How to Seamlessly Integrate Bluetooth in Your iOS Apps with Swift
Bluetooth is an essential technology for countless applications today, from connecting wireless headphones to transferring data between devices. In this blog post, we’ll explore how to integrate Bluetooth capabilities into your iOS app using Swift and the Core Bluetooth framework. If you’re looking to enhance your app further, consider the option to hire Swift developers for expert guidance and implementation.
Table of Contents
1. Introduction to Core Bluetooth
Core Bluetooth is Apple’s framework for working with Bluetooth-equipped devices. With it, you can discover, connect, and exchange data with other Bluetooth devices directly from your app. Specifically, Core Bluetooth focuses on Bluetooth Low Energy (BLE) devices, a power-efficient version of the classic Bluetooth.
2. Core Concepts
Before diving into code, let’s understand a few key concepts:
– Central: The device (e.g., iPhone) searching for and connecting to peripheral devices.
– Peripheral: The device (like a heart rate monitor or a smart bulb) being connected to by the central.
– Characteristic: A data point on a peripheral, such as the current heart rate value on a heart rate monitor.
– Service: A collection of related characteristics.
3. Setting Up Your Project
To start, you need to ensure you have the proper permissions:
- Open your project’s `Info.plist`.
- Add the `NSBluetoothAlwaysUsageDescription` key with a message describing why your app needs Bluetooth access.
4. Discovering Devices
To discover BLE devices:
```swift import CoreBluetooth class BluetoothManager: NSObject, CBCentralManagerDelegate { private var centralManager: CBCentralManager! override init() { super.init() centralManager = CBCentralManager(delegate: self, queue: nil) } func centralManagerDidUpdateState(_ central: CBCentralManager) { if central.state == .poweredOn { // Start scanning for devices centralManager.scanForPeripherals(withServices: nil, options: nil) } } func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String : Any], rssi RSSI: NSNumber) { print("Discovered \(peripheral.name ?? "a device")") } } ```
5. Connecting to a Device
Once a desired device is discovered, you can connect to it:
```swift func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String : Any], rssi RSSI: NSNumber) { print("Discovered \(peripheral.name ?? "a device")") centralManager.stopScan() centralManager.connect(peripheral, options: nil) } ```
6. Discovering Services and Characteristics
After connecting, you can explore the peripheral’s services and their respective characteristics:
```swift func centralManager(_ central: CBCentralManager, didConnect peripheral: CBPeripheral) { print("Connected to \(peripheral.name ?? "a device")") peripheral.delegate = self peripheral.discoverServices(nil) } func peripheral(_ peripheral: CBPeripheral, didDiscoverServices error: Error?) { for service in peripheral.services! { peripheral.discoverCharacteristics(nil, for: service) } } func peripheral(_ peripheral: CBPeripheral, didDiscoverCharacteristicsFor service: CBService, error: Error?) { for characteristic in service.characteristics! { print(characteristic) } } ```
7. Reading from and Writing to Characteristics
To read a characteristic:
```swift if characteristic.properties.contains(.read) { peripheral.readValue(for: characteristic) } func peripheral(_ peripheral: CBPeripheral, didUpdateValueFor characteristic: CBCharacteristic, error: Error?) { let data = characteristic.value // Handle the data as needed } ```
To write to a characteristic:
```swift if characteristic.properties.contains(.write) { let data: Data = // your data here peripheral.writeValue(data, for: characteristic, type: .withResponse) } ```
Conclusion
Building Bluetooth-enabled iOS apps might seem daunting at first, but with Swift and the Core Bluetooth framework, it’s more straightforward than you might think. By understanding the basic concepts and working step-by-step through discovering, connecting, and communicating with BLE devices, you can open up a world of possibilities for your iOS applications. Whether you’re creating a fitness app, home automation, or any other Bluetooth-centric application, the power of Core Bluetooth is there to help you succeed. If you’re looking to achieve optimal results and robust functionalities, you might also consider the option to hire Swift developers for tailored solutions and expertise.
With this basic walkthrough, you’re well on your way to integrating Bluetooth functionalities into your iOS apps. As with all development, practice and real-world application are key. Dive in, start building, and watch the magic of Bluetooth unfold in your hands.
Table of Contents
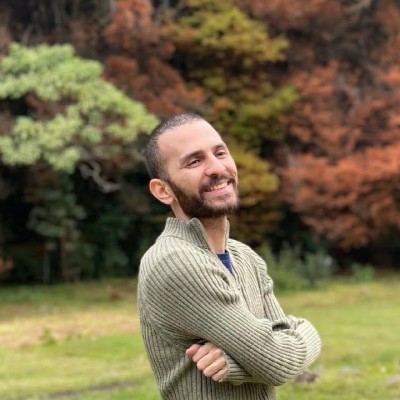
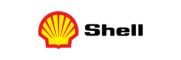