from './routes/UserProfile.svelte'; </script> <Router> <Route path="user" let:params component={User}> <Route path="profile/:id" component={UserProfile} /> </Route> </Router> <nav> <Link to="/user">User</Link> </nav> ```
In this example, the `UserProfile` route is nested inside the `User` route, creating a URL structure like `/user/profile/:id`.
Parameterized Routes
Parameterized routes are routes that contain a dynamic part, typically an ID. In the previous example, `:id` is a route parameter.
In your `UserProfile` component, you can access this `id` using the `params` prop provided by the `Route` component:
```jsx <script> import { Route } from 'svelte-routing'; </script> <Route let:params> <p>User ID: {params.id}</p> </Route> ```
This code will display the `id` from the URL, so if you navigate to `/user/profile/123`, it will display “User ID: 123”.
Programmatic Navigation
In some cases, you might need to navigate programmatically, such as after a user submits a form. `svelte-routing` provides a `navigate` function for this purpose:
```jsx <script> import { navigate } from 'svelte-routing'; function handleSubmit() { // Form handling code... // After form submission, navigate to home navigate('/'); } </script> ```
In this example, after form submission, the `handleSubmit` function navigates the user back to the Home page.
Conclusion
While Svelte does not natively support routing, libraries such as `svelte-routing` fill the gap effectively, providing both basic and complex routing capabilities. These libraries are among the many tools Svelte developers use to enhance the functionality of your application. With the concepts and examples discussed in this blog post, you should now be able to set up routing in your Svelte applications with ease. If these tasks seem daunting, consider hiring Svelte developers who have the expertise to handle such complexities. Remember, routing is a fundamental part of any SPA, enabling Svelte developers to create more interactive and user-friendly web applications. Happy coding!
Table of Contents
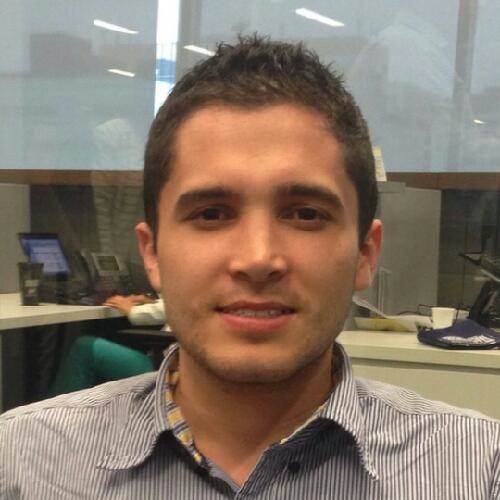
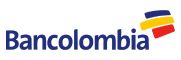