Svelte: Shaping the Web Development Landscape with its Revolutionary Techniques
In the world of web development, there is a constant stream of new technologies aiming to simplify the process of creating complex web applications. Amidst a pantheon of web development tools, Svelte has rapidly emerged as a standout tool, redefining the paradigm of reactive programming with a revolutionary approach. As more businesses recognize its potential, the demand to hire Svelte developers has significantly increased. This blog post will dive into the power of Svelte, backed by practical examples, demonstrating why it might be beneficial for your organization to consider hiring Svelte developers. Unearth Svelte unique attributes that are changing the game of web development and understand why it’s a favored choice amongst modern web developers.
What is Svelte?
In the simplest terms, Svelte is a component-based JavaScript framework developed for building user interfaces. Like React and Vue, it enables developers to construct complex UIs from simple building blocks. However, what sets Svelte apart is its compiler-based approach.
Unlike traditional frameworks that do the bulk of their work in the browser, Svelte shifts that work into a compile step that happens when you build your app, converting your components into highly efficient imperative code that updates the DOM surgically. This leads to faster initial load, smoother updates, and less overall code.
The Magic of Svelte: An Example
To better understand Svelte power, let’s build a simple interactive counter.
```svelte <script> let count = 0; function handleClick() { count += 1; } </script> <button on:click={handleClick}> Clicked {count} {count === 1 ? 'time' : 'times'} </button> ```
This simple Svelte component renders a button that, when clicked, increments a counter. The `{count}` syntax in the button’s text is a simple data binding that will automatically update the button’s text whenever the value of `count` changes.
The `on:click` attribute in the button tag is how we listen for click events. Whenever the button is clicked, it calls the `handleClick` function, which increments the count.
With just a few lines of code, we have created an interactive UI element. All the state management and event handling are done without having to touch the DOM directly or use extra libraries. Svelte handles it all under the hood.
A Deeper Dive: Reusable Components and Props
Svelte components are reusable and can accept props, just like React components. Let’s expand our previous example by creating a reusable `Counter` component:
```svelte <script> export let initialCount = 0; let count = initialCount; function handleClick() { count += 1; } </script> <button on:click={handleClick}> Clicked {count} {count === 1 ? 'time' : 'times'} </button> ```
Now, this `Counter` component can be imported and used with different initial counts:
```svelte <script> import Counter from './Counter.svelte'; </script> <Counter initialCount={3} /> <Counter initialCount={10} /> ```
In the `Counter` component, we’re using the `export` keyword to declare a prop that we can pass in from the parent component. This allows us to customize each instance of the `Counter` component with a different initial count.
Svelte Stores: State Management Simplified
State management is often one of the most complex aspects of developing web applications. Svelte simplifies state management with a concept called “stores”. A store in Svelte is essentially a single source of truth, a place where you can store state that needs to be shared across multiple components.
To illustrate this, let’s create a simple store that holds a count, and a couple of components that interact with this store:
```svelte <script> import { writable } from 'svelte/store'; // Create a writable store with an initial value of 0 export const count = writable(0); </script> ```
Now we can create a `Counter` component that increments the count in the store:
```svelte <script> import { count } from './store.js'; function handleClick() { $count += 1; } </script> <button on:click={handleClick}> Clicked {$count} {$count === 1 ? 'time' : 'times'} </button> ```
And a `DisplayCount` component that displays the current count:
```svelte <script> import { count } from './store.js'; </script> <p>The count is {$count}</p> ```
In both of these components, we’re using the `$count` syntax to access the current value of the `count` store. Anytime the `count` store changes, these components will automatically re-render with the new count.
The `handleClick` function in the `Counter` component uses this same `$count` syntax to update the count store when the button is clicked.
Svelte Revolutionary Approach: Benefits & Advantages
Let’s explore why Svelte approach of moving work from the browser to the compile step is revolutionary:
- Performance: Since Svelte applications are compiled ahead of time into tiny, surgical JavaScript operations, they can be highly optimized. This means faster initial load times and smoother updates compared to traditional frameworks that need to do a lot of work at runtime.
- Simplicity: Svelte aims to make the code more readable and less complex. There’s less boilerplate code, which results in cleaner codebases that are easier to maintain.
- Reactive declarations: Svelte has a unique approach to reactivity. You can create reactive statements using a simple syntax, and Svelte will automatically update the DOM whenever the underlying data changes.
- No virtual DOM: Unlike React and Vue, Svelte doesn’t use a virtual DOM. This results in a significant reduction in memory usage and faster updates.
- Smaller bundle sizes: Since most of the work is done at compile time, Svelte can eliminate unnecessary code, leading to smaller bundle sizes.
Conclusion
Svelte is not just another tool in the bloating JavaScript ecosystem. Instead, it represents a paradigm shift in how we think about and write web applications. Its innovative compile-time approach, coupled with its simplicity and high performance, makes it a promising framework for modern web development, thereby increasing the demand to hire Svelte developers.
If you’re a web developer who values efficiency and simplicity, Svelte might be just the tool you’ve been looking for. Alternatively, if you’re a business aiming to optimize your web applications, it might be time to consider hiring Svelte developers. Why not give it a try? Who knows, it might revolutionize your approach to building web apps, just as it’s revolutionizing the web development landscape.
Table of Contents
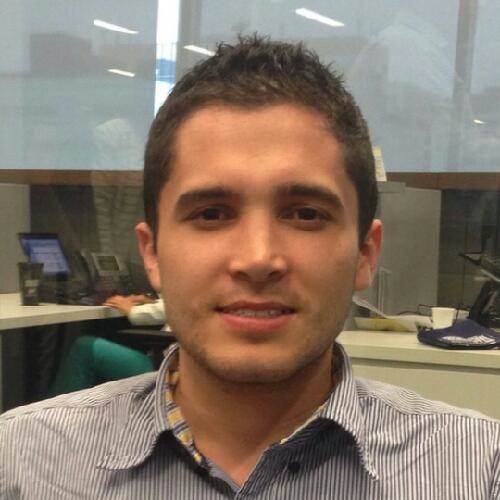
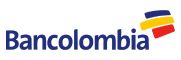