How do I use conditional statements in Swift?
Conditional statements in Swift are essential for controlling the flow of your code based on certain conditions. They allow you to execute different blocks of code depending on whether specific conditions are met. Swift provides several types of conditional statements, including `if`, `else if`, `else`, and `switch`. Here’s a breakdown of how to use conditional statements in Swift:
- `if` Statements:
The most basic form of conditional statement in Swift is the `if` statement. It allows you to execute a block of code if a given condition is true. You can also use multiple `if` statements with `else if` and `else` clauses for more complex conditions.
```swift let age = 25 if age < 18 { print("You are a minor.") } else if age < 65 { print("You are an adult.") } else { print("You are a senior.") } ```
- `switch` Statements:
Swift’s `switch` statement is versatile and allows you to evaluate a value against multiple possible cases. Each case can have associated code that is executed when the case matches. Swift’s `switch` is more powerful than many other languages because it doesn’t “fall through” to the next case by default.
```swift let day = "Monday" switch day { case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday": print("It's a weekday.") case "Saturday", "Sunday": print("It's a weekend.") default: print("Invalid day.") } ```
- Ternary Conditional Operator (`? :`):
Swift also provides a concise ternary conditional operator for inline conditional expressions. It allows you to assign a value based on a condition in a single line of code.
```swift let isEven = (age % 2 == 0) ? true : false ```
- `guard` Statements:
As mentioned earlier, `guard` statements are used for early exits and are particularly useful for unwrapping optionals safely. If a condition isn’t met, a `guard` statement can exit the current scope gracefully.
```swift func processValue(_ value: Int?) { guard let unwrappedValue = value else { print("Value is missing!") return } // Continue with 'unwrappedValue' safely } ```
Conditional statements are fundamental to programming and are used extensively in Swift to make decisions and control program flow. They are essential for building logic and responding to different situations in your code, making your applications more flexible and robust.
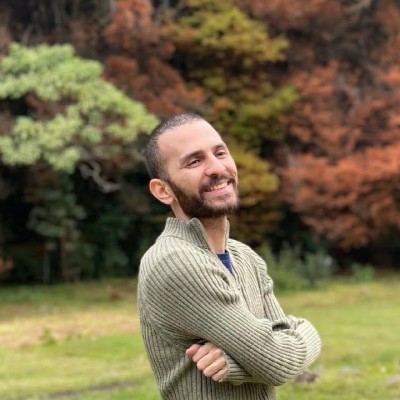
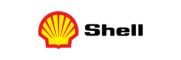