SwiftUI and Combine: Crafting Seamless and Reactive iOS User Experiences
The landscape of iOS development has evolved rapidly over the past few years, with SwiftUI and Combine leading the way into the realm of reactive programming. As businesses look to hire Swift developers, it becomes crucial to understand these new paradigms. Both frameworks introduce a fresh approach that places a strong emphasis on reactive, declarative code, offering developers an innovative way to conceptualize and manage UI and data.
Table of Contents
In this blog post, we will dive deep into the synergy of SwiftUI and Combine, illustrating how these two frameworks complement each other and reshape the traditional flow of iOS development for those looking to hire Swift developers or enhance their skills in the domain.
1. What is SwiftUI?
SwiftUI is Apple’s modern, declarative framework for building user interfaces across all Apple platforms. Instead of imperatively constructing UI elements as with UIKit, with SwiftUI, you declare the UI’s state and the framework automatically derives the necessary interface.
Example: SwiftUI Text View
Traditional UIKit Label:
```swift let label = UILabel() label.text = "Hello, SwiftUI!" ```
SwiftUI Text View:
```swift Text("Hello, SwiftUI!") ```
The SwiftUI approach is more concise and focuses on the content, rather than the configuration.
2. What is Combine?
Combine is a framework that Apple introduced to provide tools for processing values over time. It offers a way to work with asynchronous and event-driven code using declarative Swift syntax. With Combine, you can reactively handle changes, such as user input, network responses, and more.
Example: Network Request with Combine
Using Combine, a simple network request can be transformed and processed:
```swift let url = URL(string: "https://api.example.com/data")! let publisher = URLSession.shared.dataTaskPublisher(for: url) .map(\.data) .decode(type: DataResponse.self, decoder: JSONDecoder()) .eraseToAnyPublisher() ```
With this approach, the `publisher` will emit values or errors when the network request completes.
3. The Power of Combining SwiftUI with Combine
SwiftUI’s declarative nature paired with Combine’s reactive handling of asynchronous events creates a powerful combo. This integration simplifies UI updates in response to data changes.
Example: Binding a TextField with a Published Property
Let’s see how we can combine the two frameworks with a real-world example: a `TextField` updating its value and triggering a change.
First, create a view model with a `@Published` property:
```swift class UserViewModel: ObservableObject { @Published var username: String = "" } ```
The `@Published` property wrapper will automatically publish changes to the `username` property.
Now, let’s create a SwiftUI view that binds to this property:
```swift struct UserView: View { @ObservedObject var viewModel: UserViewModel var body: some View { TextField("Enter your username", text: $viewModel.username) } } ```
Here, `$viewModel.username` is a binding to the `username` property in `UserViewModel`. Any change in the `TextField` will automatically update the `username` property and vice versa.
4. Advantages of this Approach:
- Reduced boilerplate code: The tight integration eliminates the need for numerous delegate methods or manual observation.
- Improved data consistency: The reactive bindings ensure that the UI and the data are always in sync.
- Simplified error handling: With Combine’s operators, handling errors becomes a more streamlined process.
- Enhanced code readability: The declarative nature of both SwiftUI and Combine results in cleaner, more intuitive code.
Conclusion
SwiftUI and Combine, when used together, form a robust duo that paves the way for efficient, reactive iOS development. By shifting the focus from how things should be done to what needs to be achieved, they enable developers to write more consistent, readable, and maintainable code. As businesses increasingly seek to hire Swift developers and as the iOS development ecosystem evolves, embracing these modern tools can provide a significant edge, leading to more interactive and responsive applications.
Table of Contents
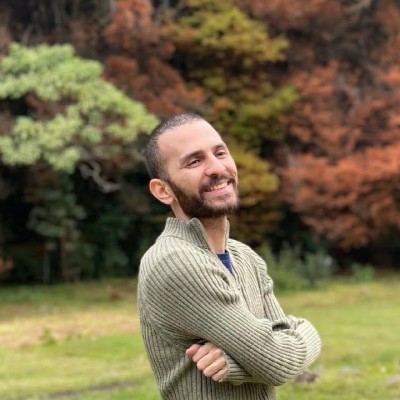
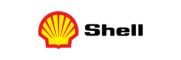