How do I use ‘continue’ in Swift?
In Swift, the ‘continue’ statement is a control flow statement that allows you to skip the rest of the current iteration within a loop and proceed to the next iteration. It’s a valuable tool for controlling the flow of your loops and selectively executing code based on specific conditions.
Here’s how you can use the ‘continue’ statement in Swift:
- Skipping Specific Iterations: When the ‘continue’ statement is encountered within a loop (such as ‘for-in,’ ‘while,’ or ‘repeat-while’), it immediately exits the current iteration and moves on to the next one. This can be helpful when you want to skip certain values or conditions within a loop.
```swift for number in 1...5 { if number == 3 { continue } print(number) } ```
In this example, when ‘number’ is equal to 3, the ‘continue’ statement skips the printing step for that value and moves to the next iteration.
- Looping with Conditions: You can use ‘continue’ in conjunction with conditional statements to control which iterations should be skipped. For example, you might want to skip even numbers or specific elements in an array.
```swift let numbers = [1, 2, 3, 4, 5] for number in numbers { if number % 2 == 0 { continue // Skip even numbers } print(number) } ```
Here, the ‘continue’ statement skips even numbers, resulting in only odd numbers being printed.
- Avoiding Unwanted Execution: ‘continue’ helps you avoid executing unnecessary code when certain conditions are met, improving the efficiency of your loops.
The ‘continue’ statement is a valuable tool for fine-tuning the behavior of your loops. It allows you to skip specific iterations based on conditions, making your code more efficient and enabling you to focus on executing only the necessary code for your desired logic.
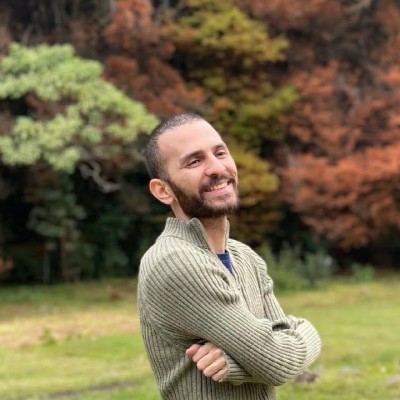
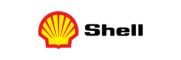