Swift and Core Animation: Creating Engaging Animations in iOS Apps
Animations play a crucial role in creating engaging and dynamic user experiences in iOS apps. Core Animation, a powerful framework provided by Apple, offers the tools needed to bring your app’s interface to life. Combined with Swift’s modern syntax and features, Core Animation enables developers to implement smooth, interactive animations with ease. This blog explores how Swift can be used with Core Animation to enhance user interactions and provides practical examples for creating compelling animations.
Understanding Core Animation
Core Animation is a framework that enables the creation of high-performance, smooth animations in iOS apps. It allows developers to animate views and layer properties effortlessly, making it a vital tool for improving user engagement and interface responsiveness.
Using Swift with Core Animation
Swift’s concise syntax and features, such as closures and type inference, complement Core Animation’s capabilities perfectly. Below are some key aspects and code examples demonstrating how to leverage Core Animation with Swift.
1. Basic Animations with Core Animation
Core Animation simplifies the process of animating view properties. You can animate changes in properties such as position, opacity, and size.
Example: Basic Fade Animation
Here’s how to create a simple fade-in animation for a view.
```swift import UIKit class ViewController: UIViewController { let animatedView = UIView() override func viewDidLoad() { super.viewDidLoad() // Setup the view animatedView.frame = CGRect(x: 100, y: 100, width: 100, height: 100) animatedView.backgroundColor = .red animatedView.alpha = 0 view.addSubview(animatedView) // Perform the animation UIView.animate(withDuration: 1.0) { self.animatedView.alpha = 1 } } } ```
2. Keyframe Animations
Keyframe animations allow you to specify multiple animations within a single animation block, creating more complex effects.
Example: Bouncing Animation
This example demonstrates how to create a bouncing animation using keyframes.
```swift import UIKit class ViewController: UIViewController { let animatedView = UIView() override func viewDidLoad() { super.viewDidLoad() // Setup the view animatedView.frame = CGRect(x: 100, y: 100, width: 100, height: 100) animatedView.backgroundColor = .blue view.addSubview(animatedView) // Perform keyframe animation UIView.animateKeyframes(withDuration: 1.0, delay: 0, options: [.repeat, .autoreverse]) { UIView.addKeyframe(withRelativeStartTime: 0, relativeDuration: 0.25) { self.animatedView.transform = CGAffineTransform(translationX: 0, y: -50) } UIView.addKeyframe(withRelativeStartTime: 0.25, relativeDuration: 0.25) { self.animatedView.transform = CGAffineTransform.identity } } } } ```
3. Layer Animations
Core Animation’s `CALayer` class provides more advanced animation capabilities beyond what’s available with `UIView`. You can animate properties of layers, such as position, bounds, and transformations.
Example: Rotating a Layer
Here’s how to animate the rotation of a layer.
```swift import UIKit class ViewController: UIViewController { let animatedLayer = CALayer() override func viewDidLoad() { super.viewDidLoad() // Setup the layer animatedLayer.frame = CGRect(x: 100, y: 100, width: 100, height: 100) animatedLayer.backgroundColor = UIColor.green.cgColor view.layer.addSublayer(animatedLayer) // Create rotation animation let rotationAnimation = CABasicAnimation(keyPath: "transform.rotation.z") rotationAnimation.fromValue = 0 rotationAnimation.toValue = Double.pi * 2 rotationAnimation.duration = 2.0 rotationAnimation.repeatCount = .infinity animatedLayer.add(rotationAnimation, forKey: "rotationAnimation") } } ```
4. Animating Constraints
You can animate layout changes by animating constraints, making the animations responsive to user interactions and dynamic content.
Example: Expanding View
This example demonstrates how to animate a view’s constraints to create an expanding effect.
```swift import UIKit class ViewController: UIViewController { let expandableView = UIView() var viewHeightConstraint: NSLayoutConstraint! override func viewDidLoad() { super.viewDidLoad() // Setup the view expandableView.backgroundColor = .purple expandableView.translatesAutoresizingMaskIntoConstraints = false view.addSubview(expandableView) NSLayoutConstraint.activate([ expandableView.centerXAnchor.constraint(equalTo: view.centerXAnchor), expandableView.centerYAnchor.constraint(equalTo: view.centerYAnchor), expandableView.widthAnchor.constraint(equalToConstant: 100), viewHeightConstraint = expandableView.heightAnchor.constraint(equalToConstant: 100) ]) // Perform the animation UIView.animate(withDuration: 1.0) { self.viewHeightConstraint.constant = 200 self.view.layoutIfNeeded() } } } ```
Conclusion
Swift, combined with Core Animation, provides a powerful toolkit for creating engaging and dynamic animations in iOS apps. By leveraging these tools, developers can enhance user experiences, improve interaction responsiveness, and make their apps stand out. From basic animations to complex layer transformations, Core Animation offers a wealth of possibilities to explore.
Further Reading:
Table of Contents
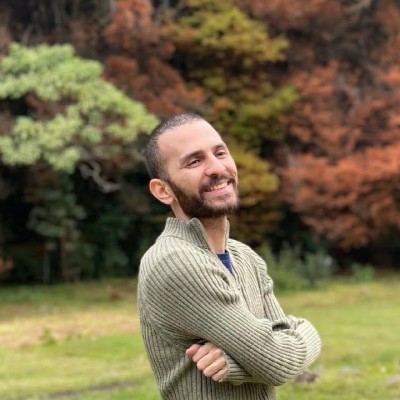
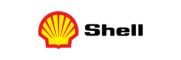