Swift and Augmented Reality: Creating AR Experiences for iOS
Augmented Reality (AR) is transforming the way users interact with the digital world by overlaying digital information onto the real world. With Swift and ARKit, Apple’s framework for AR development, creating immersive AR experiences for iOS devices has become more accessible and powerful. This blog explores how Swift can be utilized to build engaging AR applications and provides practical examples for integrating AR into your iOS projects.
Understanding Augmented Reality
Augmented Reality involves blending digital content with the real world in a way that appears natural to the user. AR applications can range from interactive games to practical tools like virtual furniture placement or real-time navigation.
Using Swift for AR Development
Swift, Apple’s powerful and intuitive programming language, pairs excellently with ARKit to bring AR experiences to life. Here are key aspects and code examples demonstrating how Swift can be used for AR development.
1. Setting Up an AR Project
To start developing AR applications, you need to set up an Xcode project with ARKit. Create a new project and select the “Augmented Reality App” template.
Example: Basic AR Setup in Swift
Here’s how to initialize a simple AR session using Swift.
```swift import UIKit import SceneKit import ARKit class ViewController: UIViewController, ARSCNViewDelegate { @IBOutlet var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() sceneView.delegate = self let configuration = ARWorldTrackingConfiguration() sceneView.session.run(configuration) } func renderer(_ renderer: SCNSceneRenderer, updateAtTime time: TimeInterval) { // Update logic here } } ```
2. Adding 3D Objects
You can add and manipulate 3D objects in AR using SceneKit. Swift and ARKit make it easy to position and interact with virtual objects in the real world.
Example: Adding a 3D Cube
Here’s how to add a simple 3D cube to the AR scene.
```swift import UIKit import SceneKit import ARKit class ViewController: UIViewController, ARSCNViewDelegate { @IBOutlet var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() sceneView.delegate = self let configuration = ARWorldTrackingConfiguration() sceneView.session.run(configuration) addCube() } func addCube() { let cube = SCNBox(width: 0.1, height: 0.1, length: 0.1, chamferRadius: 0) let material = SCNMaterial() material.diffuse.contents = UIColor.red cube.materials = [material] let cubeNode = SCNNode(geometry: cube) cubeNode.position = SCNVector3(0, 0, -0.5) sceneView.scene.rootNode.addChildNode(cubeNode) } } ```
3. Implementing AR Interactions
ARKit provides various ways to interact with AR content, such as tapping on objects or detecting gestures.
Example: Handling Tap Gestures
Here’s how you can detect taps and interact with AR objects.
```swift import UIKit import SceneKit import ARKit class ViewController: UIViewController, ARSCNViewDelegate { @IBOutlet var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() sceneView.delegate = self let configuration = ARWorldTrackingConfiguration() sceneView.session.run(configuration) let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap)) sceneView.addGestureRecognizer(tapGesture) } @objc func handleTap(sender: UITapGestureRecognizer) { let location = sender.location(in: sceneView) let hitTestResults = sceneView.hitTest(location, options: [:]) if let tappedNode = hitTestResults.first?.node { tappedNode.geometry?.firstMaterial?.diffuse.contents = UIColor.green } } } ```
4. Integrating with ARKit Features
ARKit includes advanced features like plane detection, face tracking, and environment mapping. These can be leveraged to create more sophisticated AR experiences.
Example: Plane Detection
Here’s how to detect horizontal planes and place objects on them.
```swift import UIKit import SceneKit import ARKit class ViewController: UIViewController, ARSCNViewDelegate { @IBOutlet var sceneView: ARSCNView! override func viewDidLoad() { super.viewDidLoad() sceneView.delegate = self let configuration = ARWorldTrackingConfiguration() configuration.planeDetection = .horizontal sceneView.session.run(configuration) } func renderer(_ renderer: SCNSceneRenderer, didAdd node: SCNNode, for anchor: ARAnchor) { if let planeAnchor = anchor as? ARPlaneAnchor { let plane = createPlane(from: planeAnchor) node.addChildNode(plane) } } func createPlane(from anchor: ARPlaneAnchor) -> SCNNode { let plane = SCNPlane(width: CGFloat(anchor.extent.x), height: CGFloat(anchor.extent.z)) let material = SCNMaterial() material.diffuse.contents = UIColor.transparentWhite plane.materials = [material] let planeNode = SCNNode(geometry: plane) planeNode.position = SCNVector3(anchor.center.x, 0, anchor.center.z) planeNode.eulerAngles.x = -.pi / 2 return planeNode } } ```
Conclusion
Swift, combined with ARKit, provides a robust framework for creating immersive augmented reality experiences on iOS. From setting up basic AR scenes to adding interactive elements and leveraging advanced ARKit features, Swift enables developers to build engaging AR applications with ease. By harnessing these capabilities, you can create compelling AR experiences that enhance user interaction and bring digital content to life.
Further Reading:
Table of Contents
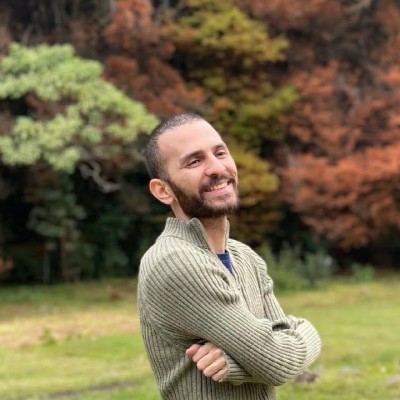
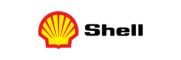