How do I create and use arrays in Swift?
An array in Swift is a collection of values that are stored in a specific order. You can create and manipulate arrays using the following steps:
Creating an Array:
To create an array, you declare it using the `var` keyword (if you want to modify it) or the `let` keyword (if it’s immutable or constant), followed by the array’s name, a colon, and the type of elements the array will contain enclosed in square brackets. Here’s an example of creating an array of integers:
```swift var numbers: [Int] = [1, 2, 3, 4, 5] ```
You can also create an array using type inference:
```swift var fruits = ["apple", "banana", "cherry"] ```
Accessing Elements:
You can access elements in an array by using square brackets with the index of the element you want to access. Swift arrays are zero-indexed, meaning the first element has an index of 0. For example:
```swift let firstFruit = fruits[0] // Accessing the first element ("apple") ```
Modifying Arrays:
To add an element to the end of an array, you can use the `append` method:
```swift fruits.append("orange") ```
To insert an element at a specific index, you can use the `insert` method:
```swift fruits.insert("grape", at: 2) // Inserts "grape" at index 2 ```
To remove an element, you can use the `remove` method:
```swift fruits.remove(at: 1) // Removes the element at index 1 ("banana") ```
Iterating through an Array:
You can use loops, like `for-in`, to iterate through the elements of an array:
```swift for fruit in fruits { print(fruit) } ```
Array Methods and Properties:
Swift provides numerous methods and properties for working with arrays, such as `count` to get the number of elements, `isEmpty` to check if the array is empty, and `sort` to sort the elements. These methods make it easy to manipulate arrays efficiently.
In summary, arrays are a fundamental data structure in Swift, used for storing collections of values. You can create, access, modify, and iterate through arrays using a variety of techniques and methods, making them a versatile tool for managing data in your Swift programs.
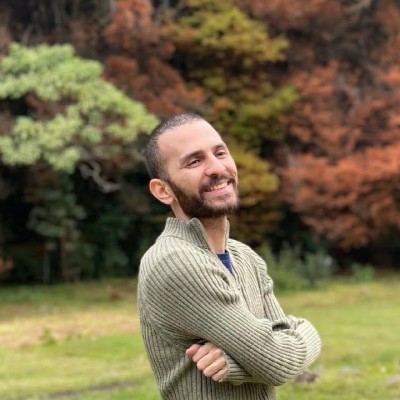
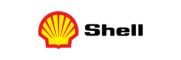