Redefining iOS Data Management: Discover Advanced Core Data Secrets
Core Data is an essential framework for data persistence in iOS apps. While the basics of Core Data might be straightforward, mastering advanced techniques can supercharge your app’s performance and responsiveness. In this post, we’ll delve deep into some advanced strategies and techniques for effective data management in iOS using Swift and Core Data.
Table of Contents
1. Batch Processing
When working with a large dataset, one might encounter performance bottlenecks, especially when inserting, updating, or deleting multiple records. Instead of doing these operations one-by-one, batch processing can be used.
Example: Let’s assume we have an `Employee` entity, and we need to update the status of multiple records:
```swift let fetchRequest: NSFetchRequest<NSFetchRequestResult> = Employee.fetchRequest() fetchRequest.predicate = NSPredicate(format: "status == %@", "inactive") let batchUpdate = NSBatchUpdateRequest(entityName: "Employee") batchUpdate.propertiesToUpdate = ["status": "active"] batchUpdate.resultType = .updatedObjectsCountResultType do { let result = try context.execute(batchUpdate) as? NSBatchUpdateResult print("Updated \(result?.result ?? 0) records") } catch { print("Failed to execute request:", error) } ```
2. Fetch Request Templates
Instead of writing fetch requests programmatically every time, you can define them in the Data Model. These are called Fetch Request Templates.
Example: If we often fetch all employees in a department, we can create a fetch request template.
- Open the `.xcdatamodeld` file.
- Click `Add Fetch Request`.
- Name it “employeesInDepartment”.
- Set its predicate to `department == $DEPARTMENT_NAME`.
Now, in Swift:
```swift let fetchRequest = managedObjectModel.fetchRequestTemplate(forName: "employeesInDepartment") fetchRequest?.predicate = NSPredicate(format: "department == %@", "HR") do { let employees = try context.fetch(fetchRequest!) as? [Employee] } catch { print("Error fetching:", error) } ```
3. Asynchronous Fetching
Fetching data can be time-consuming, especially with larger datasets. To keep the UI responsive, fetch data asynchronously.
Example:
```swift let fetchRequest: NSFetchRequest<Employee> = Employee.fetchRequest() fetchRequest.predicate = NSPredicate(format: "status == %@", "active") let asyncFetchRequest = NSAsynchronousFetchRequest(fetchRequest: fetchRequest) { result in guard let employees = result.finalResult else { return } // Process the fetched employees } do { try context.execute(asyncFetchRequest) } catch { print("Error fetching:", error) } ```
4. Lightweight Migration
When evolving your app, the data model will likely change. Core Data supports automatic “lightweight” migrations for simple changes.
Steps:
- Click on your `.xcdatamodeld` file.
- Add a new version (Editor > Add Model Version).
- Make the desired changes.
- Set the new version as the current version.
- Ensure that the “Lightweight Migration” options are set in the `NSPersistentStore` initialization.
5. Derived Attributes
Derived attributes are read-only and are computed based on one or more other attributes.
Example: Suppose our `Employee` entity has attributes `firstName` and `lastName`. We can add a derived attribute `fullName`.
- Add the `fullName` attribute to the entity.
- Set its derived expression to `firstName + ” ” + lastName`.
- Use it in fetch requests like any other attribute.
6. Advanced Relationships: Uniquing
Uniquing ensures that for a given managed object context, there’s only one managed object representing a specific record in the persistent store.
Example: Suppose two `Employee` objects point to the same `Department`. Instead of having two different `Department` objects in memory, uniquing ensures that both `Employee` objects reference the same `Department` instance.
Uniquing is automatically managed by Core Data. It’s important to know about it as it helps optimize memory usage.
7. Faulting
Faulting is a mechanism by which Core Data minimizes memory usage. Instead of fetching the entire object, Core Data fetches only a placeholder. The actual data is fetched when that particular attribute is accessed.
You might sometimes want to turn off faulting for performance reasons:
```swift fetchRequest.returnsObjectsAsFaults = false ```
8. Advanced NSPredicates
NSPredicate is a powerful tool in Core Data. Beyond basic comparisons, it can perform advanced operations.
Example: Fetch employees who have worked for more than a specific number of days:
```swift let daysWorked = 100 let predicate = NSPredicate(format: "daysWorked > %@", daysWorked) fetchRequest.predicate = predicate ```
Conclusion
Core Data is a robust and comprehensive solution for data management in iOS apps. By mastering the advanced techniques listed above, you can ensure that your app remains efficient, responsive, and scalable. Always remember to profile your app using Instruments to keep a check on performance, especially when working with large datasets.
Table of Contents
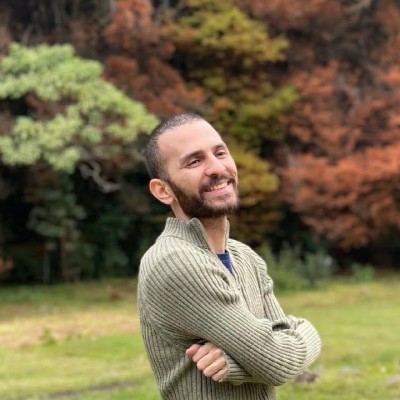
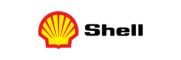