Swift Concurrency: Exploring the New Features for Asynchronous Programming
Asynchronous programming plays a vital role in modern software development, allowing us to build responsive and efficient applications. With the release of Swift 5.5, a game-changing feature was introduced: Swift Concurrency. This new set of language features provides developers with powerful tools to handle concurrency in a more structured and intuitive manner. In this blog post, we will explore the key features of Swift Concurrency, including tasks, actors, and structured concurrency, and understand how they can revolutionize your asynchronous code.
Understanding Swift Concurrency
What is Concurrency?
Before diving into Swift Concurrency, let’s first understand the concept of concurrency itself. Concurrency is the ability of a program to perform multiple tasks simultaneously, allowing for efficient resource utilization and improved responsiveness. Traditionally, concurrency in Swift was achieved using Grand Central Dispatch (GCD) or Operation Queues. However, these mechanisms often led to complex and error-prone code due to the lack of structured constructs.
Introducing Swift Concurrency
Swift Concurrency introduces a set of language features specifically designed to simplify asynchronous programming. These features allow developers to write more readable, maintainable, and safe concurrent code. Let’s explore some of the key features of Swift Concurrency:
Tasks: The foundation of Swift Concurrency is the “Task” abstraction. A task represents a unit of work that can be executed concurrently. It provides a structured and predictable way to perform asynchronous operations. Tasks can be created using the “async” keyword and are executed concurrently by the Swift runtime.
Asynchronous Functions
An asynchronous function is a function that can be suspended while waiting for a long-running operation to complete. These functions are marked with the “async” keyword and use the “await” keyword to pause the execution until a value is available. Here’s an example of an asynchronous function that fetches data from a network:
swift async func fetchData() -> Data { let url = URL(string: "https://api.example.com/data")! let (data, _) = try await URLSession.shared.data(from: url) return data }
In the above example, the function suspends its execution at the await statement until the data is fetched from the network. Once the data is available, it is returned to the caller.
Structured Concurrency
Swift Concurrency introduces the concept of structured concurrency, which helps manage the lifecycle of concurrent tasks. With structured concurrency, you can ensure that all child tasks complete before the parent task exits. This eliminates common pitfalls such as “zombie tasks” or tasks that continue executing even after their parent has completed. Structured concurrency is achieved using the TaskGroup construct. Here’s an example:
swift func performTasks() async { await withTaskGroup(of: Void.self) { group in group.async { // Task 1 } group.async { // Task 2 } // Wait for all tasks to complete await group.waitForAll() } }
In the above example, the withTaskGroup creates a task group, and the group.async calls execute tasks concurrently. The group.waitForAll() call ensures that all tasks have completed before the performTasks() function returns.
Actors
Swift Concurrency introduces a new abstraction called “actors” to safely manage shared mutable state in a concurrent environment. An actor encapsulates state and ensures that access to its properties is serialized, preventing data races. Actors are defined using the actor keyword and can be accessed concurrently only through their designated access points. Here’s an example:
swift actor Counter { private var value = 0 func increment() { value += 1 } func getValue() -> Int { return value } }
In the above example, the Counter actor encapsulates a mutable value property, and its methods ensure exclusive access to that property.
Conclusion
Swift Concurrency revolutionizes asynchronous programming in Swift, providing developers with powerful tools to write more readable, maintainable, and safe concurrent code. The introduction of tasks, actors, and structured concurrency simplifies the handling of asynchronous operations and mitigates common pitfalls. By adopting Swift Concurrency, you can build highly responsive and efficient applications while maintaining code clarity and safety.
This blog post only scratches the surface of Swift Concurrency, and there’s much more to explore. Swift Concurrency is a significant step forward for the Swift language and empowers developers to embrace concurrency with confidence. So, dive into the world of Swift Concurrency and unlock the full potential of asynchronous programming in your Swift projects!
Table of Contents
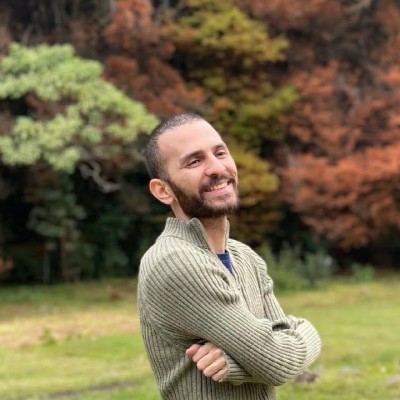
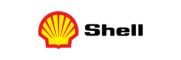