Svelte and Server-Side Rendering: Enhancing Performance and SEO
In the fast-paced world of web development, performance and search engine optimization (SEO) are crucial factors that can make or break the success of your web application. Users demand lightning-fast loading times, while search engines rely on well-structured content for effective indexing. This is where a combination of Svelte and Server-Side Rendering (SSR) comes into play, offering a powerful solution to meet both of these demands.
1. Understanding the Power of Svelte
Svelte, often hailed as a radical new approach to building web applications, is a component-based JavaScript framework that compiles components into highly optimized vanilla JavaScript code during build time. This unique compilation process shifts much of the heavy lifting from the browser to the build process itself, resulting in leaner, faster, and more efficient applications.
2. The Virtual DOM Difference
Unlike traditional frontend frameworks that rely on a virtual DOM (Document Object Model), Svelte doesn’t create a virtual representation of the DOM to track changes and updates. Instead, Svelte compiles components into imperative code that directly manipulates the DOM. This means that there’s no runtime overhead for managing a virtual DOM, resulting in a significant reduction in memory usage and faster runtime performance.
3. Code Sample: A Basic Svelte Component
svelte <script> let count = 0; function increment() { count += 1; } </script> <button on:click={increment}> Clicked {count} {count === 1 ? 'time' : 'times'} </button>
4. Unveiling the Benefits of Server-Side Rendering (SSR)
Server-Side Rendering (SSR) is a technique that renders web pages on the server and sends a fully populated HTML page to the client, as opposed to rendering components on the client side using JavaScript. This approach has several advantages, particularly in terms of performance and SEO.
5. Improved Initial Page Load Time
When a user visits a website, traditional client-side rendering frameworks load the necessary JavaScript bundles and then render the components on the client’s browser. This process can lead to longer initial page load times, especially if the user has a slower internet connection or less powerful device. With SSR, the server generates a complete HTML page with pre-rendered content, reducing the time users spend waiting for content to appear.
6. Enhanced SEO and Indexing
Search engines rely on the HTML content of a web page to index and rank it accurately. With client-side rendering, search engine bots may struggle to index the content efficiently because they often load pages before JavaScript has fully executed, resulting in incomplete or missing content. SSR ensures that search engine bots receive a fully populated HTML page, improving the chances of better indexing and ranking in search results.
7. Code Sample: Implementing SSR with SvelteKit
SvelteKit, the official routing and rendering framework for Svelte, makes it relatively straightforward to implement Server-Side Rendering.
7.1 Install SvelteKit:
bash npx degit sveltejs/kit my-svelte-app cd my-svelte-app npm install
7.2 Create a Svelte Page Component:
Create a new Svelte component for the page you want to render on the server. For example, create a file named src/routes/index.svelte.
7.3 Export Load Function:
In your page component, export a load function that fetches the necessary data for the page.
svelte <script context="module"> export async function load({ fetch }) { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return { props: { data } }; } </script>
7.4 Use Fetched Data in Component:
You can access the fetched data in your page component and render it accordingly.
svelte <script> export let data; </script> <main> {#each data as item} <article>{item.title}</article> {/each} </main>
7.5 Build and Start the Server:
Build your SvelteKit app and start the server to see SSR in action.
arduino npm run build npm run start
8. Achieving the Perfect Symbiosis
By combining the power of Svelte with Server-Side Rendering, you can create web applications that offer exceptional performance and SEO benefits. Here’s a recap of the advantages this combination provides:
- Faster Initial Load Times: SSR reduces the time users spend waiting for content to appear, resulting in a more seamless and responsive user experience.
- Improved SEO: Search engine bots can easily index the fully populated HTML pages generated by SSR, leading to better search engine rankings.
- Lower Time-to-Interactivity: Pre-rendered content means users can interact with your application sooner, even before JavaScript bundles are fully loaded and executed.
- Optimized Performance: Svelte’s efficient compilation process combined with SSR’s reduced client-side rendering overhead results in highly performant web applications.
- Progressive Enhancement: SSR serves a functional HTML page to all users, regardless of whether they have JavaScript enabled or not.
Conclusion
In today’s competitive web development landscape, it’s crucial to prioritize performance and SEO to deliver the best possible user experience and reach a wider audience. Svelte’s unique compilation approach and the implementation of Server-Side Rendering through SvelteKit provide an effective solution for achieving these goals. By leveraging these technologies, you can build web applications that load faster, rank better in search engines, and provide a seamless experience for users across the board. So why wait? Dive into Svelte and SSR and take your web development skills to the next level.
Remember, the journey to mastering Svelte and SSR may have its challenges, but the rewards in terms of performance and SEO are well worth the effort. Happy coding!
Table of Contents
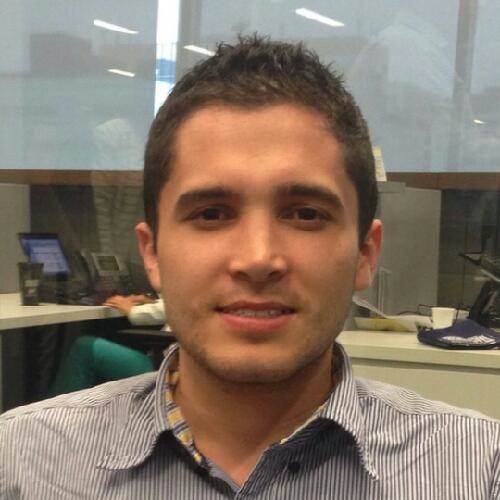
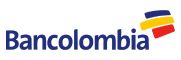