What is a class in Swift?
In Swift, a class is a fundamental building block of object-oriented programming (OOP) that defines a blueprint for creating objects. Classes are used to encapsulate data and behavior into a single unit, allowing you to create instances (objects) of that class. These instances can have their own properties (data) and methods (functions) associated with them. Classes serve as the foundation for creating complex, structured, and reusable code in Swift.
Here are key characteristics and concepts related to classes in Swift:
- Object-Oriented Programming: Swift is an object-oriented programming language, and classes are a core concept of OOP. They allow you to model real-world entities or abstract concepts in your code, making it more organized and maintainable.
- Properties: Classes can have properties, which are variables or constants that store data specific to an instance of the class. These properties define the attributes or characteristics of the object.
- Methods: Classes can also contain methods, which are functions associated with the class. Methods define the behaviors or actions that the objects of the class can perform.
- Initialization: Classes can have an initializer method, often called the constructor, which is used to set up the initial state of an object when it’s created.
- Inheritance: Swift supports class inheritance, allowing you to create new classes that inherit properties and methods from existing ones. This promotes code reuse and hierarchy in your codebase.
- Encapsulation: Classes provide encapsulation, which means they encapsulate data and behavior, and access to their properties and methods can be controlled through access control modifiers like `public`, `internal`, and `private`.
- Reference Types: Objects created from classes are reference types, meaning that when you assign an instance to another variable or pass it as an argument, you’re working with references to the same instance in memory.
Here’s a simplified example of a class in Swift:
```swift class Person { var name: String var age: Int init(name: String, age: Int) { self.name = name self.age = age } func sayHello() { print("Hello, my name is \(name) and I'm \(age) years old.") } } ```
In this example, we’ve defined a `Person` class with properties for name and age, an initializer, and a method to say hello.
In summary, classes in Swift are a foundational concept of object-oriented programming that allow you to model and encapsulate data and behavior into reusable and structured units. They are an essential part of building complex and maintainable applications in Swift.
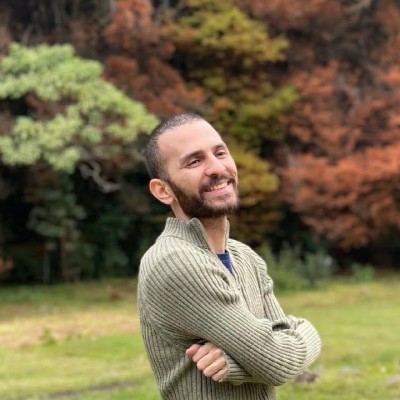
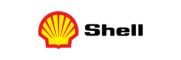