Creating Visually Captivating iOS Interfaces with Swift: An Advanced Guide
Every detail matters in crafting an exceptional user experience for an application, and that’s why User Interface (UI) customization is critical. With Apple’s Swift programming language, the ability to customize the UI has never been more accessible, prompting many to hire Swift developers for their projects. In this blog post, we delve into advanced UI customization in Swift and explore some techniques for creating unique iOS interfaces. With the expertise of Swift developers, you can effectively tackle custom views, animations, and touch gestures, among other elements, to add life and a distinctive aesthetic to your applications.
Table of Contents
1. Custom Views
Custom views are an integral part of UI customization in Swift. They allow you to design reusable components that can be used throughout the application.
Example: Custom Button Design
Let’s start with a simple but effective example – designing a custom button. We will create a rounded button with a gradient background.
```swift import UIKit class GradientButton: UIButton { override init(frame: CGRect) { super.init(frame: frame) setupButton() } required init?(coder: NSCoder) { super.init(coder: coder) setupButton() } private func setupButton() { layer.cornerRadius = frame.size.height/2 backgroundColor = UIColor.clear layer.masksToBounds = true } override func layoutSubviews() { super.layoutSubviews() applyGradient() } private func applyGradient() { let gradientLayer = CAGradientLayer() gradientLayer.colors = [UIColor.red.cgColor, UIColor.orange.cgColor] gradientLayer.startPoint = CGPoint(x: 0, y: 0) gradientLayer.endPoint = CGPoint(x: 1, y: 1) gradientLayer.frame = bounds layer.insertSublayer(gradientLayer, at: 0) } } ```
In this code snippet, we define a GradientButton class that extends UIButton. We override the layoutSubviews() function to apply a gradient to the button whenever it changes its layout.
2. Custom Transitions and Animations
Animations play a vital role in enhancing the user experience of an application. Swift provides several ways to create custom animations and transitions.
Example: Custom Transition Animation
Let’s create a simple transition animation where one view slides in from the right to replace the current view.
```swift import UIKit class SlideInTransition: NSObject, UIViewControllerAnimatedTransitioning { var isPresenting = false func transitionDuration(using transitionContext: UIViewControllerContextTransitioning?) -> TimeInterval { return 0.3 } func animateTransition(using transitionContext: UIViewControllerContextTransitioning) { let containerView = transitionContext.containerView let finalWidth = containerView.frame.width * 0.8 let finalHeight = containerView.frame.height if isPresenting { let toView = transitionContext.view(forKey: UITransitionContextViewKey.to)! containerView.addSubview(toView) toView.frame = CGRect(x: -finalWidth, y: 0, width: finalWidth, height: finalHeight) let transform = { toView.transform = CGAffineTransform(translationX: finalWidth, y: 0) } UIView.animate(withDuration: transitionDuration(using: transitionContext), animations: transform) { (_) in transitionContext.completeTransition(true) } } else { let fromView = transitionContext.view(forKey: UITransitionContextViewKey.from)! let transform = { fromView.transform = CGAffineTransform(translationX: -finalWidth, y: 0) } UIView.animate(withDuration: transitionDuration(using: transitionContext), animations: transform) { (_) in transitionContext.completeTransition(true) } } } } ```
In this code snippet, we define a SlideInTransition class that implements UIViewControllerAnimatedTransitioning protocol to create a custom transition animation.
3. Touch Gestures
Touch gestures can significantly enhance your application’s user interaction. Swift supports a variety of touch gestures, including tap, pinch, rotate, swipe, pan, and long press.
Example: Swipe Gesture Recognizer
Let’s add a swipe gesture to a view.
```swift import UIKit class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() let swipeRight = UISwipeGestureRecognizer(target: self, action: #selector(handleGesture)) swipeRight.direction = .right view.addGestureRecognizer(swipeRight) } @objc func handleGesture(gesture: UISwipeGestureRecognizer) -> Void { if gesture.direction == .right { print("Swipe Right") } } } ```
In this code, we add a right swipe gesture to the view. When a right swipe is detected, the handleGesture function is called.
Conclusion
These are just the tip of the iceberg in terms of UI customization capabilities in Swift. To fully leverage these capabilities, many companies opt to hire Swift developers, as they can dramatically increase your application’s usability and aesthetics with custom views, animations, and gestures. This leads to improved user satisfaction. Keep exploring the extensive Swift libraries and frameworks alongside your developers to discover and create more unique, intuitive, and visually appealing interfaces. Happy coding!
Table of Contents
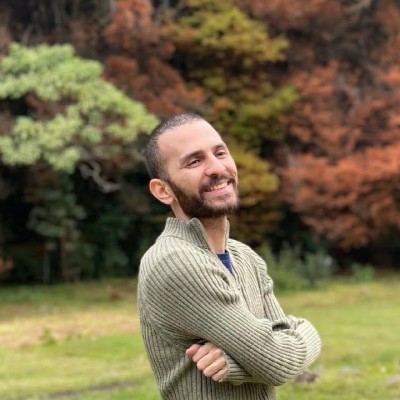
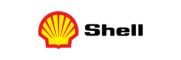