From Basic Layers to 3D: Mastering Visual Effects with Swift and Core Animation
When developing for iOS, the potential to deliver visually striking interfaces and interactions is a major advantage. Core Animation, Apple’s framework for high-performance, hardware-accelerated animations, is the cornerstone of this capability. Paired with the elegance and efficiency of Swift, creating attention-grabbing visual effects becomes a fluid process. If you’re looking to elevate your app’s visuals, hiring Swift developers can provide a significant edge. In this article, we’ll delve into a few techniques to make your iOS applications pop with the magic of Core Animation.
Table of Contents
1. What is Core Animation?
At its core (pun intended), Core Animation is a graphics rendering and animation infrastructure available to all iOS and macOS apps. It allows developers to animate properties of UI components over time, and thanks to its hardware acceleration, it ensures smooth and responsive animations.
2. Layer Animations
Every view in iOS has an associated layer, the `CALayer`, which is the real canvas for drawing and animating content. To create a simple fade-in animation for a `UIView` object:
```swift let myView = UIView(frame: CGRect(x: 50, y: 50, width: 100, height: 100)) myView.backgroundColor = .blue // Create a basic animation changing the opacity of the layer let animation = CABasicAnimation(keyPath: "opacity") animation.fromValue = 0 animation.toValue = 1 animation.duration = 2 myView.layer.add(animation, forKey: "opacity") ```
3. Springy Button Animation
A button press with a “spring” effect can make UI feel lively:
```swift func animateButtonPress(button: UIButton) { // Start with a reduced scale button.transform = CGAffineTransform(scaleX: 0.9, y: 0.9) UIView.animate(withDuration: 0.5, delay: 0, usingSpringWithDamping: 0.5, initialSpringVelocity: 2, options: .curveEaseInOut, animations: { button.transform = .identity }) } ```
4. Rotating Views
This is a fun animation, especially useful for refreshing icons or loading spinners.
```swift func rotateView(view: UIView, duration: CFTimeInterval = 1) { let rotationAnimation = CABasicAnimation(keyPath: "transform.rotation") rotationAnimation.fromValue = 0 rotationAnimation.toValue = 2 * .pi rotationAnimation.duration = duration rotationAnimation.repeatCount = Float.infinity view.layer.add(rotationAnimation, forKey: "rotation") } ```
5. Gradient Animations
Dynamic gradients can provide your app with a sophisticated feel:
```swift func animateGradient(for view: UIView) { let gradientLayer = CAGradientLayer() gradientLayer.frame = view.bounds gradientLayer.colors = [UIColor.red.cgColor, UIColor.blue.cgColor] let animation = CABasicAnimation(keyPath: "colors") animation.fromValue = [UIColor.red.cgColor, UIColor.blue.cgColor] animation.toValue = [UIColor.blue.cgColor, UIColor.red.cgColor] animation.duration = 3 animation.autoreverses = true animation.repeatCount = Float.infinity gradientLayer.add(animation, forKey: "colors") view.layer.addSublayer(gradientLayer) } ```
6. 3D Transformations
Achieving 3D effects gives depth to the flat world of screens:
```swift func apply3DTransform(to view: UIView) { var transform = CATransform3DIdentity transform.m34 = -1.0 / 500.0 transform = CATransform3DRotate(transform, .pi / 4, 0, 1, 0) UIView.animate(withDuration: 2) { view.layer.transform = transform } } ```
7. Keyframe Animations
For more intricate animations, such as multi-step transformations, `CAKeyframeAnimation` is invaluable:
```swift func bounceAnimation(for view: UIView) { let animation = CAKeyframeAnimation(keyPath: "transform.scale") animation.values = [1, 1.5, 0.8, 1.2, 0.9, 1.1, 1] animation.keyTimes = [0, 0.2, 0.4, 0.6, 0.8, 0.9, 1] animation.duration = 2 view.layer.add(animation, forKey: "bounce") } ```
8. Grouping Animations
For simultaneous animations, `CAAnimationGroup` is the tool to use:
```swift func groupAnimations(for view: UIView) { let scaleAnimation = CABasicAnimation(keyPath: "transform.scale") scaleAnimation.fromValue = 1 scaleAnimation.toValue = 1.5 let rotationAnimation = CABasicAnimation(keyPath: "transform.rotation") rotationAnimation.fromValue = 0 rotationAnimation.toValue = 2 * .pi let group = CAAnimationGroup() group.animations = [scaleAnimation, rotationAnimation] group.duration = 2 view.layer.add(group, forKey: "scaleAndRotate") } ```
Conclusion
The interplay between Swift and Core Animation opens up a world of mesmerizing visual effects in iOS apps. With just a few lines of code, you can breathe life into your UI, making your application engaging and memorable. For those looking to further enhance their app’s aesthetics, hiring Swift developers can provide an invaluable advantage.
By understanding the underlying principles and experimenting with different animation properties, you’ll be equipped to craft experiences that delight and retain users. Dive into the world of animations and let your creativity, coupled with expert Swift developers, truly shine!
Table of Contents
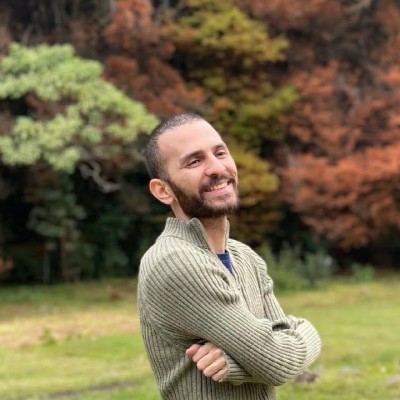
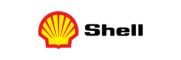