Swift and Core Audio: Manipulating Audio in iOS Apps
Understanding Core Audio
Core Audio is a powerful framework provided by Apple for handling audio processing on iOS and macOS devices. It offers a suite of APIs that allow developers to manipulate audio at a low level, enabling high-quality audio experiences in their applications. Core Audio is ideal for tasks such as real-time audio processing, audio recording, and playback.
Using Swift for Audio Manipulation
Swift, combined with Core Audio, allows developers to build applications with advanced audio features. The framework’s rich API provides access to various audio functionalities, from simple playback to complex audio effects. Below are key aspects and code examples demonstrating how Swift can be employed for audio manipulation using Core Audio.
1. Setting Up an Audio Session
Before performing any audio operations, you need to configure an audio session. The `AVAudioSession` class helps manage the audio behavior of your app.
Example: Configuring an Audio Session
```swift import AVFoundation class AudioManager { private let audioSession = AVAudioSession.sharedInstance() func configureSession() { do { try audioSession.setCategory(.playAndRecord, mode: .default) try audioSession.setActive(true) } catch { print("Failed to configure audio session: \(error)") } } } ```
2. Playing and Recording Audio
Core Audio provides APIs for both audio playback and recording. `AVAudioPlayer` and `AVAudioRecorder` are commonly used classes for these purposes.
Example: Playing Audio
```swift import AVFoundation class AudioPlayer { var audioPlayer: AVAudioPlayer? func playAudio(fileName: String) { guard let url = Bundle.main.url(forResource: fileName, withExtension: "mp3") else { print("Audio file not found.") return } do { audioPlayer = try AVAudioPlayer(contentsOf: url) audioPlayer?.play() } catch { print("Failed to play audio: \(error)") } } } ```
Example: Recording Audio
```swift import AVFoundation class AudioRecorder { var audioRecorder: AVAudioRecorder? func startRecording() { let fileName = "recording.m4a" let fileURL = FileManager.default.temporaryDirectory.appendingPathComponent(fileName) let settings: [String: Any] = [ AVFormatIDKey: Int(kAudioFormatMPEG4AAC), AVSampleRateKey: 12000, AVNumberOfChannelsKey: 1, AVEncoderAudioQualityKey: AVAudioQuality.high.rawValue ] do { audioRecorder = try AVAudioRecorder(url: fileURL, settings: settings) audioRecorder?.record() } catch { print("Failed to start recording: \(error)") } } func stopRecording() { audioRecorder?.stop() } } ```
3. Applying Audio Effects
Core Audio allows for real-time audio processing. You can use `AVAudioEngine` and `AVAudioUnit` to apply various audio effects and filters.
Example: Applying a Reverb Effect
```swift import AVFoundation class AudioEffects { private let audioEngine = AVAudioEngine() private let reverb = AVAudioUnitReverb() func setupReverb() { reverb.loadFactoryPreset(.mediumRoom) reverb.wetDryMix = 50 audioEngine.attach(reverb) audioEngine.connect(audioEngine.inputNode, to: reverb, format: nil) audioEngine.connect(reverb, to: audioEngine.outputNode, format: nil) do { try audioEngine.start() } catch { print("Failed to start audio engine: \(error)") } } } ```
4. Integrating with Core Audio APIs
For advanced use cases, you may need to interact with lower-level Core Audio APIs. Swift provides interoperability with these APIs through bridging and wrapping techniques.
Example: Using Audio Queue Services
```swift import AudioToolbox class AudioQueueManager { private var audioQueue: AudioQueueRef? func setupAudioQueue() { var format = AudioStreamBasicDescription() format.mSampleRate = 44100 format.mFormatID = kAudioFormatLinearPCM format.mFormatFlags = kAudioFormatFlagIsSignedInteger | kAudioFormatFlagIsPacked format.mBitsPerChannel = 16 format.mChannelsPerFrame = 2 format.mBytesPerPacket = 4 format.mFramesPerPacket = 1 format.mBytesPerFrame = 4 format.mReserved = 0 let status = AudioQueueNewOutput(&format, nil, nil, nil, nil, 0, &audioQueue) if status != noErr { print("Failed to create audio queue: \(status)") } } } ```
Conclusion
Swift, in combination with Core Audio, offers a comprehensive set of tools for audio manipulation in iOS applications. Whether you’re playing and recording audio, applying effects, or integrating with low-level APIs, Swift provides a powerful framework to enhance your app’s audio capabilities. By leveraging these tools effectively, you can create rich and immersive audio experiences for your users.
Further Reading:
Table of Contents
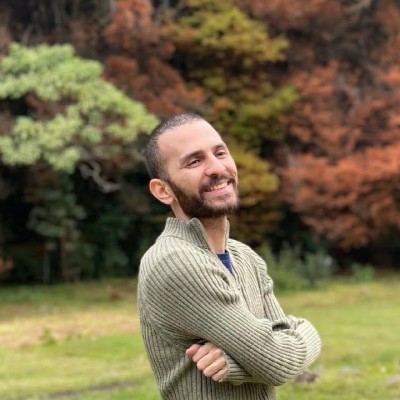
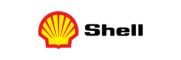