What is the difference between ‘Any’ and ‘AnyObject’ in Swift?
In Swift, ‘Any’ and ‘AnyObject’ are both used to work with values of unknown types, but they serve different purposes and have distinct characteristics.
- ‘Any’:
– ‘Any’ is a type in Swift that can represent an instance of any type, including both value types (like integers, strings, structs, enums) and reference types (like classes, closures, and functions).
– It is a flexible type that is often used when you need to work with values of various types within the same context, such as when dealing with heterogeneous collections.
– ‘Any’ allows you to store and manipulate values of different types without specifying the exact type at compile time. However, you lose type safety when working with ‘Any’ since the compiler can’t perform type checking and type inference for these values.
```swift var anyValue: Any anyValue = 42 // Integer anyValue = "Hello, Swift" // String anyValue = [1, 2, 3] // Array ```
- ‘AnyObject’:
– ‘AnyObject’ is also a type in Swift, but it is specific to reference types, primarily classes and class instances.
– It is often used when you need to work with objects of unknown class types, such as when dealing with instances of different classes in a collection or when using Objective-C APIs that return objects of various class types.
– ‘AnyObject’ provides a level of type safety compared to ‘Any’ because it is limited to reference types, and you can still perform type casting and type checking for these objects.
```swift var anyObjectValue: AnyObject anyObjectValue = UIView() // UIView instance anyObjectValue = UIButton() // UIButton instance ```
In summary, ‘Any’ is a more general type that can represent values of any type, while ‘AnyObject’ is specifically used for reference types and provides more type safety in scenarios involving class instances. Your choice between ‘Any’ and ‘AnyObject’ depends on the specific requirements of your code and whether you need to work with value types, reference types, or a combination of both.
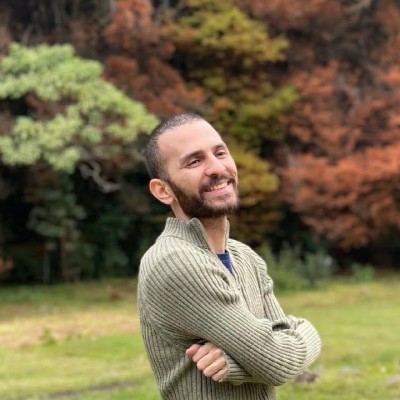
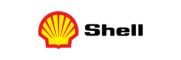