What is the ‘associatedtype’ keyword in Swift?
In Swift, the `associatedtype` keyword is a powerful feature used in protocols to define placeholder types that are associated with the protocol. These associated types allow you to write flexible and generic protocols that can be adopted by conforming types while specifying that certain types and their relationships should exist within those conforming types. Here’s a detailed explanation of the `associatedtype` keyword:
- Declaration of Associated Types: When you define a protocol that includes an associated type, you’re essentially saying that conforming types must provide a concrete type that will fulfill the role of that associated type. You declare an associated type using the `associatedtype` keyword within your protocol. For instance:
```swift protocol Container { associatedtype Item func addItem(_ item: Item) func getItem() -> Item? } ```
Here, the `Container` protocol has an associated type `Item` which represents the type of elements that the conforming type will hold.
- Type Abstraction: The `associatedtype` keyword abstracts the actual type, allowing conforming types to define the specific type they want to use. This flexibility is crucial because it lets you write generic protocols that can work with various types.
- Conforming to Protocols with Associated Types: When a type conforms to a protocol with an associated type, it must specify the actual type that will be used for the associated type. For example:
```swift struct MyArrayContainer<T>: Container { typealias Item = T private var items: [Item] = [] mutating func addItem(_ item: Item) { items.append(item) } func getItem() -> Item? { return items.first } } ```
In this example, `MyArrayContainer` conforms to the `Container` protocol and specifies that its associated type `Item` is of type `T`.
- Use Cases: `associatedtype` is commonly used when defining protocols for collections, data structures, or abstractions that can work with different types. It enables you to write highly reusable code by letting conforming types decide on the specific types they’ll use for the associated types.
In summary, the `associatedtype` keyword in Swift is a powerful tool for defining flexible, generic protocols. It allows you to create protocols that can work with various types while ensuring that conforming types provide the necessary associated types, making your code more adaptable and versatile.
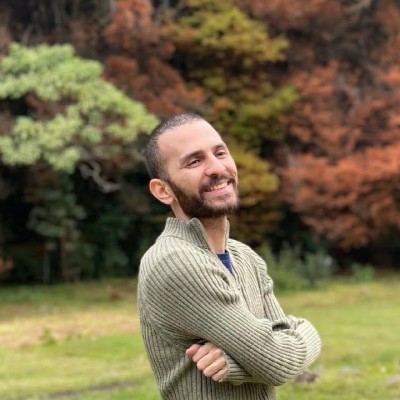
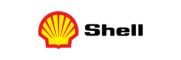