Swift and ARKit: Transform Your iOS Development Journey with Augmented Reality
With the advent of ARKit, Apple provided developers an incredibly powerful tool to create compelling augmented reality experiences for iOS devices. When combined with Swift, Apple’s easy-to-use programming language, developers can leverage the power of ARKit to quickly bring their ideas to life. If you’re looking to speed up the process, you might want to hire Swift developers, who can effectively harness these tools for rapid AR development. This blog post will delve into how we can build AR experiences using Swift and ARKit, with some practical examples, highlighting the efficiency and creativity Swift developers can bring to the table.
Table of Contents
1. Understanding ARKit
ARKit is an iOS framework that allows developers to create augmented reality (AR) experiences. It combines device motion tracking, camera scene capture, advanced scene processing, and display conveniences to simplify the task of building an AR experience. It can detect surfaces, estimate lighting conditions, and provide high-level understanding of the real-world environment, such as recognizing 3D objects or images.
2. Prerequisites
To follow along with the examples, you should have a basic understanding of Swift and iOS development. You’ll also need the following:
– An iOS device with an A9 processor or later, running iOS 11 or later
– Xcode 9 or later
– A basic understanding of SceneKit or SpriteKit (depending on your preference)
Example 1: Displaying a 3D Object in AR
Our first example will demonstrate how to display a 3D object using ARKit and SceneKit. We’ll place a 3D model of a chair in the real world.
Step 1: Set Up an ARKit Project
In Xcode, create a new project and select the “Augmented Reality App” template. Name the project and ensure that you’ve selected SceneKit as the content technology.
Step 2: Import the 3D Model
Import the 3D model (a .scn or .dae file) of the object you want to display. For this example, let’s use a chair model. You can add this model to the ‘art.scnassets’ folder in your project.
Step 3: Set Up the AR Scene
In the ViewController.swift file, import ARKit and set up the AR scene in the viewDidLoad function:
```swift import ARKit class ViewController: UIViewController { @IBOutlet var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() // Set the view's delegate sceneView.delegate = self // Show statistics such as fps and timing information sceneView.showsStatistics = true } } ```
Step 4: Add the 3D Object to the Scene
In the viewWillAppear function, create an ARWorldTrackingConfiguration and run the scene:
```swift override func viewWillAppear(_ animated: Bool) { super.viewWillAppear(animated) // Create a new scene let scene = SCNScene(named: "art.scnassets/chair.scn")! // Set the scene to the view sceneView.scene = scene // Create a session configuration let configuration = ARWorldTrackingConfiguration() // Run the view's session sceneView.session.run(configuration) } ```
Step 5: Handle Session Interruptions
AR sessions can be interrupted (e.g., by receiving a phone call), so it’s good to handle such situations. Add the following methods:
```swift func sessionWasInterrupted(_ session: ARSession) { // Inform the user that the session has been interrupted } func sessionInterruptionEnded(_ session: ARSession) { // Reset tracking and/or remove existing anchors if consistent tracking is required } ```
Now, run the app and point your device around to track the world, and you should see the 3D model of a chair appear in your AR scene.
Example 2: Plane Detection
ARKit can detect horizontal and vertical planes in the real world. Let’s add plane detection to our project.
Step 1: Update the Session Configuration
Modify the ARWorldTrackingConfiguration in the viewWillAppear function to enable plane detection:
```swift let configuration = ARWorldTrackingConfiguration() configuration.planeDetection = [.horizontal, .vertical] sceneView.session.run(configuration) ```
Step 2: Implement ARSCNViewDelegate Methods
Implement ARSCNViewDelegate methods to handle the addition, update, and removal of ARAnchors (detected planes in this case):
```swift func renderer(_ renderer: SCNSceneRenderer, didAdd node: SCNNode, for anchor: ARAnchor) { guard let planeAnchor = anchor as? ARPlaneAnchor else { return } let planeNode = createPlaneNode(planeAnchor) node.addChildNode(planeNode) } func renderer(_ renderer: SCNSceneRenderer, didUpdate node: SCNNode, for anchor: ARAnchor) { guard let planeAnchor = anchor as? ARPlaneAnchor, let planeNode = node.childNodes.first, let plane = planeNode.geometry as? SCNPlane else { return } updatePlaneNode(planeNode, with: planeAnchor) } func renderer(_ renderer: SCNSceneRenderer, didRemove node: SCNNode, for anchor: ARAnchor) { guard let _ = anchor as? ARPlaneAnchor else { return } node.childNodes.forEach { $0.removeFromParentNode() } } ```
You’ll need to create `createPlaneNode` and `updatePlaneNode` methods to create and update the plane nodes:
```swift func createPlaneNode(_ planeAnchor: ARPlaneAnchor) -> SCNNode { let plane = SCNPlane(width: CGFloat(planeAnchor.extent.x), height: CGFloat(planeAnchor.extent.z)) plane.materials.first?.diffuse.contents = UIColor.transparentLightBlue let planeNode = SCNNode(geometry: plane) planeNode.position = SCNVector3(planeAnchor.center.x, 0, planeAnchor.center.z) planeNode.transform = SCNMatrix4MakeRotation(-Float.pi / 2, 1, 0, 0) return planeNode } func updatePlaneNode(_ planeNode: SCNNode, with planeAnchor: ARPlaneAnchor) { planeNode.position = SCNVector3(planeAnchor.center.x, 0, planeAnchor.center.z) if let plane = planeNode.geometry as? SCNPlane { plane.width = CGFloat(planeAnchor.extent.x) plane.height = CGFloat(planeAnchor.extent.z) } } ```
Now, run the app again. As you move your device around, you should see planes being detected and visualized in the AR scene.
Example 3: Interaction with AR Objects
Finally, let’s make our AR objects interactive. We’ll make the chair from our first example respond to taps.
Step 1: Add Tap Gesture Recognizer
In the viewDidLoad function, add a UITapGestureRecognizer:
```swift let tapGestureRecognizer = UITapGestureRecognizer(target: self, action: #selector(handleTap(_:))) sceneView.addGestureRecognizer(tapGestureRecognizer) ```
Step 2: Implement the Tap Handler
Implement the handleTap function to handle the tap gesture:
```swift @objc func handleTap(_ gestureRecognize: UITapGestureRecognizer) { // Check if we've tapped on the plane let p = gestureRecognize.location(in: sceneView) let hitResults = sceneView.hitTest(p, options: nil) // Check that we've touched the chair node if hitResults.count > 0 { let result = hitResults[0 ] let node = result.node // If the chair node is touched, apply an upward force to it if node.name == "chair" { if let chairPhysicsBody = node.physicsBody { chairPhysicsBody.applyForce(SCNVector3(0, 9.8, 0), asImpulse: true) } } } } ```
Now, when you tap on the chair in the AR scene, it will react as if a force has been applied to it.
Conclusion
With these examples, you should now have a basic understanding of how to use Swift and ARKit to create AR experiences for iOS. ARKits ability to integrate digital information with the real world opens up a myriad of possibilities for app development. From gaming and entertainment to education and productivity, the potential applications of AR are vast. If you’re looking to expand your team’s expertise, you might consider the option to hire Swift developers, who can help accelerate your journey in this exciting field. By mastering Swift and ARKit, either on your own or with the help of experienced developers, you’re well on your way to being part of the thrilling world of augmented reality.
Table of Contents
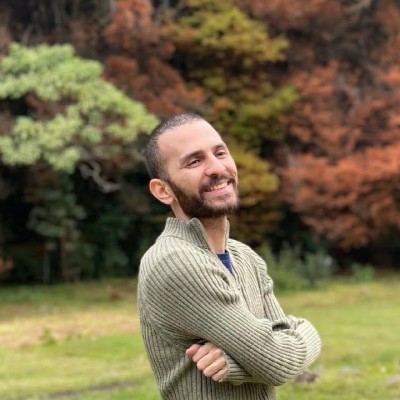
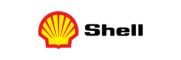